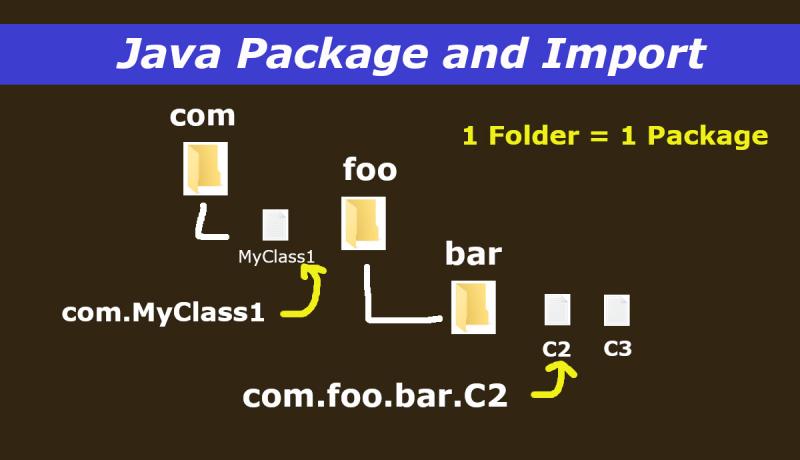
Java Packages are containers for CLASS files with a folder structure. Packages allow us in Visibility and Namespace management of Java Classes, Variables and Methods. Let us learn more about a Java Package, its rules and usage with examples in this Last Minute Java Tutorial.
All Java classes, Abstract classes and Interfaces can use these package and import statements. Development teams can maintain individual packages so that they do not need to worry about Same name collisions for classes and interfaces.
The concept of Java packages is similar to a House containing members whose names are unique. The members of an outside House may reuse names without collision.
Java Packages Rules Explained
In simple words, a Java package is Directory on a computer file system. The computer may run any OS (Operating System like Windows, Unix or Linux). The file system is common.
There are two ways we use Java packages.
- Declaring a package
- Importing a package.
1. Declaring a Java Package
The keyword used to declare a package is "package". A package declaration statement should be the very first statement in a Java class file. The line number may be anyone like 1, 2, 3 or any. All the classes in the java file belong to the mentioned PACKAGE-NAME.
Syntax:
package PACKAGE_NAME; //very first statement in a class file
2. Importing a Java Package
The keyword used to import a Java package or Java class is "import". Java import statements can only come after the PACKAGE declaration statement if any.
Syntax:
package PACKAGE_NAME2; //It may be absent also import PACKAGE_NAME1.*; //STAR imports all classes import PACKAGE_NAME2.MyClass; //import a specific class import PKG2.PKG3.PKG.*; //before class declaration class CLASSNAME { } //
Note: By default, java.lang package is imported into each class file by the compiler and runtime. The popular classes packed in this Java Language package are String, StringBuffer, StringBuilder, Integer, Short, Long, Float, Double, Byte, Boolean, Math, Number, System, Thread etc. All other library packages should be explicitly imported if required.
Rules of a Java Package:
- Package name and directory name should be the same.
- A Java package may contain Sub-Packages or sub-directories with unique names.
- A Java package may contain any number of class files.
- The package declaration statement should be the very first statement in a Java file.
- The package import statement can come after the package declaration statement and before any Class declarations.
- The same package-name or class-name can be reused in some other packages but not within the same package.
- The directories corresponding to the Java packages are hierarchical in nature.
- To refer to an inner-package, use DOT or PERIOD symbol in conjunction with all the names of parent-packages. For example, use pkga.pkgb.pkgc.pkgd.
- Java does not define the maximum depth of inner packages. But the OS may limit this number.
- You can declare only one package name in a CLASS file. In other words, there can only be one package (declaration) statement.
- You can import any number of Packages or specific Package-classes into one java CLASS. In other words, there can be any number of import statements.
- You can import only one specific class of a given package by directly mentioning the package name and the class name.
- You can import all classes of a given package with a START (*) symbol next to the package name separated by a DOT or PERIOD symbol.
Example Program 1:
Let us define a class Vehicle under the package com kept in the folder "com".
Vehicle.java
package com; //package name is COM public class Vehicle { int caculateMileage() { return 10; //dummy } }
Let us define a class that imports the above class from the package "com" using an "import" statement.
PackageExample.java
package com4; import com.*; //we want to use Vehicle class public class PackageExample { public static void main(String[] args) { //Using the imported class from package com Vehicle vehicle = new Vehicle(); int mileage = vehicle.caculateMileage(); System.out.println("Mileage = " + mileage); } } //OUTPUT Mileage = 10
Example Program 2:
Let us import "Calendar" class from the package "util" in a real-world example.
package mypack; import java.util.Calendar; //importing a class public class PackageExample2 { public static void main(String[] args) { Calendar cal = Calendar.getInstance(); int year = cal.get(Calendar.YEAR); System.out.println("Current Year = " + year); } } //OUTPUT Current Year = 2020
The above program needs to know the current Year. So, it has imported a Readymade library class Calendar from the package "util". Otherwise, you need to write more lines of code to get the same.
This is how Java packages ease your job of code reusability.
Let us discuss Java access modifiers, their usage and visibility with packages in the next chapter.
Share this Last Minute Java Packages tutorial with your friends and colleagues to encourage authors.