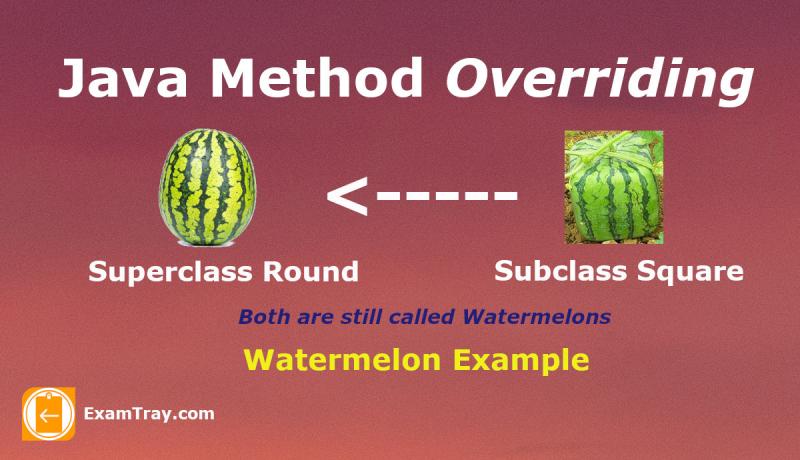
Java Inheritance allows inheriting the methods and properties of a Superclass into a Subclass. Java Method Overriding is nothing but writing a method in a Subclass with the same method signature of the Superclass so that the Java Runtime invokes the method in the subclass. Let us know more about Java Method Overriding and its Rules in this Last Minute Java Tutorial with examples.
Java Method Overriding Rules and Examples
The differences between Java Method Overriding and Method Overloading are given below.
- Java Method Overloading works in the Same Class whereas Method Overriding requires at least two classes (Superclass and Subclass).
- Method Overloading allows reusing the same method name for a different combination of parameters whereas Method Overriding forces the Java Runtime to choose the method in the Subclass to the method of Superclass.
Java Method Signature is the combination of three things namely Return-Type, Method-Name and Parameter-List.
Rules to successfully Override a Java Method are given below.
- Overriding-Method Name in the Superclass and Subclass should be the same.
- The Parameter list of the Overriding-Method in the Superclass and Subclass should be the same.
- The Return-type of Overriding method in the Subclass can be the same as the return-type of superclass's method. For example, use int and int return-types in both the methods.
- The Return-type of Overriding method in the Subclass can be a Subclass-type of return-type of superclass's method. For example, List and ArrayList are return types of Superclass method and Subclass method respectively.
- Access modifier used by the Overridden method of the Subclass should be Less restrictive or same. For example, use protected and public modifiers for methods of Superclass and Subclass respectively.
- Exceptions thrown by the Overridden method of the Subclass should be either same or subtype. Even the Subclass method can omit to throw any exception at all in the process of Overriding a Superclass method.
So, you can maintain the Same method signature for both the methods in the Superclass and Subclass with minor changes to the return type for a successful Method Override.
Example 1: Method Overriding
MethodOverridingExample.java
class Vehicle { void showInfo() { System.out.println("Generic Vehicle"); } void dummy() { System.out.println("Vehicle Dummy"); } } class Car extends Vehicle { void showInfo() //Overridden method { System.out.println("CAR BENZ"); } } public class MethodOverridingExample { public static void main(String[] args) { Vehicle veh = new Vehicle(); //Superclass reference - superclass object //Superclass reference pointing subclass object Vehicle veh2 = new Car(); Car car = new Car(); //subclass reference subclass object veh.showInfo(); veh2.showInfo(); car.showInfo(); veh.dummy(); veh2.dummy(); car.dummy(); } } //OUTPUT Generic Vehicle CAR BENZ CAR BENZ Vehicle Dummy Vehicle Dummy Vehicle Dummy
In the above example, we have a Vehicle-Superclass and a Car-Subclass. Car Subclass maintained the same method signature of the Vehicle Superclass. Thus, showInfo() method has been overridden. If a method of Superclass is not overridden, it can be called simply by the Car-Subclass object as if it is part of the Car-Subclass. Even if the method dummy() is overridden, it can be called by a Car object. The same can be observed seeing the output of the method dummy() by a Vehicle object and a Car object.
Note: If a method or variable is present only in the Subclass, then it can not be called or accessed by a Superclass reference. Only Overridden methods and variables of a subclass can be accessed through a Superclass reference.
Example 2: Method Overriding
MethodOverridingExample2.java
class WaterMelonRound { //Superclass defines general or generic behaviour void show() { System.out.println("Round Watermelon - Superclass"); } } class WaterMelonSquare { //Subclass defines its own version of method void show() { System.out.println("Square Watermelon - Subclass"); } } public class MethodOverridingExample2 { public static void main(String[] args) { WaterMelonRound round = new WaterMelonRound(); round.show(); WaterMelonSquare square = new WaterMelonSquare(); square.show(); } } //OUTPUT Round Watermelon - Superclass Square Watermelon - Subclass
In the above example, we have a Superclass WaterMelonRound and a Subclass WaterMelonSquare. In the process of inheriting the properties of the superclass, a subclass can redefine or define new behaviour for the inherited methods through Overriding. The Overridden method show() in the subclass is an example of that.
Share this Last Minute Java Tutorial on Java Method Overriding among your friends and colleagues to encourage authors.