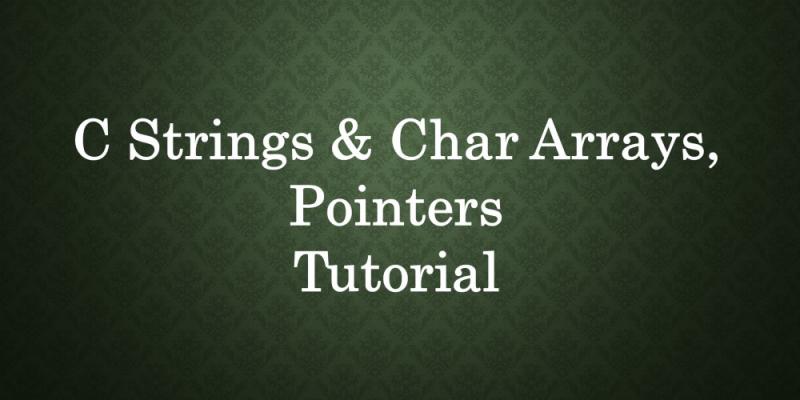
String is just like an array of elements of character (char) data type. Every valid string in c programming language must end with a NULL character or '\0'. ASCII value of a NULL character is 48. Without null character at the end, array is just an array of characters but not STRING.
C Strings and Character Arrays
C language provide 'char' data type to hold Alphabets and Special characters. Size of a single character is 1 Byte.
Let us examine a character array and see how to turn it in to a string. 'string' is not a keyword.
char names[] = {'a','b','c','d'};
we shall turn this character array to string by adding a NULL character at the end. NULL character is also called SLASH ZERO. You can use DOUBLE QUOTES to create a STRING.
char names[] = {'a','b','c','d', '\0'}; //SLASH ZERO (or) char names[] = "abcd";
What is the need to add SLASH ZERO at the end ?
In practice, you will be using String Library Functions to know Length of a String, Copy Strings, Concatenate Strings and more. All these functions want to know after what character string ends in memory. This NULL character tells these string functions about end of a string.
Note: SIZEOF() function always gives the Size of a STRING including '\0'. STELEN() function gives the Length of a string counting number of characters. So the size of a string is one more than the length of that string.
Example 1: Printing a String with %C
int main() { char name[] = {'j','e','r','r','y','\0'}; int i=0; while(name[i] != '\0') { printf("%c", name[i]); i++; } return 9; }
We check each character against a NULL character. It it is not NULL we print it using "%c" format specifier.
Example 2: Printing a String with %S
int main() { char name[] = {'j','e','r','r','y','\0'}; printf("%s", name); return 9; }
"%S" format specifier prints the whole string in one go. It will not print NULL character.
Example 3: Reading a String with %S
int main() { char name[10]; printf("ENTER a name..\n"); scanf("%s", name); printf("Entered=%s", name); return 9; } //INPUT > Enter a name.. //TOMCAT //OUTPUT: TOMCAT
Example 4: Reading a String with Spaces with GETS() function & PUTS() to print
You can not read a string with spaces using %S format specifier. So we use predefined Library function GETS() to read a Line of Text With Spaces.
#include<string.h> int main() { char name[10]; printf("ENTER a name..\n"); gets(name); puts(name); return 9; } //INPUT: > ENTER a name.. //TOM CAT //Entered=TOM CAT
C String Assignments
Just like assigning the value of one variable to another variable, you can not assign one String Variable to another.
Why do you want to assign one variable to another ?
Answer is that you want to copy the value of 1st variable to the second variable.
So use STRCPY() string library function to copy string value and assign to the second variable.
#include<string.h> int main() { char str1[] = "Hello"; char str2[10]; char str3[10]; str2 = str1; //Compiler error; strcpy(str3, str1); //works return 9; }
You can not change the value of whole string to another string constant. You can only change individual characters of a string. If you use a char pointer, you can change the string pointed by it. Let us try changing the value of a string variable in below example.
int main() { char str1[] = "Hello"; str1[1] = 'a'; // You can change individual characters str1 = "AFRICA"; //Error. You can not change char *p; p = "JIMMY"; p = "TOMMY"; //works. You are using pointers. return 8; }
C String Arrays or Multidimensional Arrays
C language allows us to create Array of Strings or String Multidimensional Arrays. What developers use are just 2D String arrays that hold a number of 1D Char Array Strings.
Example 1: String Array - Fixed Length Strings
If you use plain String array without pointers, you can not change the maximum length of strings inside Array of Strings.
int main() { char names[5][10] = { "ARICA", "JAPAN", "SEA", "EGGS", "RIVERS" }; printf("%s", names[0]); printf(" %s, names[1]); names[0] = "PENCIL"; //ERROR. CAN NOT CHANGE. return 9; }
Example 2: String Array - Variable Length Strings
You change maximum length of Strings if you use CHARACTER POINTER to hold address of STRINGS. These STRINGS can be stored anywhere in memory. There is no need to allocate contiguous memory locations to store strings or char arrays in this pointer concept. Also you can reinitialize strings with other strings.
int main() { char *names[5] = { "ARICA", "JAPAN", "SEA", "EGGS", "RIVERS" }; printf("%s", names[0]); name[0] = "PEN AFRICA"; // CAN CHANGE printf(" %s, names[0]); return 9; }
Note: You can not use SCANF() function to scan strings using a CHAR POINTER like *name[0].
C String Library Functions Overview
C strings help us do some most common string operations like COPY, CONCATENATION, UPPERCASE, LOWERCASE and more. Here is a list of STRING function we most use.
SNO | Functions | Description |
---|---|---|
1 | strlen(source) | Returns length of a string without counting NULL character |
2 | strcpy(target, source) | Copies contents of one string to the other. Return type is void. |
3 | strcat(target, source) | Appends new string to the end of SOURCE string. Return type is void. |
4 | strcmp(string1, string2) | Compares string1 with string 2 and returns a number. >0 if greater, 0 for equal and <0 if string1 is less than string2. |
5 | strrevv(string) | Reverses a string and returns. |
6 | strupr(string) | Converts a string to uppercase and returns |
7 | strlwr(string) | Converts a string to lowercase and returns |
8 | toupr(char) | Converts a character to uppercase and returns |
9 | tolwr(char) | Converts a character to lowercase and returns |
10 | strchr(string, char1) | Finds first occurrence of a character in the given string |
11 | strstr(string, str1) | Finds first occurrence of a string str1 in a given string. |
12 | strrchr(string, char1) | Finds last occurrence of a character in a given string. |
13 | strrstr(string, str1) | Finds last occurrence of a string str1 , in a given string. |
C Online Tests on Strings and Char Arrays
1 | Strings, Char Arrays, Pointers - Online Test 1 |
2 | Strings, Char Arrays, Pointers - Online Test 2 |