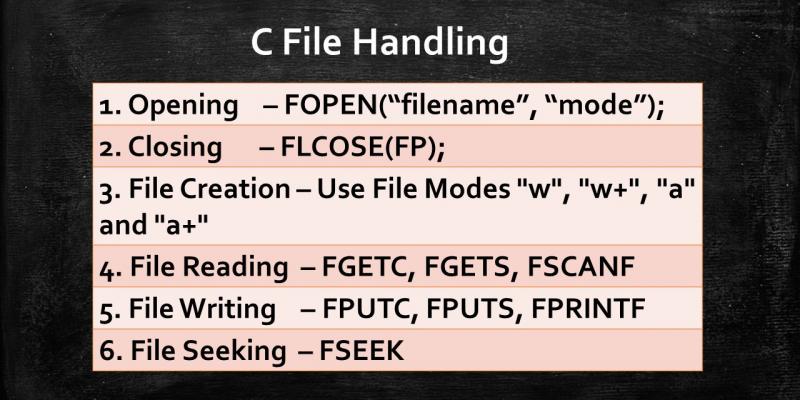
In a computer, information is organized into Files and Directories. C File Handling functions enable us to read files from hard disk and write files to the hard disk.
Note: There is a special data type namely FILE (in capital letters). You should create a Pointer Variable to FILE to handle files on disk. FILE is a struct structure type internally.
C File Handling Functions
Most common file operations are as below.
- File Opening
- File Closing
- File Creation
- File Reading (fscanf, fgetc, fgets & fread)
- File Writing / Editing (fprintf, fputc, fputs & fwrite)
- File Seeking
1. File Opening
To open a file in C language, fopen() function is used. fopen function returns a FILE pointer.
Syntax:
FILE *fp; fp = fopen("filename", "readmode"); if(fp == NULL) { printf("Unable to Read / Write / Create File"); exit(1); } //readmode = "r", "r+", "w", "w+", "a", "a+"
File Read Modes in C language are "r", "r+", "w", "w+", "a" and "a+". R is for Read, W is for Write and A is for Append.
2. File Closing
Every file which is opened should be closed after use to release resources or memory used by it. To close a file in C, fclose() function is used.
Syntax:
FILE *fp; fp = fopen("filename", "readmode"); fclose(fp);
3. File Creation
File can be created simply by changing the File Opening Mode. There are no special functions in C language to create files. File opening modes "w", "w+", "a" and "a+" create a File if it is not existing on the disk.
Syntax:
FILE *fp; fp = fopen("abc.txt", "w+");
4. File Reading
To read a file, it has to be opened first. File Opening modes like "r", "r+", "w+" and "a+" allow reading contents of files. We use EOF as End of File. EOF is a predefined constant that tells END of file.
Example 1: File Reading using FGETC - character by character reading
#include <stdio.h> int main() { char ch; FILE *fp; fp = fopen("abc.txt", "w+"); while( (ch=fgetc(fp)) != EOF ) { printf("%c", ch); } fclose(fp); return 9; }
Example 2: File Reading using FGETS - Line by line reading
#include <stdio.h> int main() { char str[80]; FILE *fp; fp = fopen("abc.txt", "w+"); while( (fgets(str, 79, fp)) != NULL ) { printf("%s", str); } fclose(fp); return 9; }
5. File Writing
To write a file on disk, it has to be opened in suitable File Opening Mode. File opening modes like "w", "w+", "a" and "a+" allow writing of content to files.
Example 1: File Writing using FPUTC - Character by Character
#include <stdio.h> int main() { char str[]= "Tom and Jerry are Good Friends.."; int i=0; FILE *fp; fp = fopen("abc.txt", "a"); while( str[i] != '\0' ) { fputc(str[i], fp); i++; } fclose(fp); return 9; }
Example 2: File Writing using FPUTS - Line by Line or String by String
#include <stdio.h> int main() { char str[]= "Tom and Jerry are Good Friends.."; FILE *fp; fp = fopen("abc.txt", "a"); fputs(str, fp); fclose(fp); return 9; }
Example 3: File Reading and Writing using FSCANF and FPRINTF
#include <stdio.h> int main() { char str[20], str2[20]; int age; FILE *fp; fp = fopen("abc.txt", "a"); printf("Enter name and age: "); scanf("%s%d", str, &age); //Printing to File or Writing to File fprintf(fp, "%s %d", str, age); fclose(fp); //Now reading or Scanning from FILE fp = fopen("abc.txt", "r"); fscanf(fp, "%s %d", str2, &age); printf("Name=%s, Age=%d", str2, age); fclose(fp); return 9; }
6. File Seeking
File seeking is nothing but moving the virtual Cursor in the file so that Read or Write operation can be performed at that cursor position. C language provides a function fseek() to move the cursor in a file.
Note: File Seeking is usually done in Binary File Opening Modes "rb" and "wb".
There are two types of File Opening Modes.
- Binary Mode
- Text Mode
1. Binary Mode
Binary mode saves disk space by consuming only required amount of bytes to store data like text, numbers, video data, audio data and more. Binary mode is difficult to handle. Functions used in Binary mode are fread, fwrite and fseek().
2. Text Mode
Text mode can not save disk space. An integer 24 occupies same space as for storing a big number like 1234. Binary mode takes exactly same amount of bytes required to store in memory. Functions used in Text Mode are fprintf and fscanf. Text mode is easy to handle for humans.
File Opening Modes in C
There are Six file opening modes in C. Two more File opening modes for Binary Data "rb" and "wb" are also present. Letter "b" is added at the end to indicate Binary Mode. Letter "t" is added at the end of mode to indicate Text Mode. So, "rt" represents Read Mode for Text. are also present.
SNO | Mode | Explanation |
---|---|---|
1 | r |
Read: 1. Reading of file 2. File is not created. |
2 | r+ |
Read Plus: 1. Reading 2. Writing 3. File is not created. |
3 | w |
Write: Overwrites (modify) existing. 1. Writing 2. File is created |
4 | w+ |
Write Plus: Overwrites (modify) existing. 1. Writing 2. Reading 3. File is created. |
5 | a |
Append: Appends text at the end. 1. Writing 2. File is created |
6 | a+ |
Append Plus: Appends text at the end. 1. Writing 2. Reading 3. File is created |
Online Test on File Handling Functions
1 | C File Operations Online Test 1 |