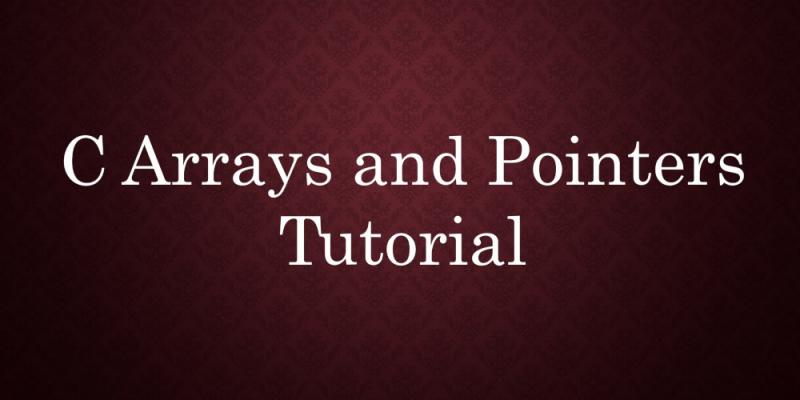
An Array is a group of elements of same data type. C Programming Language allows programmers to deal with maintainability of a C program with tens of hundreds of variables used for a specific purpose.
C Programming Arrays and Pointers
An Array can be thought of as Single Row of Chairs in a Cinema Theater. Each row has a Number. Also, each Chair in that row has a number.
Syntax of Array:
int pincodes[20]; //Array Declaration
Facts about Arrays:
- Every array has a Name which is nothing but the Variable Name.
- Every Array has a Size. It can NOT be be specified at run time or later.
- Every Array has a Data Type.
In the above array declaration, pincodes is an array variable with a capacity of 20 elements. All elements belong to int data type. Even before storing data in the array, memory is reserved in contiguous or continuous memory locations. These contiguous locations can be thought of as Reserved or Booked Chairs in a Cinema Theater.
Array Index Out of Bounds or accessing index more than array size does not produce any error. It simply shows garbage values. Java language shows errors or exceptions in this case.
Array size must be specified in between two Brackets [ ]. It is also called Subscript. A new word INDEX is used to identify the location or order of a particular element in an Array. Eg. pincodes [5] = 10;. Array index always starts with ZERO '0'. So pincodes[5] represents 6th element in the array.
Array elements can be used in all Arithmetic operations.
Array Initialization
By default, array elements are initialized with some default values even if you do not assign any value. Depending on the Storage Class, array elements get some default values. 'auto' and 'register' type array elements hold garbage values by default. 'static' and 'extern' type array elements hold ZERO as default values. By default, 'auto' storage class is applied.
//Declaration and Initialization int pincodes[4] = {1234, 4321, 2345, 5432}; int marks[] = {98, 75, 89, 34, 28, 65}; int buses[3]; //Declaration //Initialization buses[0] = 23; buses[1] = 56; buses[2] = 34;
Array elements can be initialized at the time of Declaration it self. Usually, we assign values to array elements at run time or during execution of program like in buses example above.
Note that we have not specified array size for the variable marks. Because we are initializing the array at the same time. In the case of buses, specifying array size is mandatory as initialization is not done.
Example 1
int main() { int marks[] = {23, 45, 78}; int num = 3, i=0; for(i=num-1; i >= 0; i--) printf("a[%d]=%d, ", i, marks[i]); return 9; } //output //a[2]=78, a[1]=45, a[0]=23,
In the above example, Array "marks" contains 3 elements. Array index starts from 0 and ends with 2.
Example 2: Passing array elements with / without Pointers
We can pass array elements to a function using Pass By Value and Pass By Reference. Pass By Reference is achieved using Pointers.
void display(int, int*); int main() { int x=5, y=8; display(x,y); printf("%d, %d", x, y); return 9; } //a = call by value //*b = call by reference void display(int a, int *b) { *b = *b + 1; printf("%d, %d", a, b); } //OUTPUT //5, 8 //5, 9 //8 becomes after incrementing using pointers
Multidimensional Arrays
An array with only one Subscript is called a Single Dimensional Array or 1D array. An array with more than one dimension is called a Multidimensional Array or nD array. A 2D array is composed of 1 row and 1 column. Size of an array is obtained by multiplying the sizes of individual 1D arrays. For example, students[2][3] represents the element present at 3rd row 4th column.
In a multidimensional array, specifying last subscript or Last Dimension is mandatory during combined declaration and initialization. If you are initializing elements later, mentioning all subscripts or dimension sizes are mandatory.
Example
int main() { //3 Rows each consisting of 2 columns. //WE HAVE NOT MENTIONED ROW SIZE ********** int chairs[][2] = {{22,33},{44,55},{66,88}}; int i=0; j=0; while(i<=(3-1)) { while(j <= (2-1)) { printf("%d,", chairs[i][j]); j++; } printf("\n"); i++; } return 9; } //OUTPUT //22,33, //44,55, //66,88 //Last element of this 2D array = chairs[2][1]
We have used WHILE loop instead of FOR loop in this example. Most of the developers use FOR loop to handle multidimensional arrays.
Using Pointers with Arrays
Arrays are handled internally using pointers. & Operator is called Address Of Operator. &a represents the address of variable 'a'. * (STAR) operator is called VALUE AT ADDRESS operator. So *p represents the value at address if p is a pointer to an address.
To pass an array, we usually pass the Base Address or Address of First Element of an Array.
Example 1: Passing an array to a function
void show(int[]); void show2(int *); int main() { int a[3] = {3,4,5}; show(&a[0]); //We are passing base address show2(&a[0]); return 9; } void show(int k[]) { printf("%d,", k[0]); //print 1st element } void show2(int *p) { p++; //points to 2nd element printf("%d, *p); } //OUTPUT //3,4,
Notes on Array Pointers
We use below code for notes.
int ary[3]; int *p = &ary[0]; int *q = ary; int cats[i]; int kites[i][j]; int bats[a][b][c];
1. ary[5] = *(p+4);
2. q[i] = i[q] = *(q+i) = *(i+q)
3. cats[ i ] = i [ cats] = *(cats+i) = *(i+cats)
4. kites[i][j] = *(*(kites+i) + j)
5. bats[a][b][c] = *(*(*(bats + a) + b) + c)
6. Incrementing an array pointer points to next memory location after skipping memory bytes of the data type.
Online Test on Arrays and Pointers
1 | Arrays, Multidimensional Arrays and Pointers - Online Test 1 |
2 | Arrays, Multidimensional Arrays and Pointers - Online Test 2 |
3 | Arrays, Multidimensional Arrays and Pointers - Online Test 3 |