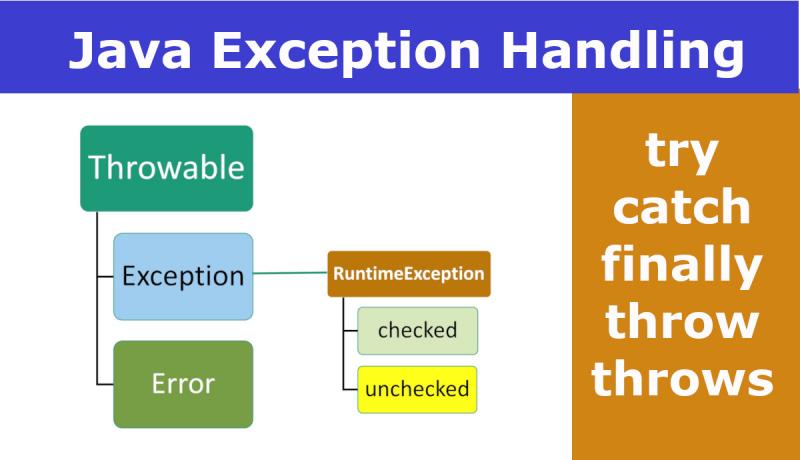
Java Exceptions are runtime errors that stop the execution of the program suddenly. Java Exception handling is done using the keywords namely try, catch, finally, throw and throws. Let us learn more about how to throw exceptions and catch exceptions in this Last Minute Java Tutorial using good example programs.
Java Exception Handling explained
At the time of compiling a Java program, some errors will come complaining about bad syntax. At the time of running the Java program too, some errors may come that spoil user experience creating confusion. So, these exceptions (errors actually) should be handled properly in order to run the program continuously and smoothly.
Java language created a top-level error Class namely Throwable. The two main subclasses of the superclass Throwable are Exception and Error. Finally, RuntimeException subclassed the class Exception. Most of the Java runtime exceptions are subclasses of this RuntimeException. This is also called Exception Hierarchy.
There are two types of Runtime Exceptions namely Checked and Unchecked. The compiler does not check and complain about properly handling Unchecked Runtime exceptions. But the same Java compiler complains about mishandling Checked Runtime Exception.
Let us discuss the syntax and usage of each keyword to handle Java exceptions.
1. Java try block
A Java try block encloses the code that is doubtful to cause runtime errors or exceptions. It is the duty of the developer to identify such risky code blocks and use a try statement. The try should be used in conjunction with a catch or finally block. Otherwise, a compiler error is generated.
Note: A Java TRY statement is comparable to an IF statement waiting for exceptions to happen at runtime and report.
Syntax:
try { //code statements }
Example 1:
int ary[] = {10, 20, 30}; try { int b = ary[5]; //array index 5 does not exist }
We tried to access using the wrong index. So, the try block asks JVM to throw an Exception object.
2. Java catch block
Java catch block does the actual work of safely catching the exception object generated in a TRY block and receiving a reference for you inspect. The Exception object contains useful methods like getMessage, printStackTrace, getCause, initCause and more. The catch statement should always be accompanied by a try statement.
Syntax:
catch(EXCEPTION_TYPE obj) { //code statements }
Example 2:
int ary[] = {10, 20, 30}; try { int b = ary[5]; } catch(ArrayIndexOutOfBoundsExceptione ae) { ae.printStackTrace(); } catch(Exception e) { e.printStackTrace(); } //OUTPUT java.lang.ArrayIndexOutOfBoundsException: 5 at abc/com.programs.ExceptionExample1.main(ExceptionExample1.java:11)
The printStackTrace method is useful for debugging the Java program. It displays the line numbers, method-names and class-names of all sources of exception.
Just like nesting IF-ELSE statements, Java TRY-CATCH statements can also be nested one inside the another. If an exception occurs, JVM tries to find a suitable catch statement from inner to outer catch statements.
In the above program, multiple catch blocks are used one below another. Remember that you need a catch a Subclass type exception before a Superclass type exception. Otherwise, the compiler error code-not-reachable-error is created.
3. Java Finally block
The problem with a Java exception is that the remaining lines of code below the line causing an exception are skipped by the JVM (Java Virtual Machine ) or runtime. If some important code like for closing a File resource is present, that will not be executed causing memory mismanagement or leak.
We can not depend on the Java Garbage collector (GC) to free the memory as GC is not time guaranteed. The purpose of a Java finally block is that the code kept inside the FINALLY block is guaranteed to be executed whether an exception occurs or not. It is executed even if a conditional return statement is present to return from a method suddenly.
A Java finally-block should be accompanied by a try or try-catch block. It can not be used alone.
Syntax:
finally { //code statements }
Example Program:
public class ExceptionExample2 { void calc() { int a = 10, b=0; try { int c = a/b; //This code is not executed System.out.println("After exception.."); } catch(Exception e) { System.out.println("Exception: " + e.getMessage()); } finally { System.out.println("Inside Finally Guaranteed.."); } } public static void main(String[] args) { ExceptionExample2 obj = new ExceptionExample2(); obj.calc(); } } //OUTPUT Exception: / by zero Inside Finally Guaranteed..
In the above example, the print statement after the ArithemeticException Divide by Zero is not executed. The finally-block is always executed in all situations for any Java exception.
4. Java throws statement
Java throws statement next to a method-name tells the compiler that an exception may be thrown. Any code that calls this method will be checked for a try-catch block by the compiler.
Syntax:
return-type METHOD_NAME(Params) throws Exception_Type { //statements }
So, a method can throw any type of exception. If a method mentions that it is throwing an exception, it means that the try-catch employed by the method may be missing or incapable of catching exceptions generated inside the method. In that case, it is the duty of the developer to employ such try-catch blocks when calling such risky methods.
Example Program:
class YException extends Exception { public String getMessage() { return "YException occurred."; } } public class ExceptionExample1 { //this method throws unchecked exception. //No compiler error for directly calling this although the exception breaks the program. void show() throws ArithmeticException { int c = 1/0; } void someMethod1() throws YException { try{ int c = 10/0; } catch(Exception e) { throw new YException(); //throw statement } } void someMethod2() throws YException { throw new YException(); //throw statement } public static void main(String[] args) { ExceptionExample1 obj = new ExceptionExample1(); try{ obj.show(); } catch(Exception e1) { System.out.println(e1.getMessage()); } try{ obj.someMethod2(); } catch(Exception e2) { System.out.println(e2.getMessage()); } } } //OUTPUT / by zero YException occurred.
User-defined exception YException in the above program is treated as a checked runtime exception. So the compiler always expects a try-catch for calling methods throwing such exceptions. To throw an exception, you need to use the keyword "throw" and an object next to it.
5. Java throw statement
To throw a Java exception from within a method, you can use the keyword "throw" and an exception object. A throw statement is usually accompanied by a throws-statement. Above example explains this clearly. Most of the times, this Java throw statement is also called a rethrow statement as you can throw the same or different exception object again.
List of Unchecked Java RuntimeException classes
Below is the list of frequently seen unchecked Java RuntimeException classes.
- NullPointerException
- ArithmeticException
- ArrayIndexOutOfBoundsException
- IndexOutOfBoundsException
- ArrayStoreException
- ClassCastException
- IllegalArgumentException
- IllegalThreadStateException
- StringIndexOutOfBoundsException
List of checked Java RuntimeException classes
Below is the list of frequently seen checked Java RuntimeException classes.
- ClassNotFoundException
- NoSuchMethodException
- InterruptedException
Share this Last Minute Java Exception Handling Tutorial with your friends and colleagues to encourage authors.