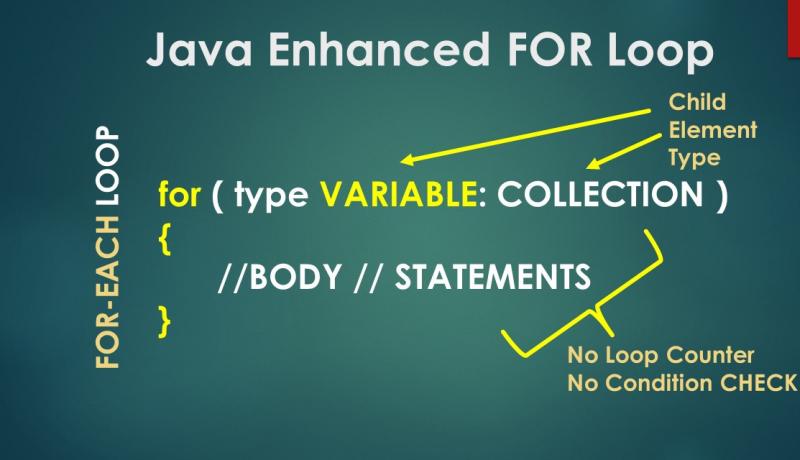
Another variation of the traditional FOR loop is an Enhanced FOR loop. It is also called a FOR-EACH loop. It is completely different from the traditional WHILE, DO WHILE and FOR loops which were adopted from the C Programming Language.
Enhanced FOR loop was introduced with JDK 5 or J2SE 5 version. If you are using an older version of Java, you may not use it.
Enhanced FOR Loop
An Enhanced FOR loop is used to loop through a collection of objects like Array, ArrayList and more. Enhanced FOR loop allows accessing each and every element of the collection sequentially from the start to the end.
Syntax:
for(type VARIABLE: COLLECTION) { //Statements with VARIABLE }
We have to declare a VARIABLE of the same data type as the type of elements of the COLLECTION. Data Type can Primitive like int, char, byte etc or a Non Primitive Object type. There is NO CONDITION part in this enhanced for loop or for-each loop. By default, it works from the first element to the last element.
Example:
int ary[] = {10,11,12,13,14}; for(int a: ary) { System.out.print(a +","); } //OUTPUT //10,11,12,13,14,
Enhanced FOR loop with Break Statement
You can add a BREAK statement inside a FOR-EACH loop or ENHANCED FOR loop. The break statement causes the program control to exit the loop suddenly. The statements after the loop will be executed afterwards.
Example: Prints first 3 elements of Array
int ary[] = {11,12,13,14, 15}; int c=1; for(int a: ary) { System.out.print(a +","); if(c >= 3) break; c++; //OUTPUT //11,12,13,
Enhanced FOR loop with Continue Statement
You can add a CONTINUE statement inside an Enhanced FOR loop. The CONTINUE statement causes the program control to go to the beginning of the loop. If the next elements are available in that collection, the loop continues. Otherwise, the loop completes and the control exits.
Example: Prints odd elements of int Array
int ary[] = {11,12,13,14, 15}; for(int a: ary) { if(a%2==0) continue; System.out.print(a +","); } //OUTPUT //11, 13, 15,
Nesting of Enhanced FOR loop
You can nest an Enhance FOR loop inside another Enhanced FOR loop, Normal FOR loop, WHILE loop and DO WHILE loops.
Example: Enhanced FOR using String and Char Array
String ary[] = {"BE", "BTECH", "DEPLOMO", "MASTERS"}; for(String name: ary) { for(char ch: name.toCharArray()) { System.out.print(ch); } System.out.println(""); } //OUTPUT //BE //BTECH //DEPLOMO //MASTERS
This is how an Enhanced FOR loop or FOR-EACH loop works in Java.
Share this article with your friends and colleagues to encourage authors.