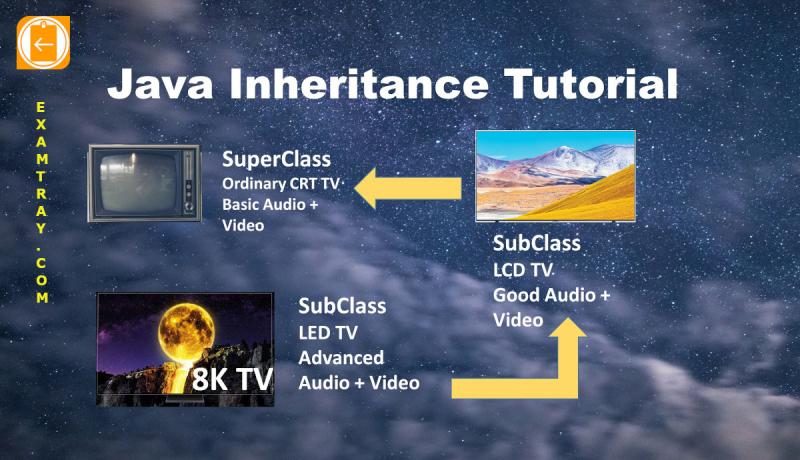
As Java is an Object Oriented Programming (OOP) language, it supports Inheritance. Inheritance is a process of acquiring Methods and Properties of an existing class into a custom class written by us so that all the inherited code becomes part of our custom Class. Here, the already existing Class is called a Superclass and the custom Class written by us is called a Subclass. Let us know more about Java Inheritance with Superclass and Subclass relationship and usage with examples in this Last Minute Java Tutorial.
Java Inheritance with Superclass and Subclass Examples
Let us take Television or TV as our Superclass which is already existing with some methods and properties. Properties are simply variables.
Java allows inheritance only through the "extends" keyword. The class being inherited is called a Superclass and the class inheriting the Superclass is called a Subclass.
Syntax:
class Subclass extends Superclass { //some code }
We explain Superclass-Subclass relationship using a TV example. As the technology evolved, more and more features were added to the basic Superclass TV. There came an LCD TV with Good Audio and Video. Then came LED TV with even good Video and true Dolby Audio. Additions like Smart TV with an Android operating system on top of it made the Basic TV like a modern computer. TVs now support Bluetooth, Wifi, Ethernet and HDMI input outputs. All these additions can be thought as extra Properties defined by the Subclasses with each Subclass defining a new feature over time.
All Subclasses have access to the properties and methods of the Superclasses depending on the access level defined by an access modifier like public, protected or private.
A Subclass can become a Superclass to another class down the hierarchy. The chain continues up to any level with the last class being a Subclass to the Class one level up.
At any point in time, a Class can inherit only one Class through extends keyword.
Let us define our example superclass CRT_TV. It defines two properties or variables audioChannels and resolution.
class CRT_TV { char category='A'; int audioChannels = 1; int resolution = 640*480; //480p void showVideo(int res) { System.out.println("Showing Video Pixels " + res); } }
Now, we shall extend or inherit the class CRT_TV from LCD_TV class. CRT_TV becomes a Superclass to the LCD_TV Subclass.
class LCD_TV extends CRT_TV { int audioChannels = 2; int resolution = 1280*720; //720p void connectBluetooth() { System.out.println("Connecting Bluetooth"); } void show2() { //super keyword to avoid name clash System.out.println("Access Super Resolution= " + super.resolution); } }
LCD_TV subclass defined an extra feature using "connectBluetooth" method. It also improved existing features like audioChannels and resolution of the Superclass CRT_TV. Subclass LCD_TV has access to the Superclass's method "showVideo" and variables audioChannels, resolution and category. Notice that we have overwritten (overridden) the two properties "audioChannels" and "resolution". Yes, Java inheritance allows customizing the inherited methods too.
Let us use the above classes into a testing class "TestingInheritance".
class TestingInheritance { public static void main(String[] args) { CRT_TV crt = new CRT_TV(); crt.showVideo(crt.resolution); LCD_TV lcd = new LCD_TV(); System.out.println("Accessing Superclass category= " + lcd.category); lcd.showVideo(lcd.resolution); //accessing superclass method lcd.show2(); //try to know superclass resolution lcd.connectBluetooth(); //crt.connectBluetooth(); //error: can not access Subclass's methods with a superclass reference. System.out.println("LCD Audio Channels= " + lcd.audioChannels); System.out.println("CRT Audio Channels= " + crt.audioChannels); } } //OUTPUT Showing Video Pixels 307200 Accessing Superclass category= A Showing Video Pixels 921600 Access Super Resolution= 307200 Connecting Bluetooth LCD Audio Channels= 2 CRT Audio Channels= 1
Notice that we have accessed a variable "resolution" which is present in both the Superclass and Subclass. You can directly access the own class's variables. In Java, to access a Superclass's variable, use the keyword "super" to avoid a name clash with the current instance variable. If the names or identifiers are different, use the variables directly.
We shall learn more about Overriding Methods in the coming chapters using the same Java Inheritance concepts explained above.
Share this Last Minute Java Inheritance Tutorial with your friends and colleagues to encourage authors.