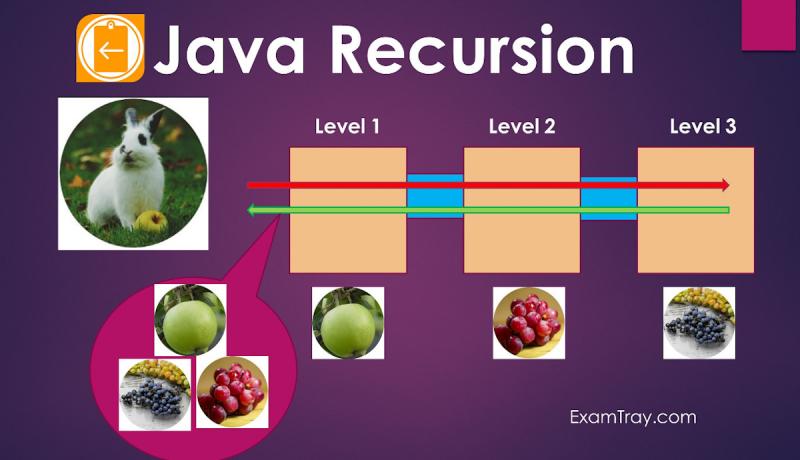
Java programming language supports Recursion. Recursion is a process of calling the same method from within the method. It is achieved only with methods. Let us know more about Java Recursion using this Last Minute Java tutorial.
Java Recursion process uses the Stack memory to store all local variables. The variables or objects are added to and removed from the stack after each method call. Storage for the local variables is allocated fresh on each method invocation. So, there will be a total of N memory allocations if N is the depth of recursion.
Java Recursion of Methods Explained with Examples
A Java class contains so many methods. You can call a method from another method with some arguments and get a return-value from the method after completion of execution. This is very clear. In Recursion, you should call the same method from the same method.
Recursion Syntax:
type METHOD_NAME(type param1, type param2..) { METHOD_NAME(arguments); //Calling the same method }
Let us check the below example using recursion that calculates the sum of N numbers.
public class RecursionTest { int calcSum(int a) { int tmp = 0; System.out.print(a); if(a > 0) { System.out.print("+"); tmp = a + calcSum(a-1); } return tmp; } public static void main(String[] args) { RecursionTest rt = new RecursionTest(); System.out.print("\nSum of first 5 digits: " + rt.calcSum(5)); } } //OUTPUT //5+4+3+2+1+0 //Sum of first 5 digits: 15
Remember that we have stopped this never-ending recursion loop using an IF statement. Even, ELSE statement can also be used. Here the same method you called, again and again, is "int calcSum(int)".
The output of the Recursion statement is given below for each invocation.
5 + calcSum(4) 5 + calcSum(4) + calcSum(3) 5 + calcSum(4) + calcSum(3) + calcSum(2) 5 + calcSum(4) + calcSum(3) + calcSum(2) + calcSum(1) 5 + calcSum(4) + calcSum(3) + calcSum(2) + calcSum(1) + calcSum(0) 5 + calcSum(4) + calcSum(3) + calcSum(2) + calcSum(1)+ 0 5 + calcSum(4) + calcSum(3) + calcSum(2) + 1 + 0 5 + calcSum(4) + calcSum(3) + 2 + 1 + 0 5 + calcSum(4) + 3 + 2 + 1 + 0 5 + 4 + 3 + 2 + 1 + 0 = 15
Here, Recursion occurred for 5 times as the very first call to the method "calcSum" is a normal call.
Note: In Java Recursion, completion of methods occurs from the latest to the oldest method.
Share this Last Minute Java Recursion Tutorial with your friends and colleagues to encourage authors.