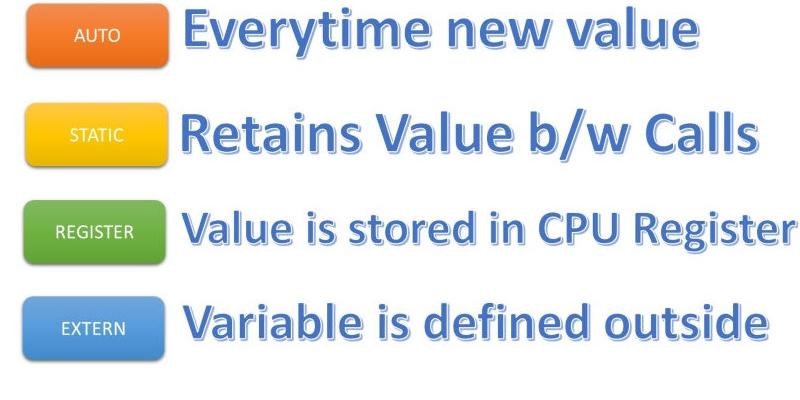
A Storage Class in C language decides the following.
- Where to store a variable in memory
- Default value to be assigned to the variable at the time of creation.
- Scope of a variable within which the variable can store value and outside which the variable is not accessible.
- Life of the variable or the amount of time variable is kept for use.
Each and every variable in C language requires both Data Type and Storage Class. By default, 'auto' storage class is applied to a variable.
C Storage Classes
There are four types of Storage Classes in C language. Keywords used to declare or define C storage classes are auto, register, static and extern.
- Auto Storage Class or Automatic Storage Class
- Register Storage Class
- Static Storage Class
- Extern Storage Class
1. Auto Storage Class
An 'auto' storage variable is stored in memory. This memory is called Stack Memory.
Default value of an auto storage variable is garbage value or random value.
Scope of an auto variable is local to the block where it is defined.
Life of an auto variable is till the execution control stays within the block where it is defined.
In the below example, variable 'a' is declared without 'auto' keyword. But the compiler treats variable like auto variable only. There is no need to explicitly mention 'auto' storage class. It is applied to variables by default.
int main() { int a; //same as auto int a; auto int b; printf("%d %d", a, b); return 0; }
As there is no value assigned to variables, output of the above program is just garbage values. A garbage value is simply a random number of declared type. Here the random value is int type.
int main() { auto int a=20; { int a=10; printf("%d",a); } printf("%d",a); return 0; } //output = 10 20
In the above example, outer a=20 is different from inner a=10. Curly braces define a block in C language. So, a=10 is cleared from memory after the block. So after printing a=10, execution control goes to outer printf to print a=20. After completion of the block, scope and life of an 'auto' variable dies.
In the above infographic image, an auto variable is compared with an AUTO CLOSE BOOK. For each new person, page number of the book is new and random. There is no stored value between function calls.
2. Register Storage Class
A Register storage variable is similar to 'auto' storage variable. Only difference between an auto variable and register variable is the memory location where the variable is stored. Register variables are stored in CPU Registers. These registers are extra memory locations along with RAM memory locations. CPU registers allow fast reading and writing of data. So processor need not ask RAM to read and write data, thus saving time.
Default value of a Register storage variable is garbage value.
Scope of a Register storage variable is local to the block where it is defined.
Life of a Register variable is till the execution control stays within the block where it is defined.
int main() { register int a=1; while(a<10) { printf("%d ", a); a++; } }
Register storage class variables are often used with loop counters or iteration variables to reduce execution time on repetitive works. Also, if a variable is used at many places in a program, then it is better to declare that variable under Register storage class to save time.
The number of CPU registers are always limited. So there is no guarantee that the storage class 'register' ensures storing the variable in CPU registers. You do not get any error. CPU treats the variable like an auto storage class variable and uses stack memory without any warning.
Also note that the size of a CPU register may be 16 bit or 32 bit. If the variable size is more than the size of the register, CPU automatically places the variables in auto storage class. Suppose you want to store a float variable in Register storage class of 16 bit register CPU. Even if you declare explicitly like register float abc=1.2f;, it is treated like an automatic storage class variable.
3. Static Storage Class
A static storage class variable retains its value between function calls. This is called persistence. These static variables die only with the program execution. In case of auto storage variable and register storage variable, value is reset to default after the block or function execution ends. So these auto and register variables can not retain value between function calls.
Static variables are stored in Data Segment Memory instead of Stack Memory.
Default value of a static variable is Zero.
Scope of a static variable is with in the block or function where it is declared.
Life of a static variable exists till the program execution ends.
Example 1:
In the image above with a computer and books on a table, a static variable is like a book with a pen. You can keep pen to maintain page number. Even if you close the book (function or block ends), pen maintains the book number. Next time you open the book (subsequent function or block calls), last page number is maintained.
Example 2:
If you open many windows in a computer and press sleep button, the computer goes to sleep. Once you wake the PC again, all programs are restored with the previous state. Similarly, the value of a static variable persists between function calls. Once you shutdown (program execution ends) the computer, all programs (variables) are removed from memory.:
Example Program:
int main() { display(); display(); } void display() { //invoked only for very first function call static int a=10; static int c; //executed everytime fresh as it is just an assignment c=30; //invoked fresh every time auto int b=20; a++; b++; c++; printf("%d %d %d\n", a, b, c); } //output //11 21 31 //12 21 31
4. Extern Storage Class
Extern or External storage class variables are called global variables. These variables are available to all functions of that C Program. These variables are defined outside of all functions. Variables that are declared inside a function or block are called local variables.
Extern variables are stored in Memory or RAM.
Default value of an extern storage class variable is Zero like static variable.
Scope of an extern variable is global or the entire program.
Life of an extern variable is until the program execution ends.
When to use external variables?
Declare and define an extern variable when you want to make the variable available to all functions of the C program. Also, if you want to make the variable available to functions outside the C program where you defined it.
To use external variables in your program, you need to do two things.
- Use include<> statement to import the C files into your program.
- Declare the extern variable in other C files with an 'extern' keyword.
Example 1:
int stock=5; int main() { display(); stock++; display(); } void display() { printf("Stock=%d\n",stock); } //output //Stock=5; //Stock=6; //variable stock is available to both main() and display() functions.
Example 2:
To use a variable that is not defined until the execution point use 'extern' keyword.
int main() { extern int stock; printf("Stock=%d\n",stock); } extern int stock=5;
In other programming languages, the order of definition of global variables is not important.
Example 3:
You can make your program variable available to included C Files using the extern keyword.
#include<display.c> void showme(); int stock=10; int main() { printf("Stock=%d\n",stock); showme(); } //display.c file extern int stock; void showme() { stock++; printf("Stock=%d", stock); } //output // Stock=10 // Stock=11
In other programming languages, the order of definition of global variables is not important.
Online Tests available:
C Data Types and Storage Classes Online Test 1 |
C Data Types and Storage Classes Online Test 2 |
C Data Types and Storage Classes Online Test 3 |