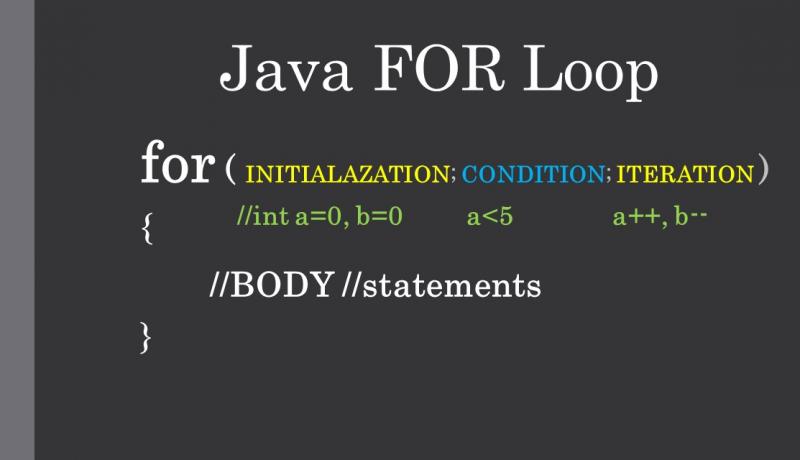
Java language provides another good looping control statement called FOR loop. A FOR loop is superior to WHILE loop and DO WHILE loop in terms of flexibility in maintaining loop variables.
Java FOR Loop Explained
There are two types of FOR loops.
- FOR loop
- FOR EACH or Advanced FOR or Enhanced FOR loop
In this chapter, we shall see usage examples of the Traditional FOR loop.
Syntax:
for(initialization; condition ; iteration) { //STATEMENTS //BODY }
Example:
for(int i=1; i<=5; i++) System.out.print(i + ","); // //OUTPUT //1,2,3,4,5,
There are three parts in a FOR loop.
- Initialization
- Condition
- Iteration (Increment or Decrement)
1. Initialization Part - FOR Loop
You can declare and initialize any type of variables and any number of variables under INITIALIZATION part of a FOR loop. If you declare a variable, you must initialize it with some value. There is no default value assigned.
Rules for declaring and Initializing Variables
- Multiple variables are separated with Commas(,).
- All variables should be of the same data type.
- Any type of variable can be declared and initialized.
- After this initialization part, a Semicolon (;) is mandatory which separates CONDITION part of FOR loop.
Example:
for(int i=1, j=0, k=4; (i<=5)&&(j<=5); i++) System.out.print(i + ","); // //OUTPUT //1,2,3,4,5, //j and k are not used.
2. Condition Part - FOR Loop
The CONDITION can be an expression that evaluates to a boolean constant. Usually, this consists of Relational or Conditional Operators along with Logical Operators to create a complex CONDITION.
3. Iteration Part - FOR Loop
Iteration Part consists of Increment and Decrement Operations on the Loop and other Variables.
FOR Loop with Break Statement
You can use a BREAK statement inside a FOR loop to break out of the loop. Program control exits the loop after encountering a BREAK statement. Usually, an IF Statement or Block surrounds the Break statement. Without the IF condition, the loop becomes Infinite or never-ending.
Example:
for(int i=1; ; i++) { if(i>5) break; System.out.print(i + ","); } //OUTPUT //1,2,3,4,5
FOR loop with Continue Statement
You can use a CONTINUE statement inside a FOR loop to force the program execution control go to the beginning of the loop. Loop condition is checked once again before executing the loop body from the beginning to the end. A CONTINUE statement usually follows an IF statement.
Example:
for(int i=1; i<=10; i++) { if(i%2!=0) continue; System.out.print(i + ","); } //OUTPUT //2,4,6,8,10,
Nested FOR Loop
You can nest a FOR loop inside another FOR loop, WHILE loop and DO WHILE loops. Nested loops are useful to access Multidimensional Arrays.
Example:
for(int i=1; i<=5; i++) { for(int j=i; j>0; j--) { System.out.print(j+" "); } System.out.println(""); } //OUTPUT //1 //2 1 //3 2 1 //4 3 2 1 //5 4 3 2 1
This is how a Java FOR loop works.
Share this tutorial with your friends and colleagues to encourage authors.