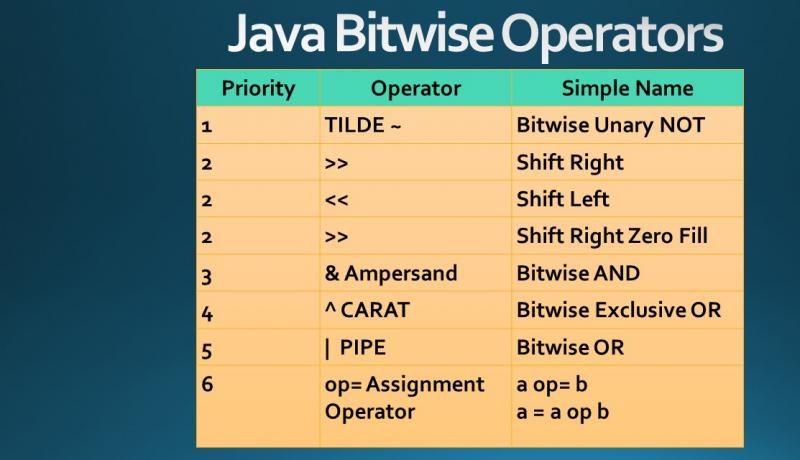
Java language allows developers to work with individual bits of a number using Logical Bitwise Operators. Bitwise logical operators and Logical Bitwise Operators are one and the same. Simply use the term "Bitwise Operators" to refer to bitwise operators. Do not use the word Logical.
Bitwise Operators vs Logical Operators in Java
Bitwise Operators | Logical Operators |
---|---|
Bitwise operators work with integer type data |
Logical operators work with boolean type data. So use LOGICAL to refer to boolean logical operators. |
If boolean operands are present, use Bitwise Operators. | If Relational operators are present, use Logical Operators. |
Bitwise operations are used less in general. | Programmers use logical operators very often. |
Bitwise operators are ~, &, |, ^, <<, >>, >>> and op=. | Logical operators are !, &, &&, |, ||, ^ and op=. |
The common operators used in both Bitwise Operation and Logical Operation are as follows.
- AND - &
- OR - |
- Exclusive OR or XOR - ^
- Compound AND - &=
- Compound OR - |=
- Compound XOR ^=
Java Logical Bitwise Operators and Priority
Each integer type number contains so many bits of information. The purpose of bitwise operators is to manipulate individual bits of an integer type number.
Supported Five Integer Types for Bitwise Operation are as follows.
- byte (8 bits)
- char (16 bits) - unsigned
- short (16 bits)
- int (32 bits)
- long (64 bits)
We have shown 6 different groups of bitwise operators with priority. This priority or precedence is used to resolve a deadlock in solving equal priority operations in an arithmetic expression.
Priority | Operator | Simple Name |
---|---|---|
1 | TILDE ~ | Bitwise Unary NOT |
2 | >> | Shift Right |
2 | << | Shift Left |
2 | >>> | Shift Right Zero Fill |
3 | & Ampersand | Bitwise AND |
4 | ^ CARAT | Bitwise Exclusive OR |
5 | | PIPE | Bitwise OR |
6 | op= |
Assignment Operator a op= b a = a op b |
6 | &= |
Bitwise AND Assignment a &=b a= a&b |
6 | |= |
Bitwise OR Assignment a |=b a= a|b |
6 | ^= |
Bitwise Exclusive OR Assignment a ^=b a= a^b |
6 | >>= |
Bitwise Shift Right Assignment a >>=b a= a>>b |
6 | >>>= |
Bitwise Shift Right Fill Zero Assignment a >>>=b a= a>>>b |
6 | <<= |
Bitwise Shift Left Assignment a <<=b a= a<<b |
Bitwise operators have less priority than arithmetic and relational operators. Only Bitwise Unary NOT or Bitwise Complementary Operator (~) has the highest priority than arithmetic and relational operators.
Example: Bitwise Operator Priority
class BitwisePriority { public static void main(String args[]) { int a=5; 0101 int b=6; 0110 //12 1100 - // 5 0101 - int c = a&b+6; System.out.println(c); } } //OUTPUT //a&(b+6) //a&12 //5&12 //4
Note: We have used Binary Literals in this example. A binary literal starts with 0b. You can specify a number in binary format using this notation. Also, "op" refers to "Operand" in this tutorial.
1. Bitwise Unary NOT Operator (~ TILDE)
Bitwise Unary NOT operator (~) is also called Bitwise Complement Operator. It simply turns bit 0 to bit 1 and bit 1 to bit 0. We have used Type Casting to byte data type in most of the examples here.
