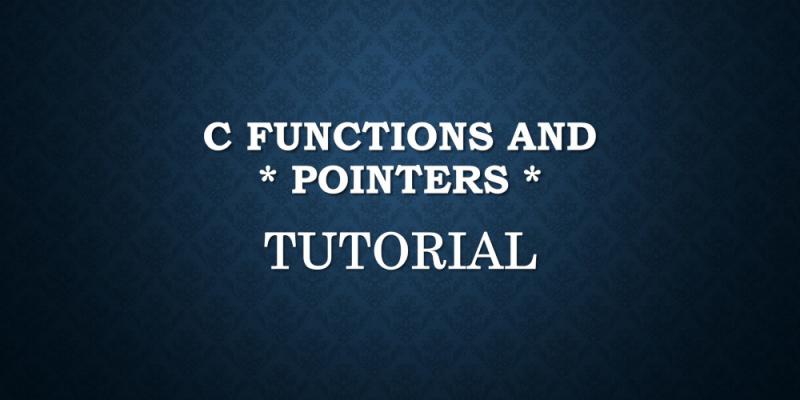
C language is built up of a number of functions. A function is a group of statements or block of statements that servers a specific purpose.
There are two types of functions in a C language.
- Library Functions
- User Defined Functions
1. Library Functions
As the name suggests, Library functions are predefined functions written for specific purposes like printing, scanning input and more. To use library functions, you have to include the header files with the file name extension DOT-H (.h) in your C program using a Preprocessor Directive.
2. User Defined Functions
User defined functions are the functions defined or written by you in your C program. You can define any number of functions in a C program.
Syntax of a Function
RETURN-TYPE FUNCTION_NAME(PARAMERTER1, PARAMETER2,..) { STATEMENTS; // FUNCTION LOGIC return SOME_VALUE; }
Notes about Writing and Working of a Function in C
- A function name should follow the same guidelines of choosing a Variable name
- A function name can not start with a number. It can start with an Underscore ( _ ) symbol.
- A function name can contain Alphabets, numbers and Special character like Underscore.
- Same function name should be repeated again in the same C program.
- Popular 'return' keyword is used most with functions.
- Default Return type of a function is a Garbage Integer value if not specified explicitly.
- A function can return only one value at a time.
- You can put any number of RETURN statements in a c program.
- You need not write a RETURN statement. It is not suggested.
- main() is also a function which is invoked or called or started automatically on execution.
- Any executable C program must contain main() function.
- Other programming languages call functions as methods.
- Arguments are nothing but Parameters a function accepts.
- Arguments received in a function are called FORMAL ARGUMENTS.
- Arguments passed to a function are called ACTUAL ARGUMENTS.
- Variables defined in a C program are called Local Variables. Local variables die after execution of the function.
- Static Storage Class Variables in a function Retain their value among multiple calls.
- Functions accept all types of data i.e int, long, float, double, char and pointers.
- Arguments are values a function accepts from a calling function. Accepting arguments is not mandatory.
- If you call a function SOMEFUNCTION() from main() function, then the main function is called CALLING FUNCTION and SOMEFUNCTION() is called CALLED FUNCTION.
- There is no limit on the number of different functions being defined in a C program.
- A function may be called any number of times.
- Nesting of functions or defining a function inside another function is not allowed.
- Passing or arguments are processed from Right to Left in C Language.
- A function can call itself. This process is called Recursion.
Example 1: Area of Rectangle Function
int area(int,int); int main() { int x=5, y=6; int z = area(x,y); int p = area(2,6); printf("%d, %d", z, p); return 9; } int area(int a, int b) { return (a*b); } //output //30, 12
In the above program, we passes arguments x and y to the function area(). Function area() returned calculated area which is assigned to the variable Z. You can directly call a function with constants without using any variables like area(2,6).
Example 2: Using Library functions
#include<math.h> int main() { float x=1; float a = sin(x); printf("%f", a); return 9; }
We have to INCLUDE the Header file of the Library we want to use. Different functions are included in different libraries. As we are dealing with Trigonometric functions, we have included MATH.H file.
Recursion or Recursive Functions
A function which calls itself is called a Recursive Function and the process is called Recursion.
- To create a recursive function, exit condition must be specified without which it becomes never ending recursion and the program crashes.
- Recursion is slow compared to normal WHILE, FOR and DO WHILE loops.
- Recursion logic by one developer is difficult to understand for other developers.
- Usually, IF or ELSE conditions are used to END recursion.
Example 1:
int main() { int a = 4335; int no_of_digits = func(a); printf("NO=%d", no_of_digits); return 9; } int func(int p) { int k; if(p<10) return 1; else k = 1 + func(p/10); return k; } //output //NO=4 //Analysis //Step1: 1 + func(433) //Step2: 1 + (1 + func(43)) //Step3: 1 + (1 + (1 + func(4))) //Step4: 1 + (1 + (1 + 1) )
Above recursive function takes the input number a P. Using recursion, it counts number of digits present in the given number.
Pointers in Functions
Pointers are used along with functions to pass variables and address of variables from one function to the other.
There are two ways of calling a function with arguments.
- Call By Value
- Call By Reference
1. Call By Value / Pass by Reference
If you pass only variables or constants to a function, it is called Call By Value. Because you do not use any Pointers or Addresses. Advantage of Call By Value is that the original values of passing variables do not change when you make changes in the called function.
Example
void show(int); int main() { int a=10; show(a); //CALL BY VALUE printf("%d", a); } void show(int x) { x = x + 1; //Here it is not a. printf("%d,", x); return; } //output: //11,10 //Value of a is not changed.
2. Call By Reference / Pass by Reference
Call By Reference passes addresses of variables to another function. So whatever changes you make to the variables in the CALLED function reflect in the CALLING function. It has side effects.
Two operators namely ADDRESS OF (&) and VALUE AT ADDRESS (*) operators are used to pass addresses by call by reference.
Example
void show(int*); int main() { int a=10; show(&a); //CALL BY Reference printf("%d", a); } void show(int *x) { *x = *x + 1; //Here it is A only. printf("%d,", *x); return; } //output: //11,11 //Value of a is CHANGED.
&a is the address of the Variable 'a'. *x is the value at the address passed. So you are changing the original copy of data in memory location passed. This is called CALL BY REFERENCE.
Online Test on Functions and Pointers
1 | Functions and Pointers - Online Test 1 |
2 | Functions and Pointers - Online Test 2 |
3 | Functions and Pointers - Online Test 3 |