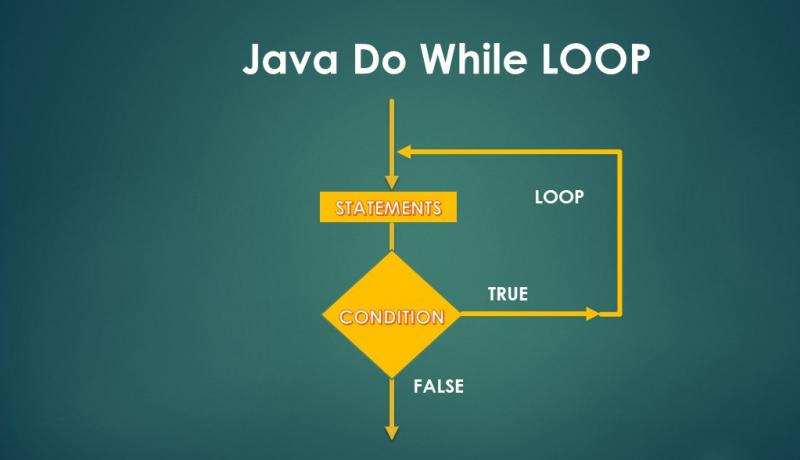
Java language provides a Do While loop with slightly modified features of the WHILE loop.
Normal WHILE loop does not execute loop statements if the condition is not satisfied or true. But a DO WHILE loop executes the loop statements for the first time without even checking the loop condition. Programmers usually prefer a WHILE loop to DO WHILE loop.
A Do While loop condition should evaluate to either true or false values.
Java Do While Loop with Break and Continue
Syntax of a Do While loop is as follows.
do{ //statements //loop counter }while( condition ); //Semicolon is present
Example:
int a=1; do { System.out.print(a +","); a++; }while(a<=5); //OUTPUT //1,2,3,4,5
Java Do While with Break Statement
"Break" statement inside a Do While loop causes the program control to exit the loop. The statements below the Do While loop if any will be executed. We use an IF statement to break out of the loop based on a condition.
Example:
int a=1; do { if(a>5) break; System.out.print(a +","); a++; }while(true);
//OUTPUT //1,2,3,4,5,
Java Do While with Continue Statement
"Continue" statement inside a Do While loop causes the program control to skip the statements below it and go to the beginning of the loop. Loop condition is checked again before executing the statements from the beginning. We have used an IF statement to use CONTINUE statement.
Example:
int a=0; do{ a++; if(a%2==0) continue; System.out.print(a +","); }while(a <= 9); // //OUTPUT //1,3,5,7,9,
Nesting of Do While Loop
A Do While loop can be nested inside another Do While loop, For Loop and While loop without any problem.
Example:
int a=5, b=5; do { b=a; do { System.out.print(b+","); b--; }while(b>0); a--; System.out.println(""); }while(a > 0); // //OUTPUT //5,4,3,2,1, //4,3,2,1, //3,2,1, //2,1, //1,
This is how a Java DO WHILE loop works. In the coming chapters, we shall see Java FOR loop.
Share this article with your friends and colleagues to encourage authors.