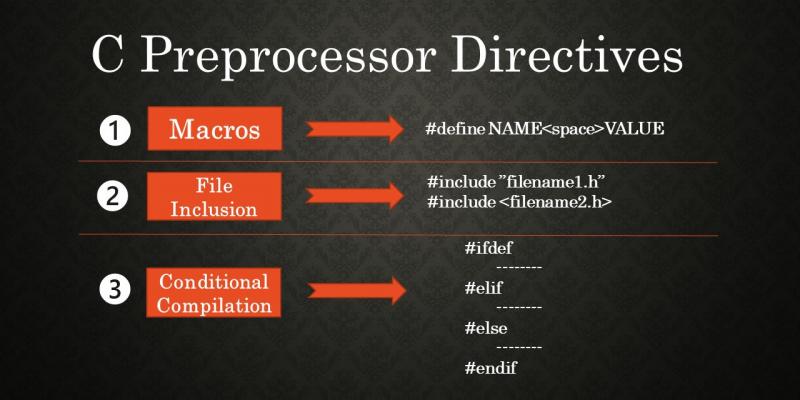
A Preprocessor in a C programming language is a Program that processes source code of a C program, expanding Macro Definitions, File Inclusions and more.
Only through Preprocessor directives, you are able to include library files in your C program.
Preprocessing in C happens before compilation. Part of the Source code is replaced with Preprocessing expressions where ever encountered. So the size of Total code increases before passing to the Compiler.
C Preprocessor Directives
All preprocessor directives start with a Pound Symbol #. There are 3 types of Preprocessor Directives
- Macro Expansion
- File Inclusion
- Conditional Compilation and Run
1. Macro Expansion Preprocessor Directives
Macro is like a function or shortcut that can be used to achieve code reuse. Syntax of defining a macro is as follows.
#define NAME EXPRESSION
Example 1:
#define AREA(x) x*x int main() { int side=20; int area = AREA(side); printf("AREA=%d", area); return 0; } // AREA(side) replaced with side * side
Example 2: Proper use of Parantheses avoids unexpected results.
#define AREA(x) x*x int main() { int side=2; float total = 20 / AREA(side); printf("AREA=%d", area); return 0; } // AREA(side) replaced with side * side // 20 / side * side // (20/side) * side // 10 * 2 // 20 //What you expected ??? // 20 / (2*2) // 5 // Correction: ??? //#define AREA(x) (x*x)
2. File Inclusion Preprocessor Directives
As the name suggests, using a C Precessor directives of File Inclusion type, you are including either Library header files or User defined files. We usually include STDIO.H in our C program to use Printf and Scanf functions.
Note: Preprocessor copies all code of the included file into our C program and sends the combined single copy of code to the compiler.
C editor like TURBO C allows defining list of directories that can be searched for files in a later time. These directories fall into two types.
- Current Directory
- Extra Specified Directories
Syntax:
#include "filename" (or) #include <filename>
There are two ways of including a file using preprocessor directive #include.
1. #include "filename"
This notation searches file in the CURRENT directory and Extra Specified Directory list.
2. #include <filename>
This notation searches files in only CURRENT directory. If the file is not available no error is raised.
Example: Including a header file
#include<math.h> int main() { float a = 10.3f; float b = sin(a); printf("SIN(%f)=%f", a, b); return 9; }
3. Conditional Compilation Preprocessor Directives
Unlike Macro Expansion and File Inclusion preprocessor directives, these Conditional Compilation preprocessor directives are written and used inside our C Program itself.
Condition check is done using IF, ELSE and ELSE IF statements. For Conditional Compilation, directives like #ifdef, #endif, #else, #elif and #ifndef are defined is C language. These help in implementing multiple source codes and version control management in Hardware and Software environments.
The conditional directives check for EXISTENCE of a MACRO and compiles code accordingly. Find examples below for good understanding.
define AREA printf("SUCCESS"); int main() { #ifdef AREA printf("EUROPE"); #else printf("AMAZON"); #endif return 9; }
In the above example, if the MACRO AREA exists, then EUROPE is printed. Otherwise, AMAZON is printed.
#IFNDEF is the reverse of #IFDEF. IFNDEF works if the MACRO does not exist.
#UNDEF Preprocessor Directive
#undef is a special preprocessor directive in C language to Nullify or Remove Definition of existing Macros.
#define AREA printf("SUCCESS"); int main() { #undef AREA #ifdef AREA printf("EUROPE"); #else printf("AMAZON"); #endif return 9; } //OUTPUT //AMAZON //Macro AREA is undefined. So #ifdef fails.
Online Test on C Preprocessor Directives
1 | C PreProcessor Directives - Online Test 1 |
2 | C PreProcessor Directives - Online Test 2 |