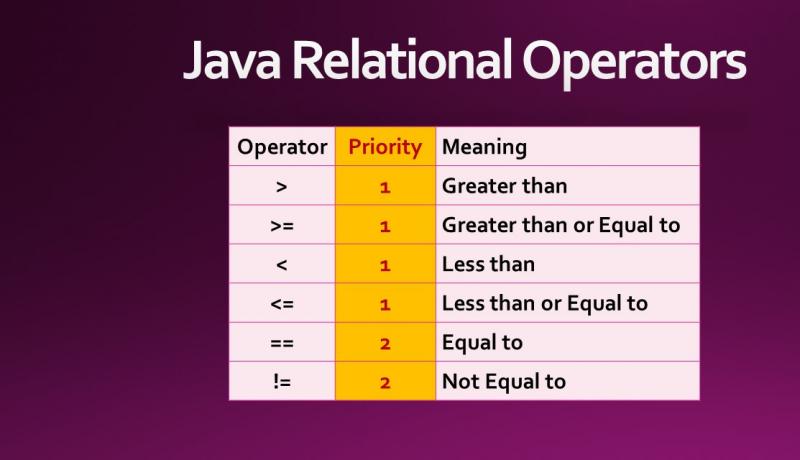
Java language comes with Relational Operators to compare two expressions, variables or constants. These Relational operators are also called Comparison Operators or Conditional Operators. Conditional operators are used inside Conditional Control Statements like IF, ELSE, ELSE IF and SWITCH.
Java Relational Operators or Comparison Operators
A Java relational operator checks the relationship of one expression with that of another expression like more, less or equal etc. The output of a Comparison or Relational Operation is a boolean data type Value. Relational operators return true for successful check and false for the unsatisfying condition. There are Six Relational operators in Java language.
You can use Relational operators with the data types like char, boolean and number data types like byte, short, int, long, float and double. You can not use Strings with relational operators directly without using String class methods like length. "char" data type value is simply treated as a number and comparison is performed.
NO | Operator | Meaning |
---|---|---|
1 | > | Greater than |
2 | >= | Greater than or Equal to |
3 | < | Less than |
4 | <= | Less than or Equal to |
5 | == | Equal to |
6 | != | Not Equal to |
1. Greater Than Operator
Greater Than Operator (>) checks if the left operand is greater than the right side operand.
2. Greater Than or Equal to Operator
Greater Than or Equal To Operator (>=) checks for two things namely Greater Than and Equal To.
3. Less Than Operator
Less Than operator (<) checks if the left side operand is less than the right side operand.
4. Less Than or Equal To Operator
Less than or Equal to Operator (<=) checks for two things namely Less Than and Equal To.
5. Equal To operator
Equal To operator (==) checks whether left and right side operands are equal or not.
6. Not Equal To Operator
Not Equal To Operator (!=) checks the opposite condition of Equal To (==) operator. It checks for Unequal or Not Equal to Condition first and returns either true or false.
Example 1: Relational Operators
class RelationalOperators { public static void main(String args[]) { int a=5; int b=20; if(a >= 4) System.out.println("a>=4"); if(b == 20) System.out.println("b is 20."); if(a < 20) System.out.println("LESS"); else System.out.println("MORE"); } } //OUTPUT:: //a>=4 //b is 20. //LESS
Example 2: Relational Operators with char
class RelationalOperators2 { public static void main(String args[]) { char a ='A'; //65 Unicode char b = 'b'; //98 Unicode if(a > b) System.out.println("A > b"); else System.out.println("A < b"); } } //OUTPUT:: //a<b
Example 3: Relational Operators with Switch Statement
You can only use String, char and integer type data as an INPUT to switch() statement. You can use Relational Operators to construct CASE Constants in a Switch case as shown in this example. With the help of Java Ternary operator, we have used Relational or Comparison operators inside a SWITCH statement. Without the "final" keyword, this program does not work as SWITCH expects only constants.
class RelationalOperators3 { public static void main(String args[]) { final int c = 5; switch(c<10?c:2) { case (c<10?c:2): System.out.println("C < 10"); break; default: System.out.println("OUTSIDE"); } } } //OUTPUT:: //C < 10
Java Relational or Comparison Operators Priority or Precedence
Notice that Equal or Not Equal to Operation has got less priority than Greater Than, Greater Than or Equal to, Less than and Less than or Equal to Operations.
Priority | Operator | Meaning |
---|---|---|
1 | >, >=, <, <= | Greater than, Greater than or Equal to, Less than, Less than or Equal to |
2 | ==, != | Equal to, Not Equal to |
Example 4: Comparison Operators with boolean and numbers
In the below example, > has more priority than ==. So, a is compared with b first. In the end, the result of the first relational expression is compared with false.
class ComparisonOperators { public static void main(String args[]) { int a=5; int b=20; boolean bill = a > b == false; System.out.println(bill); } } //OUTPUT:: //true //(a > b) == false //(false) == false //true
In the next chapters, you will learn about Bitwise Operators and Logical Operators.
Get Ready.