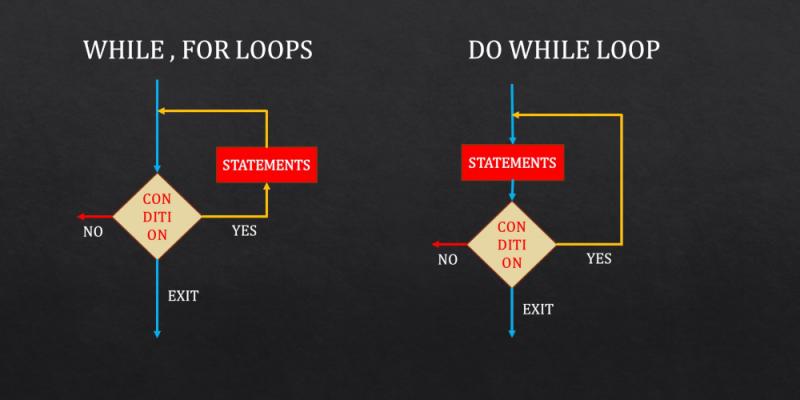
Loops help to repeatedly execute a set of instructions as long as loop condition is satisfied. Condition can be a combination of relational operators and logical operators.
C language comes with three loops.
- while loop
- for loop
- do while loop
C language provides two more keywords BREAK and CONTINUE to provide more control over loops.
break; Statement
break; statement with a semicolon at the end breaks the current loop where it is present. It can not affect outer loop in a nested loop program.
continue; Statement
continue; statement with a semicolon at the end skips executing next statements in the current loop and execution goes to the beginning of loop with next loop counter.
Increment Operator
++ is called Increment operator. It adds +1 to the variable.
Decrement Operator
-- is called Decrement operator. It subtracts -1 from the variable.
1. WHILE Loop
'while' loop executes a group of statements under the loop until a loop condition is true. Loop counter is maintained to exit the loop after crossing a certain number in most of the cases. If the loop condition fails for the first time itself, no statements are executed. Program control goes to the first statement after the while loop block.
Syntax:
while ( condition ) statements;
Example 1:
int main() { int a=1; while(a <= 10) { printf("%d,", a); a++; //increment or decrement } return 0; } //output: 1,2,3,4,5,6,7,8,9,10
In the above example, we printed all numbers from 1 to 10. If you forget to increment a using a++ statement, loop will never end and the program crashes.
Example 2: While with break statement
int main() { int a=1; while(true) //true makes while loop infinite loop { printf("%d,", a); a++; if(a>10) break; //breaks the while loop } return 0; } //output: 1,2,3,4,5,6,7,8,9,
Here we are depending on break statement to come out of loop instead of LOOP CONDITION.
Example 3: While with continue statement
int main() { int a=0; while(a<=10) //true makes while loop infinite loop { a++; if(a%2 == 0) printf("%d", a); else continue; //it skips printf("*") print("*"); } return 0; } //output: 2*4*6*8*10
Example 4: Nesting of While loop
You can nest a while inside another while loop. You can use break and continue with inner and outer loops if required.
int main() { int a=1, b=1; //outer loop while(a <= 3) { b=a; //nested while loop //inner loop while(b <= 3) { printf("%d,", b); b++; } printf("\n"); a++; } return 9; } //output //1,2,3, //2,3, //3,
2. FOR Loop
A FOR loop works like a WHILE loop except that FOR loop includes both INITIALIZATION section and INCREMENT section along with CONDITION section.
Syntax:
for(INITIALIZATION ; CONDITION ; INCREMENT OR DECREMENT) statements;
Example 1:
int main() { int a; for(a=1; a<=5; a++) printf("%d,", a); return 9; } //output: 1,2,3,4,5
The variable 'a' is initialized with 1 in the for loop. a++ increments the value by 1.
Order of execution of FOR loop
- Initialization part is executed only for the fist time.
- For loop condition is checked. If the condition is satisfied, loop statements are executed.
- After execution of loop statements, program control goes to INCREMENT or DECREMENT part. This completes First Iteration.
- Second Iteration starts with CONDITION check. If the condition is satisfied, loop statements are executed for the second time followed by INCREMENT / DECREMENT.
Note: You can skip all three sections of FOR loop like for( ; ; ){ }. You should add code to break the loop with break; statement. Other wise, it becomes an infinite loop.
Nesting of FOR loop:
You can nest one for loop inside another for loop. You can also nest a while inside for loop, for loop inside do-while loop and so on. Here is an example.
int main() { int a, b; //outer for loop for(a=1; a<=3; a++) { //inner for loop for(b=a; b<=3; b++) { printf("%d,", b); } printf("\n"); } return 9; } //output: // 1,2,3 // 2,3 // 3
You can use break and continue statements inside for loop for better coding and performance.
3. DO WHILE loop
A do-while loop is different from FOR loop and WHILE loop because a do-while loop executes loop statements at least once even if the loop condition fails for the very first time.
Syntax:
do { STATEMENTS; }while( CONDITION ); //SEMICOLON IS REQUIRED.
Example 1:
int main() { int a=1; do { printf("%d,", a); a++; }while( a <=10 ); } //output: //1,2,3,4,5,6,7,8,9,10
Order of execution of DO WHILE loop
- Statements of a DO-WHILE loop is executed at least once. First iteration is over.
- CONDITION is checked. If the condition is satisfied, second iteration starts. If the condition fails, control goes to the next statement after DO-WHILE.
Nesting of DO WHILE loop
You can nest a do-while loop inside another do-while loop or any other loop like FOR or WHILE. To better control a do-while loop, you can use break and continue statements.
Example:
int main() { int a=1, b=1; do { b=a; do { printf("%d,",b); b++; }while(b <=3); print("\n"); a++; }while(a<=3); return 9; } //output //1,2,3, //2,3, //3,
Online Test on C Language Loops
1 | Loops FOR While Do While - Online Test 1 |
2 | Loops FOR While Do While - Online Test 2 |