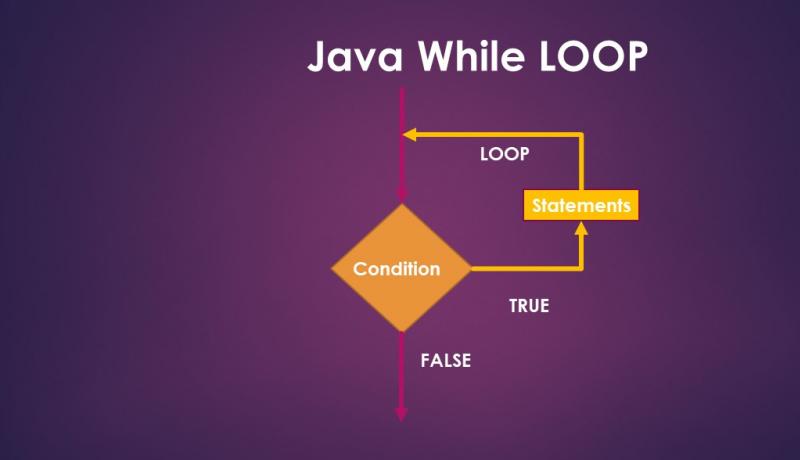
As part of Loop control statements, Java provides three loops namely WHILE loop, FOR loop and DO WHILE loop. Loops repeatedly execute a set of statements as long as the Loop condition is satisfied. A while loop executes the statements under it only if the condition is satisfied.
Java While Loop with Break and Continue Statements
Java continued the same syntax of while loop from the C Language. A while loop accepts a condition as an input. The condition should evaluate to either true or false. A while loop in Java does not work with integers. In Java, a number is not converted to a boolean constant.
Syntax:
while( condition ) { //statements //loop counters }
Example 1: A simple while loop
Note that this while loop will become an Infinite Loop if we do not increment the loop counter variable.
int num=1; while(num <= 5) { System.out.print(num + ", "); num++; //Loop counter //Comment this to make it infinite loop } //1, 2, 3, 4, 5,
While Loop with a break statement
Java provides break statement to make the program control abruptly leave the block or loop inside which a break statement is encountered. Here, we will use the break statement without a label.
int num=1; while(true) { System.out.print(num + ", "); num++; //Loop counter if(num > 5) break; //break the loop } //1, 2, 3, 4, 5,
While loop with a Continue statement
Java provides a continue statement to make the program control abruptly go to the beginning of the loop. So, the loop is not exited in this case. Only that current iteration is skipped. In the below program we try to print only EVEN numbers in a given range. Break or Continue statements are generally used along with an IF condition.
int num=1; while( num <=10 ) { num++; //Loop counter if(num%2 !=0 ) continue; System.out.print(num + ", "); } //2, 4, 6, 8, 10
Nested While Loop
You can nest one while loop inside another while loop. It is fun to use break and continue with nested while loop. In the below program, you will how to break inner and outer loops with break and continue statements. You can also nest different loops like for loop and do-while inside a while loop.
class NestedWhile { public static void main(String args[]) { int i=1, j=1; while(i <= 5) { j=1; while(true) { System.out.print(j + " "); if(i==j) break; j++; } System.out.println(""); i++; } } } //OUTPUT //1 //1 2 //1 2 3 //1 2 3 4 //1 2 3 4 5
This is how a Java While Loop works. In the next chapter, we shall discuss Java DO WHILE loop.
Share this tutorial with friends and colleagues to encourage authors.