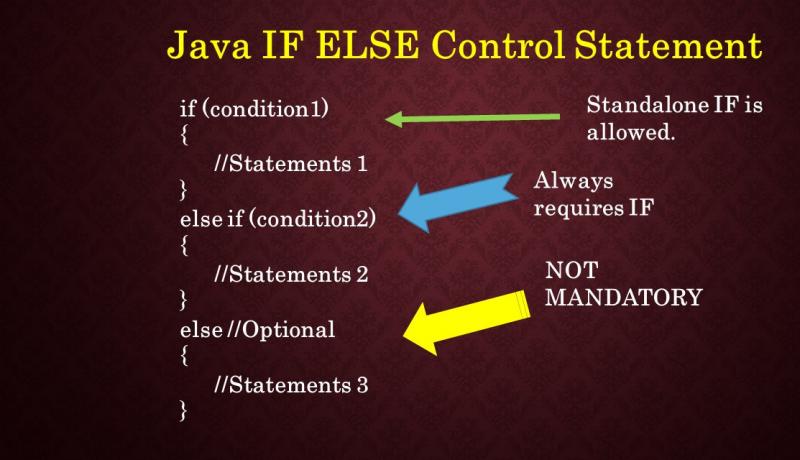
A Control statement in Java decides the code to be executed based on a condition. All control statements take the boolean value as an INPUT and branches control accordingly. Usually, the true condition takes control to one branch of code and the false condition takes control to another branch. The only exception to this is a SWITCH statement which can accept integers like byte, short, int and enum, character type data like char and String.
Java Control Statements
Java language has three types of Control Statements.
- Selection Statements
- Loop or Iteration Statements
- Jump Statements
1. Selection Control Statements
A Selection control statement takes a condition (boolean value or relational expression) as input and branches to one of the two possible branching point statements. If the condition is true, One set of instructions or statements are executed and if the condition is false, another set of instructions are executed.
There are two types of Selection Control Statements.
- IF statement (IF ELSE statements)
- SWITCH statement
2. Loop Control Statements
Loop control statements execute a particular set of instructions repeatedly as long as the Loop Condition is satisfied i.e true condition.
There are four types of Loop Control Statements
- WHILE
- DO WHILE
- FOR
- FOR EACH or Advanced FOR or Enhanced FOR
3. Jump Control Statements
Jump control statements take the execution control by skipping a number of statements in between. Unlike other control statements, which go in a predictable linear way of execution, Jump control statements work in a non-linear nested way by skipping and jumping a number of statements.
There are three Jump Control statements.
Java IF, ELSE and ELSE IF control statements
Java language continues the same features of IF ELSE statements introduced by the C Language. Programmers heavily depend of IF ELSE statements to create good java products with efficiency. Remember that you can put any number of white spaces like TAB, New Line and Space between two tokens or keywords of a Java program. You can write all IF statements of a program in just one line though it is not reading friendly.
1. IF Control Statement
IF statement takes a condition as an input. This condition should evaluate to a boolean type constant like true or false. If you do not use Braces, only one statement or Single statement can be specified inside an if statement. With the use of Braces, you can add any number of statements inside a Single IF statement.
If the condition evaluates to true, statements below IF will be executed. Otherwise, program control goes to the next statement after the IF block.
Syntax:
if( CONDITION ) //STATEMENT; (or) if( CONDITION ) { Statement1; Statement2; ... }
Example: IF statement
The second statement is not part of IF(a<10). Use braces to add if required.
class IFStatement { public static void main(String args[]) { int a=11; if(a<10) System.out.println(a + "<10"); System.out.println("AFRICA"); int b=8; if(b<10) { System.out.println(b + "<10"); System.out.println("POLAND"); } } } //OUTPUT //AFRICA //8<10 //POLAND
2. IF ELSE Control Statement
ELSE statement is a companion to the IF statement. You can not use ELSE without using the IF statement. ELSE block is executed only if IF condition fails or it is false.
Syntax:
if( CONDITION ) //STATEMENT1; else //STATEMENT2 (or) if( CONDITION ) { Statement1; Statement2; ... } else { Statement8; Statement9; ... }
Example: IF ELSE statement with String comparison
"str" is compared with "str2". Both the String variables hold the same value "ELEPHANT". So, IF condition evaluates to true and the corresponding statements are executed.
class IF_ELSE_Statement { public static void main(String args[]) { String str="ELEPHANT"; String str2= "ELEPHANT"; if(str == str2) { System.out.println("IF Condition"); } else { System.out.println("ELSE Condition"); } if(str != str2) { System.out.println("IF Condition"); } else { System.out.println("ELSE Condition"); } } } //OUTPUT //IF Condition //ELSE Condition
3. IF ELSE IF Control Statement
IF ELSE-IF ladder is useful to execute a different set of statements for different conditions. We can use as many ELSE-IF statements as we want. Do note that the ELSE statement or block at the end of IF-ELSE-IF is not mandatory or it is optional.
If any of IF or ELSE IF condition matches control goes inside that IF or ELSE IF and then exits without going inside ELSE block at the last.
"float" is promoted to type "double" in this example.
Example:
public class IF_ELSE_IF { public static void main(String[] args) { float a = 1.5f; float b = 2.5f; if(a > 2) { System.out.println("A>2"); } else if(b>2) { System.out.println("B>2"); } else if(a>1) { System.out.println("A>1"); } else { System.out.println("UNKNOWN"); } } } //OUTPUT //B>2
4. NESTED IF, ELSE and ELSE-IF Control Statements
You can nest an IF inside another IF. You can nest an IF ELSE inside another IF. You can nest IF ELSE inside another ELSE or ELSE-IF and so on. Carefully use braces to demarcate one block from another block. You can add as many conditions as you want. Some programmers write multiple conditions on a single line with the help of relational operators and a single IF.
Example:
public class NESTED_IF { public static void main(String[] args) { float a = 1.5f; float b = 2.5f; if(a > 1) { if(b>=2) { System.out.println("A>1 AND B>2"); } else { System.out.println("A>1 AND B<2"); } } else if(a>2) { System.out.println("A>2"); } else { System.out.println("UNKNOWN"); } } } //OUTPUT //A>1 AND B>2
In the next chapters, we shall discuss Java Switch Statement and Loops in detail.
Share this article with your friends and colleagues to encourage authors.