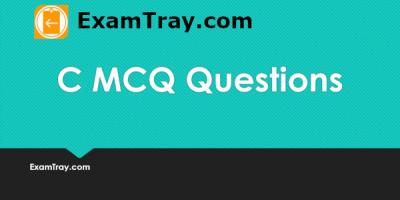
Learn C Programming MCQ Questions and Answers on Conditional Statements like Ternary Operator, IF, ELSE and ELSE IF statements. Easily attend exams after reading these Multiple Choice Questions.
Go through C Theory Notes on Conditional Operators before studying questions.
?: = Question Mark Colon is also called C Ternary Operator.
If the condition is true, expression 1 is evaluated. If the condition is false, expression 2 is evaluated.
int main() { int a=0; a = 5<2 ? 4 : 3; printf("%d",a); return 0; }
5<2 is false. So 3 will be picked and assigned to the variable a.
int main() { int a=0; a = printf("4"); printf("%d",a); return 0; }
a = printf("4");
First printf prints 4. printf() returns 1. Now the variable a=1; So 1 is printed next.
int main() { int a=0; a = 5>2 ? printf("4"): 3; printf("%d",a); return 0; }
5>2 is true. So expression1 i.e printf("4) is executed printing 4. Function printf() returns 1. So a value is 1.
int main() { int a=0; a = (5>2) ? : 8; printf("%d",a); return 0; }
expression1 = empty
expression2 = 8
If no expression is specified, it will be treated as 1.
int main() { int a=0, b; a = (5>2) ? b=6: b=8; printf("%d %d",a, b); return 0; }
Compiler error. a = (5>2) ? b=6: b=8; should be written as a = (5>2) ? b=6: (b=8);
main.c: In function ‘main’: main.c:14:23: error: lvalue required as left operand of assignment a = (5>2) ? b=6: b=8; ^
if( condition ) { //statements; }
if( condition ) { //statements; } else { //statements; }
if( condition1 ) { //statements; } else if( condition2) { //statements; } else { //statements; }
int main() { if( 4 > 5 ) { printf("Hurray..\n"); } printf("Yes"); return 0; }
if condition fails. So control will not enter Hurray printf statement.
int main() { if( 4 > 5 ) printf("Hurray..\n"); printf("Yes"); return 0; }
To include more than one statement inside If block, use { } braces. Otherwise, only first statement after if block is included. IF condition fails with false. So second if which is outside of If is executed.
int main() { if( 4 < 5 ) printf("Hurray..\n"); printf("Yes"); else printf("England") return 0; }
If block includes only Single Hurray printf statement without curly braces { }. So second Yes printf statement is not part of IF block. Else should immediately follow IF block. Otherwise, compiler throws errors. To compile well, use { } braces for two printf statements or remove second printf after IF.
int main() { if( 10 < 9 ) printf("Hurray..\n"); else if(4 > 2) printf("England"); return 0; }
You can omit ELSE comfortably. Compiler will not complain above ELSE after IF or ELSE IF.
int main() { if( 10 > 9 ) printf("Singapore\n"); else if(4%2 == 0) printf("England\n"); printf("Poland"); return 0; }
Observe that Poland printf is not under ELSE IF as there are two statements without curly braces { }. IF condition is TRUE. So, ELSE IF will not be seen at all even though 4%2 == 0 is true.
int main() { if(-5) { printf("Germany\n"); } if(5) { printf("Texas\n"); } printf("ZING"); return 0; }
You can use any number inside IF as a condition.
Positive Number or Negative Number evaluates to true.
Number 0 Zero evaluates to false.
int main() { if(10.0) { printf("Texas\n"); } printf("ZING"); return 0; }
You can use either Integer or Real numbers. 0 or 0.0 evaluates to false condition.
int main() { if("abc") { printf("India\n"); } if('c') { printf("Honey\n"); } printf("ZING"); return 0; }
"abc" is string and it returns an Integer Address Number. 'c' returns an ASCII number which is also a number. Any Non-Zero number gives TRUE condition.
int main() { if(TRUE) { printf("India\n"); } if(true) { printf("Honey\n"); } printf("ZING"); return 0; }
There are no keywords (true) or (TRUE). These available in Java, JavaScript and other languages.