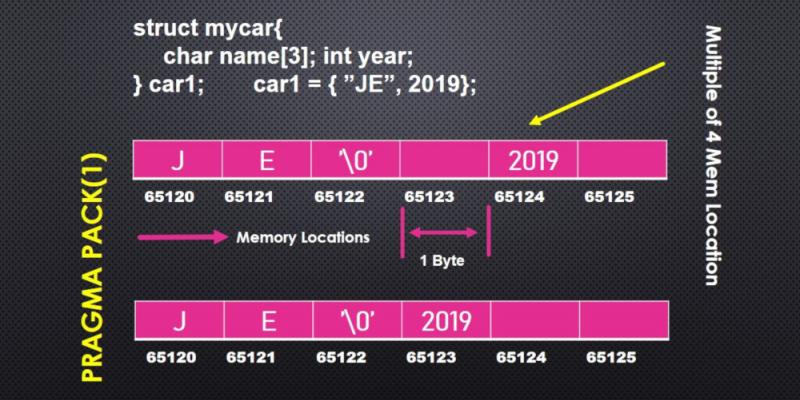
A C language Structure is a user define data type used to combine similar or different data types as one single entity.
An int variable contains only integer data. A float variable contains only real number data. But a structure can be defined to hold multiple type data and refer to the elements separately.
C Structures and Pointers
Keyword used to define a Structure Data Type is 'struct'.
Two operators namely DOT Operator and ARROW Operator are used along with structures to access elements.
Syntax of Structure
struct name { //different data type declarations //int year; }var_1, var_2, *var_3;
Observations
- To reuse structure data type, a name may be given to a structure at the time of declaration itself.
- A structure may contain different types of data like int, float, double, char, arrays and pointers.
- If you do not want to reuse structure data type, you can define some STRUCTURE VARIABLES along with declaration it self without STRUCTURE NAME.
- Structure naming convention follows same rules of defining a variable name. It should not be a keyword.
- You can omit structure NAME declaring just structure variables like var_1 etc.
- You should use DOT (.) operator to access structure elements or members like var_1.year
- You should use ARROW (->) operator to access structure elements if the variable is a POINTER, var_3->year.
- Important observation is that a STRUCTURE definition should END WITH A SEMICOLON (;).
Example 1
#include<string.h> #include<stdio.h> int main() { struct mycar { char col[10], int year; }; struct mycar car1; strcpy(car1.col, "BLACK"); car1.year = 2019; printf("COLOR: %s, Year=%d", car1.col, car1.year); return 0; }
Example 2: Using a Structure Pointer Variable
#include<string.h> #include<stdio.h> int main() { struct mycar { char col[10], int year; }*car5; //ARROW operator makes the difference. strcpy(car5->col, "RED"); car5.year = 2020; printf("COLOR: %s, Year=%d", car5->col, car5->year); return 0; }
C Structure elements in Memory
Declaring a structure does not allocate memory. Only when you create a structure variable, memory is allocated. Size of structure is the combined size of all data types in that structure.
struct mycar { char col[10], int year; }; //Size of structure = 10 bytes + 2 bytes = 12B
In the above example, size of a structure is the combined size of 10 char elements and one int element. It is 12 Bytes in total. Declaring this in a c program does not reserve memory of 12B.
struct mycar { char col[10], int year; }; struct mycar car1; //this line reserves memory of 12B
Creating a structure variable car1 reserves the actual memory required to hold all car1 elements.
Note: To create a STRUCTURE VARIABLE, keyword struct is used again.
Structure elements are aligned in contiguous memory locations like storing arrays. To store the next element type of a structure in memory, memory location starting with a multiple of 4 or multiple of 8 is chosen. So there may be a gap of 3 bytes or 7 bytes if 1B element is stored first.
A preprocessor directive #pragma pack is used to tell the compiler to choose gap between two elements. #pragma pack(1) tells the compiler to store the element in next byte. So the gap of 3 bytes or 7 bytes may be avoided. This is called Packing of Structure Elements.
C Structure Initialization and Copying
Structure elements can be initialized either individually or in one go at a time.
struct mycar { char class; int year; float weight; }car1;
Initializing structure elements individually
car1.class = 'B'; car1.year = 2019; car1.weight = 350.5f; //kg
Initializing all structure elements in one go
struct mycar car2 = {'C', 2020, 275f }; // (OR) struct mycar car3; car3 = car2;
You can copy entire structure variable into another variable by using ASSIGNMENT OPERATOR '='. You can initialize a structure variable using BRACES { } similar to initializing an array. Remember that you need to both declare and initialize elements at the same time using Braces.
You can initialize all elements of a structure variable to Zeros or Null values using { 0 } definition. First element of the structure may be of any data type.
struct mycar car4 = { 0 }; // first element may be of any data type
Default value of a number data type is ZERO and character type data is NULL or '\0'.
Structure Variable Arrays
You can declare Structure variables like any other data type variables. You can use index starting from ZERO to refer to structure array variables.
struct student { char name[10]; int age; }stu[3]; stu[0].age = 25; stu[1].age = 27;
C Nested Structures
You can nest a structure inside another structure. Accessing a nested structure member is achieved using the same DOT (.) operator and ARROW (->) operators. YOu can initialize the elements at the time of declaration of structure variable it self. Only caution is that you should maintain order of elements and their values between Braces or FLOWER BRACKETS.
int main() { struct engine { int capacity; }; struct mycar { struct engine eg; int model; }; struct mycar car1; car1.eg.capacity = 2; //Litre car1.model = 2019; //initializing in one go //maintain the order of elements struct mycar car2 = {3, 2020}; return 9; }
Passing a Structure Variable or Element in C
You can pass a Structure Variable or Element from one function to another function using PASS BY VALUE or PASS BY REFERENCE.
PASS BY VALUE mechanism passes a copy of data without changing original values.
PASS BY REFERENCE mechanism passes Pointer to original data. So, changes made in the Called Method are reflected in the Calling method after call.
Example 1: Passing Structure Variables
We used two structure variables a and b. Student structure is defined outside main() function to make it available to all other functions.
struct student { char name[10]; int rollno; }; void show1(struct student a); void show2(struct student *b); int main() { struct student stu1 = { "TOM", 123}; //PASS BY VALUE show1(stu1); //Just prints //PASS BY REFERENCE show2(&stu1); //Changes values //PASS BY VALUE show1(stu1); //Just prints return 9; } void show1(struct student a) { printf("Name=%s, No=%d\n", a.name, a.rollno); } void show2(struct student *b) { strcpy(b->name, "JIM"); b->rollno = 345; } //OUTPUT //Name=TOM, No=123 //Name=JIM, No=345
Example 2: Passing Structure Elements or Members
You can pass a structure element or member from one function to another function just like passing any other normal data type variable.
struct student { char name[10]; int age; }; void show1(int ag); void show2(int *ag); int main() { struct student stu1 = { "JIMMY", 25 }; //PASS BY VALUE show1(stu1.age); //PASS BY REF show2(&stu1.age); //DISPLAY VALUE AGAIN show1(stu1.age); } void show1(int ag) { printf("AGE=%d\n", ag); } void show2(int *ag) { *ag = 35; } //OUTPUT AGE=25 AGE=35
C Online Test on Structures and Pointers
1 | Structures and Pointers - Online Test 1 |
2 | Structures and Pointers - Online Test 2 |