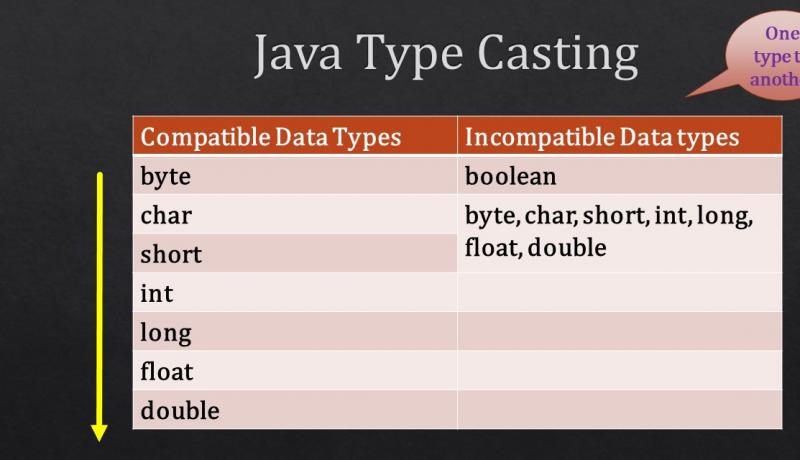
Java allows developers to work with different types of data. Java allows conversion of numeric data of type byte, short, char, int, long, float and double from one type to another type.
Automatic Type Conversion refers to both Automatic Type Promotions and Type Casting. Type Promotion is a Widening Conversion while Type Casting is a Narrowing Conversion. A widening conversion is also called Upward Casting and Narrowing Conversion is also called a Downward Casting.
Type Promotions or Type Conversions in Java
Implicit type conversion of a variable from one data type to another data type is called Type Promotion. Since it is a Type Promotion, the value of a variable is promoted to a compatible higher data type. This type of conversion is also called a Widening Conversion.
Note 1: Type Promotion is a Lossless Conversion. Data is not truncated.
Note 2: Some developers call this Automatic Type Conversion as Automatic Type Casting or Implicit Type Casting. Explicit Type casting or conversion is simply called Type Casting.
Numeric arithmetic expressions are automatically promoted to a higher data type provided the following two conditions or Rules are satisfied.
- Target data type should be compatible with the source data type.
- Target data type should be bigger in size than the source data type.
Compatible Data Types
All numeric data types are compatible for either Widening Conversion or Narrowing Conversion. The list is below.
- byte
- char
- short
- int
- long
- float
- double
Incompatible Data Types
Numeric to Non-numeric data types are incompatible for either Widening Conversion or Narrowing Conversions. The list is below.
- boolean
- byte, char, short, int, long, float, double
Automatic Type Promotion Scenarios
If one of the operands is of type long, the whole arithmetic expression gives a long value. If one of the operands is of type float, the whole arithmetic expression gives a float value. Lastly, if one of the operands is of type double, the whole arithmetic expression gives a double value.
Here is the list of possible Scenarios of how a data type is promoted automatically.
- byte to int
- char to int
- short to int
- int to long
- byte to long
- char to long
- short to long
- byte to float
- char to float
- short to float
- int to float
- byte to double
- char to double
- short to double
- int to double
- float to double
Example 1:
class TypePromotion1 { public static void main(String args[]) { short a = 120; byte b = 50; //120*50 is promoted to int automatically int c = a*b; System.out.println(c); int a = 4; byte d = a * 5; //ERROR //a*5 is converted to int //int to byte conversion is not automatic } }
Example 2:
class TypePromotion2 { public static void main(String args[]) { short a = 120; float b = 50.0f; //120*50.0f is promoted to float automatically float c = a*b; System.out.println(c); float d = 65; //65 is converted to float } }
Example 3:
class TypePromotion3 { public static void main(String args[]) { short a = 120; int b = 50; double c = 1.0; //120*50*1.0 is promoted to double automatically //short * int * 1.0 == double double e = a*b*c; System.out.println(c); double f = 35*1.0; //35*1.0 => int * double == double System.out.println(f); double g = 40; //40 is converted to double } }
Type Casting in Java
Type Casting is nothing but manually casting or converting an operand or constant or the value of an expression from one data type to another. Type Casting is explicit in nature and it is a Narrowing Type Conversion.
Note: Type Casting is a Lossy Conversion. Data is TRUNCATED.
You should check compatibility before casting the data (constant, value of expression) to another data type. Check the above list of Compatible and Incompatible data types under Type Promotions.
Numeric arithmetic expressions are manually demoted to a lower data type provided the following two conditions or Rules are satisfied.
- The target data type is compatible with the source data type.
- The target data type is smaller in size than the source data type.
Syntax of Type Casting
Syntax for explicit type casting includes Parentheses surrounding a Target Data Type and an immediately following Source Data Type.
(Target Data Type)Source Data Type Example: float a = 34.56; int b = (int)a;
Example 1:
Type casting from int to short, int to byte, int to char and char to int are shown below.
class TypeCasting1 { public static void main(String args[]) { int a = 30; //int to short type casting short b = (short)a; //int to byte byte c = (byte)a; //short to byte byte d = (byte)b; //int to char int num = 65; char ch = (char)num; System.out.println(ch); //prints A //char to int char ch2 = 'B'; int num2 = ch2; System.out.println(num2); //prints 66; } }
Example 2: Real World Example for Software Developers
Below example demonstrates how to retain only 2 digits of precision. You can use Format Specifiers to print only 2 digits after precision. But, the variable holds all the digits.
class TypeCasting2 { public static void main(String args[]) { //GOAL : PRINT : 21.42 int a = 150; int b = 7; //150/7 = 21.428572 float c = a/b; printf(c); //Unfortunately Output: 21.0 //TYPE PROMOTION //1.0f promotes int a to float value float d = (1.0f * a)/b; // 150.0/7 d = d* 100; //2142.8572 //TYPE CASTING d = (int)d; //lossy conversion d = 1.0f * d / 100; // 2142.0/100 System.out.println(d); //varible d holds only two precision digits //Output: 21.42 } }
This is how Java language implements Type Casting or Type Conversions which are essential to handle different types of input data.