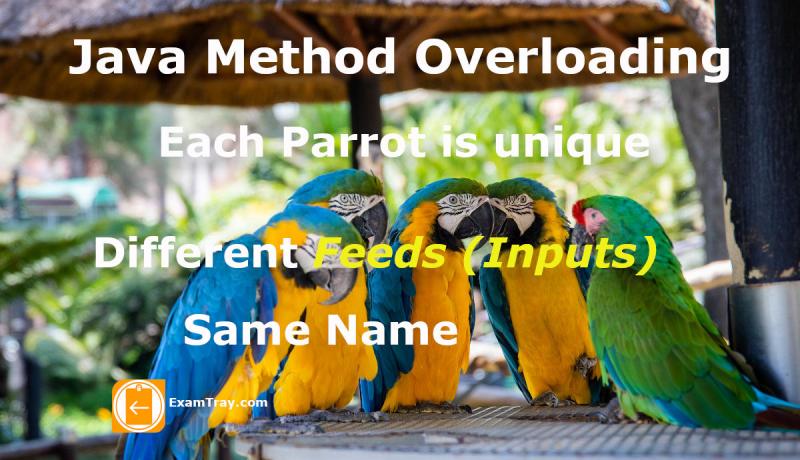
Java Method Overloading is nothing but the ability to write more than one Method with the same name in a Class with varying Return-Types or Parameters. In other words, you should maintain unique method signatures for all methods with the same name to successfully Overload. Let us know more about Method Overloading in this Last Minute Java Tutorial.
You can read more about Constructor Overloading in Java for better understanding.
Java Method Overloading Explained
Java methods contain reusable pieces of code that can be called any number of times. Java methods can receive arguments and return values. Parameters or Return values can be primitives or objects.
We simply maintain unique method signatures while maintaining the same method name to Overload a Java Method.
Rules to Overload a method:
- If Parameter-list is the same, Return-type can be Super-Class or Sub-Class type.
- If the Parameter list is different, the return value can be either the same or different.
- If the return-type is same, the Parameter or Argument list should be distinct or different.
Let us check the below example 1.
public class Bank { int amount=0; void addAmount(int amt) { amount = amount + amt; } void addAmount(String strAmt) { amount = amount + Integer.parseInt(strAmt); } int addAmount(double amt) { amount = amount + (int)amt; return amount; } }
In the above example, we have Overloaded a Java method namely "addAmount". We have used "void" and "int" as return types for all the methods with the same name "addAmount". We differentiated the overloaded methods by varying the data-type of the received parameter or argument. We have shown variation by using int, String and double types. The String is an Object-type parameter.
Let us check the below example 2.
class School { int message(String msg) //error overloading { System.out.println("Message: " + msg); return 0; //some value } void message(String msg) //eror overloading { System.out.println("Message: " + msg); } }
Above example throws a Java error like "Duplicate method message(String) in type School". Because the two methods have the same parameter list and different return-types. Return types should have been Subclass and Superclass types to successfully Overload methods.
We shall discuss Java Method Overriding which involve Inheritance concepts in the coming chapters.
Share this Last Minute Java tutorial on Java Method Overloading and Rules with your friends and colleagues to encourage authors.