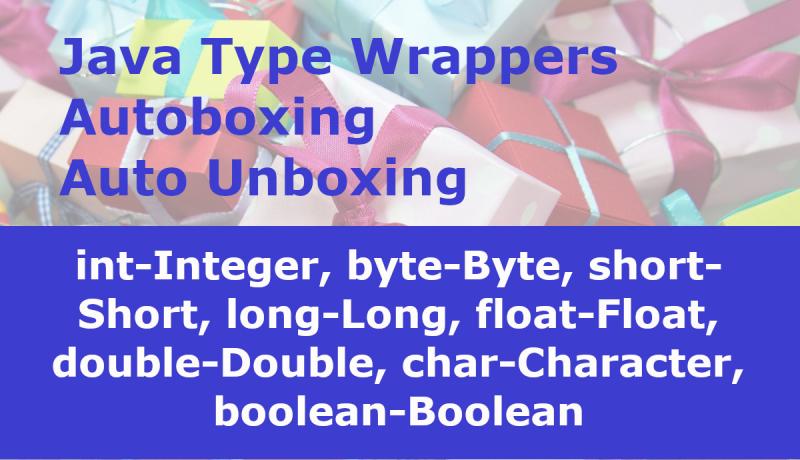
Java programming language has built-in support for Primitive Data types like byte, short, int, long, float, double, char and boolean. Java Type Wrappers are nothing but object version of primitive data types with the class names like Byte, Short, Integer, Long, Float, Double, Character and Boolean. As these are objects, they have some useful methods attached for readymade use. Java also does Autoboxing and Auto Unboxing for you. Let us learn more in this Last Minute Java Tutorial.
Java Generics work only with Objects. So, these Type-Wrappers or Wrapper Types play an important role in working with Primitive type data.
Java Primitive Type Wrappers Explained
Java Wrapper Objects are not efficient compared to their Primitive counterparts. So, the developers should use these wrapper types only when needed. That is the reason why Java still continues supporting the primitive data types. Numeric type wrappers are Byte, Short, Integer, Short, Long, Float and Double.
All Java Type Wrapper classes come with a method valueOf() which accepts either a primitive number or String number and returns the corresponding Wrapper Type Object. For example, Integer.valueOf(int) or Integer.valueOf(String) returns an Integer object.
Java 9 deprecated all Type Wrapper constructors that accept a primitive value and return a Wrapper class Object.
Let us go throw each Wrapper object with some example program.
Byte
Byte is a Type Wrapper class for the primitive byte data type. Its useful methods are byteValue(), parseByte(String), valueOf(byte) and valueOf(String).
Example:
byte b = 9; Byte b1 = new Byte(b); //deprecated Byte b2 = Byte.valueOf(b); //recommended by Java 9 byte b3 = b2.byteValue(); byte b4 = Byte.parseByte("8");
Short
Short is a Type Wrapper class for the primitive short data type. Its useful methods are shortValue(), parseShort(String), valueOf(short) and valueOf(String).
Example:
short s = 10; Short s1 = new Short(s); //deprecated Short s2 = Short.valueOf(s); //recommended by Java 9 short s3 = s1.shortValue(); short s4 = Short.parseShort("10");
Integer
An Integer is a Type Wrapper class for the primitive int data type. Its useful methods are intValue(), parseInt(String), valueOf(int) and valueOf(String).
Example:
Integer i1 = new Integer(11); //deprecated Integer i2 = Integer.valueOf(11); //recommended by Java 9 int i3 = i1.intValue(); int i4 = Integer.parseInt("11");
Long
A Long is a Type Wrapper class for the primitive long data type. Its useful methods are longValue(), parseLong(String), valueOf(long) and valueOf(String).
Example:
Long lon1 = new Long(12); //deprecated Long lon2 = Long.valueOf(12); //recommended by Java 9 long lon3 = lon1.longValue(); long lon4 = Long.parseLong("12");
Float
A Float is a Type Wrapper class for the primitive float data type. Its useful methods are floatValue(), parseFloat(String), valueOf(float) and valueOf(String)
Float f1 = new Float(15.1f); //deprecated Float f2 = Float.valueOf(15.1f); //recommended by Java 9 float f3 = f1.floatValue(); float f4 = Float.parseFloat("15.1f");
Double
A Double is a Type Wrapper class for the primitive double data type. Its useful methods are doubleValue(), parseDouble(String), valueOf(double) and valueOf(String).
Double d1 = new Double(16.5); //deprecated Double d2 = Double.valueOf(16.5); //recommended by Java 9 double d3 = d1.doubleValue(); double d4 = Double.parseDouble("16.5");
Character
A Character is a Type Wrapper class for the primitive char data type. Its useful methods are charValue(), valueOf(char), toLowerCase(char), toUpperCase(char), isLetter(char), isDigit(char) and getNumericValue(char).
Example:
Character ch1 = new Character('A'); //deprecated Character ch2 = Character.valueOf('A'); //recommended by Java 9 char ch3 = ch1.charValue(); char ch4 = Character.toLowerCase('B'); boolean bool = Character.isDigit(ch3);
Boolean
A Boolean is a Type Wrapper class for the primitive boolean data type. Its useful methods are booleanValue(), parseBoolean(String), valueOf(boolean) and valueOf(String).
Example:
Boolean bool1 = new Boolean(true); //deprecated Boolean bool2 = Boolean.valueOf(true); //recommended by Java 9 boolean bool3 = bool1.booleanValue(); boolean bool4 = Boolean.parseBoolean("false");
Java AutoBoxing and Auto Unboxing Explained
Java Autoboxing is nothing but the automatic conversion of a primitive data type value to its equivalent Type Wrapper Class object version by the compiler. For example, an int value is converted to Integer object automatically and implicitly by the compiler. You do not need to worry. So, the boxing process returns an object.
Wrapping and unwrapping of primitives and wrapper-objects work automatically with all arithmetic, comparison, bitwise and logical expressions and operators. You can use these Wrapper Objects in all Java loops, IF-ELSE statements, Switch-Statements and Ternary-Operator expressions as if they are primitives without Type-casting and conversion manually.
Examples:
Byte b = (byte)10; Short s = 11; Integer i = 12; Long lon = 15L; lon--; //increment operation also Float f = 10.5f; Double d = 15.3; d++; //decrement operation also Character ch = 'C'; Boolean bool = false;
Java Auto Unboxing is nothing but the automatic conversion of a Type Wrapper Class object to its equivalent Primitive data type value by the compiler. So, an unboxing process returns a primitive type value, for example, unboxing of Float returns a float.
Examples:
Short s2 = 25; short s3= s2; Integer i2 = 27; int i3 = i2; Long lon2 = 29L; long lon3 = lon2; Float f2 = 30.1f; float f3 = f2; Double d2 = 33.33; double d3 = d2; Character ch2 = 'D'; char ch3 = ch2; Boolean bool2 = false; boolean bool3 = bool2;
You have seen enough examples that convert objects of Type Wrappers into primitive types and vice versa. These autoboxing and Auto-unboxing operations save a lot of code and minimise errors.
Share this Last Minute Java tutorial on Type Wrappers, Autoboxing and Auto-Unboxing with your friends and colleagues to encourage authors.