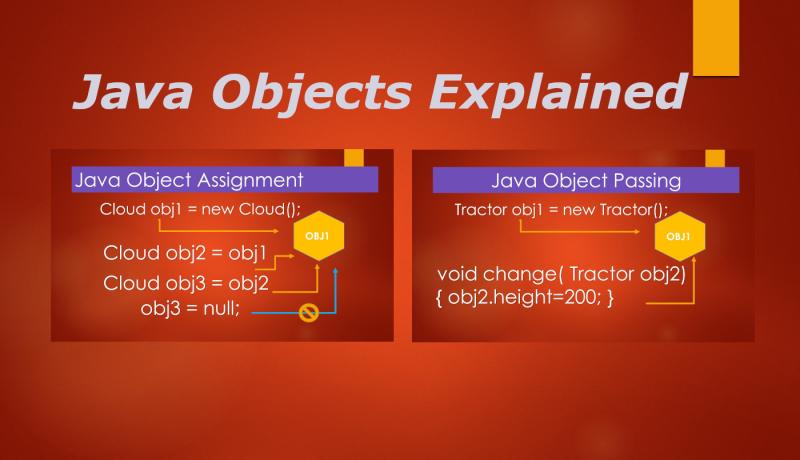
In Java, we create Classes. We create objects of the Classes. Let us know if it is possible to assign a Java Object from one variable to another variable by value or reference in this Last Minute Java Tutorial. We also try to know whether it is possible to pass Java Objects to another method by Value or Reference in this tutorial.
You may also read about Introduction to Java Class Structure.
Java Object Assignment by Value or Reference Explained
In Java, objects are always passed by Reference. Reference is nothing but the starting address of a memory location where the actual object lies. You can create any number of references to just One Object. Let us go by an example.
We create a Class "Cloud" with a single Property called "color". It is a String object. We create 3 objects of the Cloud type.
public Class Cloud { String color; public static void main(String[] args) { Cloud obj1 = new Cloud(); obj1.color = "RED"; Cloud obj2, obj3; obj2 = obj1; //Assign obj1 to obj2 obj3 = obj2; //Assign obj2 to obj3 System.out.println("OBJ2= " + obj2.color + ", OBJ3= " + obj3.color); obj2=null; obj3=null; System.out.print("Color= " + obj1.color); } } //OUTPUT //OBJ2= RED, OBJ3= RED //Color= RED
Notice that all three references obj1, obj2 and obj3 are pointing to the same Cloud object. Thus the above example outputs the same color-property value "RED". Later, we made the references obj2 and obj3 to point to the null location. Still the original object pointed or referenced by obj1 holds the value. So the final PRINT statement outputs "RED" as usual.
Note: Reference is also called a Variable whether Primitive or Object.
This concludes that a Java object assignment happens by Reference. But the actual value of memory location pointed by References are copied by Value only. So we are actually copying memory locations from one Reference variable to another Reference variable by Value. Interviewers ask this famous Java question just to test your knowledge. If someone asks in C language point of view, say that it is by Reference. If they ask in Java point of view, say that it is by Value.
Java Object Passing by Value or Reference to a Method Explained
You already learnt that Object assignment in Java happens by Reference. Let us try to pass Objects to another method as a parameter or argument. We try to know whether Objects are passed by Value or Reference to another Method using the below example.
class Tractor { int height; void change(Tractor obj) { obj.height = 200; } public static void main(String[] args) { Tractor obj1 = new Tractor(); obj1.height = 100; System.out.println("Before Height= " + obj1.height); obj1.change(obj1); System.out.println("After Height= " + obj1.height); } } //OUTPUT //Before Height= 100 //After Height= 200;
In the above example, we have created an object "obj1" of type Tractor. We assigned value of 100 to the property "height". We printed the value before passing the object to another method. Now, we passed the object to another method called "change" from the calling-method "main". We modified the height property to 200 in the called-method. Now, we tried to print the value of the property in the Calling method. It shows that the Object is modified in the called-method.
Similarly, we can pass Java objects to the Constructor using Pass by Reference or simply Reference.
This concludes that Java Objects are passed to methods by Reference. Here also, we pass the memory location pointed by one Reference variable to another Reference variable of a Called method as a Value only. So, you are actually passing Values (memory locations represented by integer or long data type). But the actual passed-object can only be referenced by a Reference variable. Interviewers take advantage of this tricky logic to confuse you. If someone asks in C language point of view, say that it is by Reference. If they ask in Java point of view, say that it is by Value.
Let us know more about Java Classes with different Constructors and Methods in coming chapters.
Share this Last Minute Java Tutorial explaining Java Object Assignment using Reference and Java Object Passing with Pass by Reference with your friends and colleagues to encourage authors.