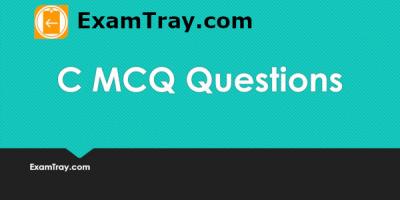
Study C MCQ Questions and Answers on Strings, Character Arrays, String Pointers and Char Pointers. Easily attend technical job interviews after practising the Multiple Choice Questions.
Go through C Theory Notes on Strings before studying questions.
char ary[]="Hello..!";
It is a simple way of creating a C String. You can also define it like the below. \0 is mandatory in this version. char ary[] = {'h','e','l','l','o','\0'};
char ary[]="Hello..!"; printf("%s",ary);
int main() { char ary[]="Discovery Channel"; printf("%s",ary); return 0; }
%s prints the while character array in one go.
int main() { char str[]={'g','l','o','b','e'}; printf("%s",str); return 0; }
Notice that you have not added the last character \0 in the char array. So it is not a string. It can not know the end of string. So it may print string with some garbage values at the end.
int main() { char str[]={'g','l','o','b','y','\0'}; printf("%s",str); return 0; }
Adding a NULL or \0 at the end is a correct way of representing a C string. You can simple use char str[]="globy". It is same as above.
char str[]={'g','l','o','b','y'};
int main() { int str[]={'g','l','o','b','y'}; printf("A%c ",str); printf("A%s ",str); printf("A%c ",str[0]); return 0; }
Notice that STR is not a string as it is not a char array with null at the end. So STR is the address of array which is converted to Char by %c. If you use %s, it prints the first number converted to char.
int main() { char str[]={"C","A","T","\0"}; printf("%s",str); return 0; }
Yes. You can not use Double Quotes " to represent a single character. Correct way is 'C' not "C". You should use Single Quotes around a single character constant.
Maximum size of a C String is dependent on implemented PC memory. C does not restrict C array size or String Length.
int main() { char str1[]="JOHN"; char str2[20]; str2= str1; printf("%s",str2); return 0; }
You can not assign one string to the other. It is an error. "
error: assignment to expression with array type
int main() { char str[25]; scanf("%s", str); printf("%s",str); return 0; } //input: South Africa
SCANF can not accept a string with spaces or tabs. So SCANF takes only South into STR.
int main() { char str[2]; scanf("%s", str); printf("%s",str); return 0; } //Input: South
In C Arrays, Overflow or Out of Bounds is not checked properly. It is your responsibility to check.
int main() { char str[2]; int i=0; scanf("%s", str); while(str[i] != '\0') { printf("%c", str[i]); i++; } return 0; } //Input: KLMN
It always overwrites the next memory locations of the array. It is your responsibility to check bounds. Scanf automatically adds a '\0' at the end of entered string.
int main() { char country[]="BRAZIL"; char *ptr; ptr=country; while(*ptr != '\0') { printf("%c", *ptr); ptr++; } return 0; }
*ptr != '\0' is the main part of traversing a C String.
Yes. gets(str) fills the array str with the input given by the user.
int main() { char *p1 = "GOAT"; char *p2; p2 = p1; printf("%s", p2); }
Yes. You can assign one String pointer to another. But you can not assign a normal character array variable to other like STR2 = STR1. It is an error.
int main() { char *p1 = "GOAT"; char *p2; p2 = p1; p2="ANT"; printf("%s", p1); }
*p1 and *p2 are completely pointing different memory locations. So, p1 value is not touched.
int main() { char p[] = "GODZILLA"; int i=0; while(p[i] != '\0') { printf("%c",*(p+i)); i++; } }
Notice the usage of *(p+i). Remember that, p[i] == *(p+i) == *(i+p) == i[p]