Usage example:
class UnaryNOT { public static void main(String args[]) { byte a = 0b00001010; //a=10 byte b = (byte)~a; System.out.println(a + ", " + b); } } //OUTPUT: 10, -11
Operand | Result |
---|---|
0 | 1 |
1 | 0 |
2. Bitwise AND Operator (&)
Bitwise AND operator gives an output of 1 only if both the input bits are 1s. Even if one input bit is 0, the output is 0. Here operand is synonymous to input bit.
Usage example:
class BitwiseAND { public static void main(String args[]) { byte a = 0b00001010; //a=10 byte b = 0b00111001; //b=57 //00001000 = 8 byte c = a & b; System.out.println(a + ", " + b + "= " + c); } } //OUTPUT: 10, 57 = 8
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
3. Bitwise OR Operator (|)
Bitwise OR Operator (|) gives an output of 1 at least if one input bit is 1. Only if both the input bits are 0s, the output is 0.
Usage example:
class BitwiseOR { public static void main(String args[]) { byte a = 0b00001010; //a=10 byte b = 0b00111001; //b=57 //00111011 = 59 byte c = a | b; System.out.println(a + ", " + b + "= " + c); } } //OUTPUT: 10, 57 = 59
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
4. Bitwise Exclusive OR Operator (^)
Bitwise XOR Operator (|) or Bitwise Exclusive OR operator gives an output of 1 if both the input bits are different i.e 1,0 or 0, 1. If both the input bits are same i.e 0,0 or 1,1, the output is 0.
Usage example:
class BitwiseXOR { public static void main(String args[]) { byte a = 0b00001010; //a=10 byte b = 0b00111001; //b=57 //00110011 = 51 byte c = a ^ b; System.out.println(a + ", " + b + "= " + c); } } //OUTPUT: 10, 57 = 51
Op1 | Op2 | Result |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
5. Bitwise Shift Left or Left Shift Operator Operator (<<)
Bitwise Shift Left or Right Shift operator shifts the individual bits from Right to Left. So gaps are formed on the right side. These gaps are filled with 0s. Left most bits are discarded.
Note: If discarded bits on the left side are zeroes, Left Shift operation is equivalent to multiplying the number by 2.
Usage example:
class BitwiseLeftShift { public static void main(String args[]) { byte a = 0b00001010; //a=10 //00010100 //20 byte b = (byte)(a << 1); System.out.println(a + ", " + b); byte c = 0b01010111; //87 //0101-01110000 //Left 4 bits discarded byte d = (byte)(c << 4); System.out.println(c + ", " + d); } } //OUTPUT: //10, 20 //87, 112
6. Bitwise Shift Right or Right Shift Operator Operator (>>)
Right Shift Operator shifts the individual bits of a number towards Right Side from the Left Side. Usually Left Most bits are filled with Zeroes in the process of filling gaps created because shifting bits to the right side. Shift Right operator does Sign Extension by putting the Left Most Bits to 1s if they are already 1s. So, negative numbers are still negative numbers after the Right Shift operation.
Note: Right Shift operation is equivalent to dividing the number by 2.
Usage example:
class BitwiseRightShift { public static void main(String args[]) { byte a = 0b00010100; //a=20 //00001010 //10 byte b = (byte)(a >> 1); //=20/2 System.out.println(a + ", " + b); byte c = 0b01010111; //87 //00000101-0111 //5 //Right 4 bits discarded byte d = (byte)(c >> 4); //=87/(2*2*2*2) System.out.println(c + ", " + d); byte e = (byte)0b11111000; //-8 //11111100 //-4 //Right 1 bit discarded byte f = (byte)(e >> 1); System.out.println(e + ", " + f); } } //OUTPUT: //20, 10 //87, 5 //-8, -4
7. Bitwise Shift Right Zero Fill Operator (or) Unsigned Right Shift Operator (>>>)
Unsigned Shift Right or Shift Right Fill Zero operator shifts the individual bits of a number from Left to Right. It fills Zeroes on the left side in the gaps created by shifting the digits to the right. This operator does not maintain Sign Extension. So, a negative number may become a positive number after the Right Shift Fill Zero operation.
Note: Right Shift Fill Zero operation is equivalent to dividing the number by 2.
Usage example:
class BitwiseRightShiftUnsigned { public static void main(String args[]) { byte a = 0b00010100; //a=20 //00001010 //10 byte b = (byte)(a >>> 1); //=20/2 System.out.println(a + ", " + b); byte c = 0b01010111; //87 //00000101-0111 //5 //Right 4 bits discarded byte d = (byte)(c >>> 4); //=87/(2*2*2*2) System.out.println(c + ", " + d); int e = -1;//(byte)0b11111111; //11111111 11111111 11111111 11111111 //-1 //00000000 00000000 00000000 00001111 //15 int f = (int)(e >>> 28); System.out.println(e + ", " + f); } } //OUTPUT: //20, 10 //87, 5 //-1, -15
This is all about Bitwise Operators in Java. You should practice these examples on your PC for better understanding.
Share this tutorial with your friends and colleagues to encourage authors.