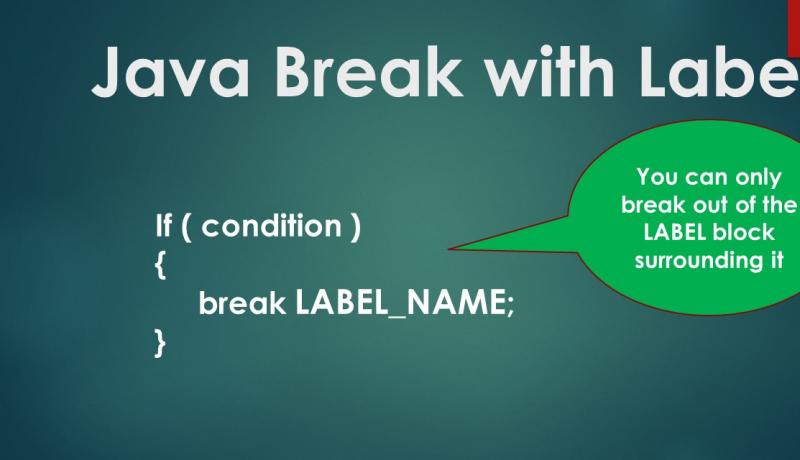
A BREAK statement in Java causes the program control to exit the block or loop immediately.
Java Label
A Java Label is any valid Identifier or name with a COLON after it.
Rules to define a Label
- A Label name must start with either Alphabet, Underscore(_) or a Dollar ($) symbol. It may contain numbers.
- A Label must be followed by a Loop or a Block.
- Defining a Label like a Goto statement produces a compiler error
There are two types of Break statements.
- Break without label
- Break with label
1. Java Break without Label Statement
Using a Break statement without specifying any Label causes the loop to close and exit immediately. It works only with Loops.
You can use the Break statement without a Label with any loop like FOR, Enhanced FOR, WHILE and DO WHILE loop. You can not break more than one loop using just a BREAK without Label.
2. Java Break with Label Statement or GOTO Statement
C language supported goto statements for the convenience of developers or programmers. Java supports GOTO statement in the form of BREAK with Label or CONTINUE with LABEL statements. That means you can not use the keyword "goto" as of now. It is just a reserved word.
Break with a Label works with both Code BLOCKS and Loops.
Break with a Label Block
You can define a Block in Java program using an Opening Brace ( { )and a Closed Brace ( } ). Just add a name to that block with a COLON symbol. SWITCH block is the best example of using a BREAK with a BLOCK statement. After the BREAK statement is encountered, program control goes out of that named block or labelled block.
Example:
class LabelBlock { public static void main(String[] args) { int a=10; MYLABEL: { System.out.println("Entered Label"); if(a>5) break MYLABEL; System.out.println("NOT REACHABLE"); } System.out.println("AFTER LABEL BLOCK"); } } //OUTPUT //Entered Label //AFTER LABEL BLOCK
Break with a Label using Loops
You can define a LABEL with a Loop BLOCK like WHILE loop block, FOR loop block, Do WHILE loop block or an Enhanced FOR loop Block. You can escape any number of loops with a Break inside a Labelled Block. Nesting is not just 2 levels in the real programming world.
Rules to use Break with a Labelled Loop
- Break statement should be part of the labelled loop.
- You can not break out of a labelled loop which does not enclose the break statement.
Example: Break a Nested Loop or Inner Loop
class BreakNesetedLoop { public static void main(String[] args) { outer: for(int i=0; i<5; i++) { for(int j=0; j<5; j++) { System.out.print(j + ","); if(j==3) break outer; } } System.out.println("\nOUTSIDE of LABEL"); } } //OUTPUT //0,1,2,3, //OUTSIDE of LABEL
This is how a BREAK with LABEL works in Java.
It is time to Share this Last Minute Java Tutorial with your friends and colleagues to encourage authors.