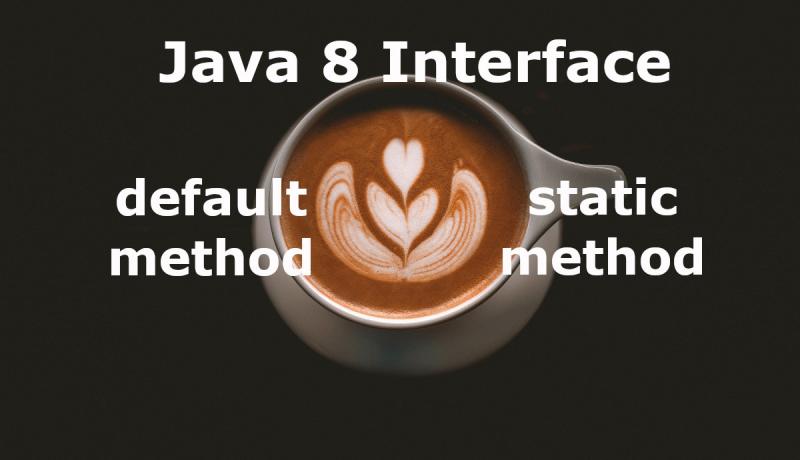
Java 1.8 or Java 8 introduced new features in Interface namely STATIC method and Java DEFAULT method. These are against the concept of an interface is a 100% abstract class. Let us know more with examples in this Last Minute Java Tutorial.
Note: The keyword "default" is also used with SWITCH CASE control statements.
Java Interface DEFAULT method
The default method in a Java interface is a concrete (non-abstract) method that will be called at runtime if the implementation is missed by the implementor class due to Source code changes.
A Java default method can not be declared static or abstract or private or protected. The compiler throws an error. It uses the public access modifier by default.
There are two things Java developers take care of.
- Updating the project Runtime Environment with the latest Java libraries containing classes and interfaces.
- Updating the own project code and compiling it to avail the new features.
Java interface default method avoids the need for updating the own source code immediately. So, the Java Runtime does not throw an exception for missing implementations for new abstract methods found in the updated interfaces.
Syntax:
default return-type METHOD_NAME(Parameters) { //Default code implmentation }
A Java default method allows a developer to add new functionality or feature to the Source code without bothering about breaking the dependencies and usages. So, developers can use either Old runtime or new runtime libraries to successfully run the existing project or program. You can override the default method of the interface later at the time of updating your implementor-class.
Look at the below example program that clearly explains Java default method usage in the real world.
DefaultMethodExample.java
interface Phone { void backCamera(); //developer added this method in next update //we are still using the old version code. default void frontCamera() //Will not break existing implementations { System.out.println("Front camera not supported yet."); } } class ABCPhone implements Phone { public void backCamera() { System.out.println("Back Camera supported."); } } public class DefaultMethodExample { public static void main(String[] args) { ABCPhone ap = new ABCPhone(); ap.backCamera(); ap.frontCamera(); //no error } } //OUTPUT Back Camera supported. Front camera not supported yet.
In the above example, the developer implemented only the first abstract method backCamera() of the interface Phone. He is happy that it works successfully. Later, someone has upgraded the Phone interface to support another method "frontCamera()". If it is an abstract method, it breaks all the existing implementations of the Phone interface. So, Java-8 simply added the keyword "default" in the place of "abstract" before the new method. Now, all existing implementations run successfully of course without the new method frontCamera().
Below example program overrides the default method in an Interface. To override any method of an interface including the default method, use the public access modifier to escape compiler errors. This is the next version to the above program. The default method "frontCamera()" is overridden by the ABCPhone class now.
interface Phone { void backCamera(); //developer added this method in next update //we are still using the old version code. default void frontCamera() //Will not break existing implementations { System.out.println("Front camera not supported yet."); } } class ABCPhone implements Phone { public void backCamera() { System.out.println("Back Camera supported."); } public void frontCamera() //overriding default method { System.out.println("Front camera supported."); } }
Java Interface STATIC method
A Java interface can not be instantiated to create objects. So, "new" keyword can not be used. You can access the variables of an interface without even implementing the interface as these are public static final constants. Java-8 introduced static methods in an interface just to make some methods available with readymade code. These methods can be accessed without even implementing the interface.
Java interface static methods can not be declared abstract or final. Access modifiers protected or private can not be used. Only public access modifier is allowed. Static methods are concrete methods with the body. You can directly call a static method of an interface using a DOT operator in between Interface-name and method-name.
You can not override a static method of an interface. The compiler shows error.
Syntax:
static return-type METHOD_NAME(Parameters) { //method body with code }
Access modifier "public" is optional before the static method of an interface.
Look at the example program that explains the usage of Java interface static methods.
StaticMethodExample.java
interface Curtain { static void show() //abstract not applicable { System.out.println("Showing Curtain.."); } void changeCurtain(); //abstract method } class CottonCurtain implements Curtain { public void changeCurtain() //implementing { System.out.println("Changed White Curtain.."); } } public class StaticMethodExample { public static void main(String[] args) { Curtain.show(); CottonCurtain cc = new CottonCurtain(); cc.changeCurtain(); //cc.show(); //error. Can not inherit the method } } //OUTPUT Showing Curtain.. Changed White Curtain..
In the above program, Curtain is an interface. CottonCurtain is an implementor class. The method "show()" is static inside the interface. You can call this static method using Curtain.show() statement.
Note that you can not access the static method directly using an instance of the implementing class. Java allows accessing the static method directly using the Interface-name and a DOT operator.
Share this Last Minute Java tutorial on Java Static Method and Java Default Method with your friends and colleagues.
1. | Java Interface rules examples |
2. | Java Interface with default method and static method |
3. | Difference between Abstract Class and Interface |