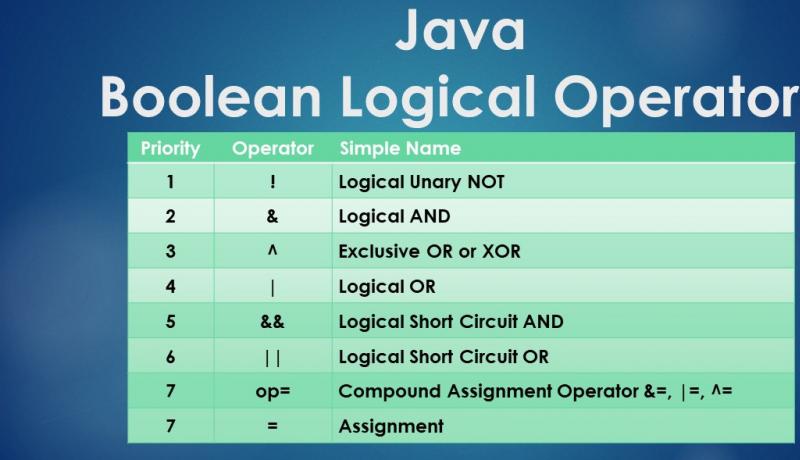
As the name suggests, Java Boolean Logical Operators work with boolean data type values. Some logical operators work with a single Operand while others work with two Operands. There are bitwise logical operators too which we discuss later.
Java Boolean Logical Operators with Priority
The list of Boolean Logical operators is given below. Note that the Logical Unary NOT (!) has got the highest priority among all other logical operators. The assignment operator has the least priority.
Note 1: Except for Logical NOT(!), all other logical operators have less priority than Arithmetic and Relational operators.
Priority | Operator | Simple Name |
---|---|---|
1 | ! | Logical Unary NOT |
2 | & | Logical AND |
3 | ^ | Exclusive OR or XOR |
4 | | | Logical OR |
5 | && | Logical Short Circuit AND |
6 | || | Logical Short Circuit OR |
7 | op= |
Compound Assignment Operator &=, |=, ^= |
7 | = | Assignment |
Note 2: Generally, software programmers use only Short Circuit Logical operators compared to normal logical operators for good performance and to differentiate bitwise AND and OR from Logical AND and OR operators. If you use AND (&) operator, it generally means you are using it in the context of bitwise operations.
Example with Precedence or Priority Explained
In the below code example, Relational operator (>) takes higher priority. Short Circuit AND (&&) has got the least priority.
class OperatorPrecedence { public static void main(String args[]) { int a=5; int b=9; boolean c = a > 4 && true & b !=9; // Priority order: >, !=, &, && // (a>4) && true & b!=9 // true && true & b==9 // true && true & false // true && false //false System.out.println(c); } } //OUTPUT: false
1. Logical Unary NOT (!) Operator
Logical Unary NOT operator requires just One Operand. It simply turns true to false and false to true. Its symbol is an Exclamation.
Expression | Result |
---|---|
false | true |
true | false |
Example Usage:
boolean show = true; show = !show; if(!show) { System.out.println("SHOW = false"); }
2. Logical AND (&) Operator
Logical AND operator gives an output of true only if both operands are true. Logical AND operator checks the value of both operands or expressions before giving output. Even if the first expression is false, it goes for evaluating the second expression. This is a time waste thing and a drawback. Its symbol is an Ampersand.
expression1 | expression2 | Result |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
Example Usage:
int k=5, p=9; c = (k<4)&(p>5); //p>5 is evaluated. Time waste. if(c) { System.out.println("Logical AND"); }
3. Logical Short Circuit AND (&&) Operator
Logical Short Circuit AND gives an output of true only if both Operands or Expressions are true. If the first expression is false, Short Circuit AND (&&) avoids evaluating the second expression. So it is faster than normal Logical AND (&) operator. Its symbol is Two Ampersands (&&).
expression1 | expression2 | Result |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
Example Usage:
int k=5, p=9; c = (k<4)&&(p>5); //p>5 is not evaluated. if(!c) { System.out.println("Short Circuit Logical AND"); }
4. Logical OR (|) Operator
The logical OR (|) operator gives an output of true if one of the operands is true. So a combination of true and false always gives true as the output. Logical OR evaluates the second expression or operand even if the first expression is true. So it is slow compared to Short Circuit Logical Operator (||). Its symbol is a PIPE.
expression1 | expression2 | Result |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
Example Usage:
int k=5, p=9; c = (k>3)|(p>5); //p>5 is evaluated. Time waste. if(c) { System.out.println("Logical OR"); }
5. Logical Short Circuit OR (||) Operator
The Logical Short Circuit OR (||) operator gives an output of true if one of the expressions or operands is true. It gives an output of true if the first expression is true without evaluating the second expression. So it is fast. Only if the first expression is false, the second expression is evaluated or executed by Short Circuit OR operator.
expression1 | expression2 | Result |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
Its symbol is Two Pipes (||).
Example Usage:
int k=5, p=9; c = (k>3)||(p>5); //p>5 is not evaluated. if(c) { System.out.println("Short Circuit Logical OR"); }
6. Logical Exclusive OR (^) Operator
The Logical Exclusive OR (^) operator gives an output of true if both operands are different. It gives an output of false if both the operands or expressions are the same. Its symbol is a CARAT.
expression1 | expression2 | Result |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | false |
Example Usage:
int k=5, p=9; c = (k>3)^(p>9); if(c) { System.out.println("Logical XOR"); }
Java Compound Logical Assignment Operators
There are only three Compound Logical Assignment operators in Java, AND, OR and Exclusive OR. Simply put an Equal To (=) symbol after the Logical AND, OR or XOR operators to turn it into a Compound Assignment operator.
Note: The compound assignment is not possible with Short Circuit AND (&&), Short Circuit OR (||) and Unary NOT(!) operators.
1. Compound Assignment AND (&=) Operator
Here "expression" should be boolean variable or constant. "var" is a variable.
Syntax:
var &= expression var = var & expression eg. a &= false a = a & false
2. Compound Assignment OR(|=) Operator
Here "expression" should be boolean variable or constant. "var" is a variable.
Syntax:
var |= expression var = var | expression eg. a |= true a = a | true
3. Compound Assignment Exclusive OR (^=) Operator
Here "expression" should be boolean variable or constant. "var" is a variable.
Syntax:
var ^= expression var = var ^ expression eg. a ^= true a = a ^ true
Example: Logical Compound Assignment Operators
class CompoundAssignmentOperators { public static void main(String args[]) { boolean a = true; boolean b= false; boolean c = true; boolean d = false; a &= false; //a = a & false = false b |= true; //b = b | true = true c ^= false; //c = c^false = true d &&= false; //Error. Not an Operator d ||= false; //Error } }
In the next chapters, we shall discuss Bitwise Operators in Java with examples.