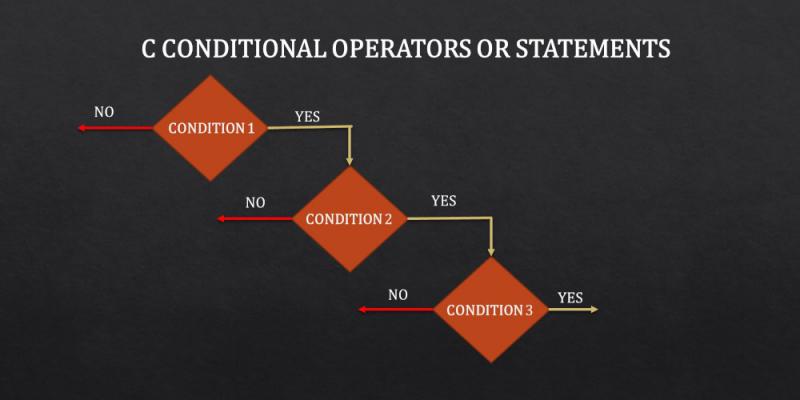
In real world, solution to any problem relies on the decision we make. Decision is nothing but a condition. C language has conditional operators or statements to deal with conditions or to make decisions. A condition is as simple as an Equals To comparison. Conditional operators are also called Relational Operators in c language.
If a statement satisfies a particular condition, one set of statements are executed. Otherwise another set of instructions are executed. Here, keep in mind that the number of conditions are more than 2 in most of the situation.
Result of a conditional statement is either true or false. Any Non Zero value is always treated as TRUE. Zero is treated as false.
We shall explain Ternary Conditional Operator ?: ( Question Mark Colon ) at the end of this tutorial.
List of popular Conditional or Relational operators in C programming
Note that there is no space between any individual single operators. = = is not equal to == operator for example.
SNO | Conditional Operator | Description |
1. | < | Less than |
2. | <= | Less than or equal to |
3. | > | Greater than |
4. | >= | Greater than or equal to |
5. | == | Equals to |
6. | != | Not Equal to |
Conditional statements in C language are implemented using IF, ELSE IF and ELSE statements. Note that these keywords should be written in lower case always.
Various combinations of if-else statements in c programming language are as below.
if(condition1) { //statements1; } //---------------------- if(condition1) { //statements1; } else { //statements2; } //---------------------- if(condition1) { //statements1; } else if(condition2) { //statements2; } //--------------------- if(condition1) { //statements1; } else if(condition2) { //statements2; } else { //statements3; }
Note: It is a good practice to keep opening and closing Braces { } for each IF block or ELSE block for clear understanding and to avoid mistakes. It is a must to use braces if more than 1 statement is present inside an IF or ELSE block. We used the word "block" to represent block of code under single IF or ELSE.
Observations on usage of IF, ELSE IF and ELSE:
- There is a SPACE between ELSE and IF. so ELSEIF is wrong.
- You can use only IF statement without ELSE.
- You can use IF, ELSE IF statements without ELSE at the end.
- For single line statements, curly braces are not required.
- You need not use ELSE IF always. ELSE is useful to check second or higher condition after first IF.
- You can nest IF, ELSE IF and ELSE statements inside any one.
Example 1:
int main() { int a=10; if(a>10) printf("YES"); else printf("NO"); return 9; } //output: NO //single statement need not be put inside braces{ }
Example 2: Need for Braces { }
int main() { int a=10; if(a>10) printf("YES"); printf("-ANT"); else printf("NO"); return 9; } //Output: error // to put more than one statement inside // if or else block, use braces { } // if(a>10) // { printf("YES"); // printf("-ANT"); // }
Example 3:
int main() { int a=8; if(a==9) printf("YES"); else if(a != 8) printf("NO"); else printf("ANT"); return 9; } //output: //ANT
Example 4: Nesting of IF, ELSE IF and ELSE
int main() { int category=9; if(category < 10) { if(category > 5) { printf("SUPERVISOR and above"); } else { printf("UP to CLERK cadre."); } } else { printf("MANAGER and above."); } return 9; } //output: // category=9; //output= SUPERVISOR and above. // //category=10; //output= MANAGER and above.
Ternary Operator ?: Operator
Syntax for Ternary Operator: (Condition) ? (True Part Expression) : (False Part Expression) ;
Example 1:
int main() { int a=20; (a >= 20)? printf("YES"): printf("NO"); return 0; } //output: YES
You can keep printf statements in true and false parts of a Ternary Operator. Semicolon at the end is compulsory as it will tell the compiler about end of a statement.
Example 2: Nesting of Ternary Operator
int main() { int a=20; (a >= 20)? (a!20 ? printf("YES"):printf("CARROT")): printf("ANT"); return 0; } //output: CARROT
Nesting of Ternary operator is achieved through proper use of Parantheses ( ) to separate True Part Expression and False Part Expression.
Example 3: Assignment Statements with Ternary Operator
int main() { int a=20; int c = a > 10 ? 5 : 8; printf("%d", c); return 0; } //output: 5
We have not used any parantheses in the above assignment operation using ternary operator.
Example 4: Assignment Statements with Ternary Operator
int main() { int a=20, b=0; int c = a > 10 ? 5 : b=8; printf("%d", c); return 0; } //output: ERROR //correct statement (b=8) with parantheses
In the example above we are making two assignments, one to c variable and another to b variable at the end. Ternaray operator should be used carefully with proper parantheses to separate code parts. Adding a parantheses ( ) to b=8 solves the problem.
C programming online tests on Conditional Operators or Statements
1 | Conditional Statements Operators - Online Test 1 |
2 | Conditional Statements Operators - Online Test 2 |