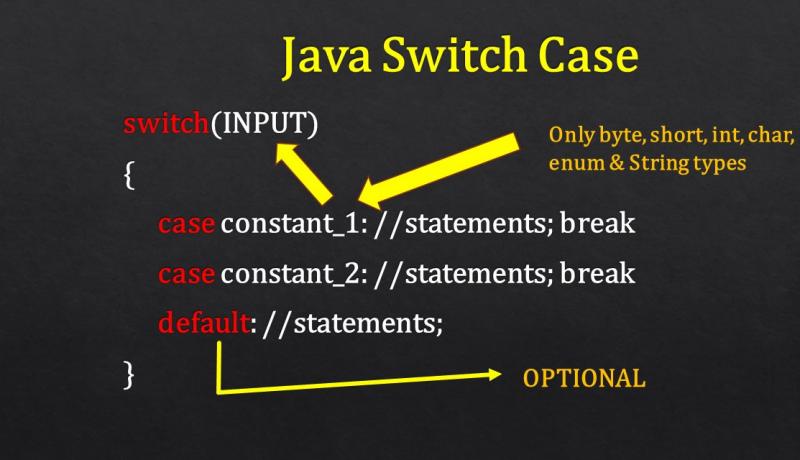
Java language provides an alternative to the IF ELSE-IF ladder in the form of SWITCH CASE.
Java Switch case uses the following predefined keywords.
- switch
- case
- default
- break
Java Switch Case Control Statement
A SWITCH case allows a programmer to define a set of conditions in the form of constants. So, it is also called a multi-condition if-else-if ladder.
SWITCH case has the following parts.
- SWITCH INPUT
- CASE constants
- CASE statements
Syntax:
switch(input) { case constant1: //statements case constant2: //statements default: //statements }
SWITCH case INPUT is compared against each case constant for matching or equality. If the condition is satisfied, the corresponding CASE Statements are executed.
Example:
int a=5; switch(a) { case 3: System.out.println("THREE"); break; case 5: System.out.println("FIVE"); break; default: System.out.println("UNKNOWN"); } //OUTPUT //FIVE
Switch Case Fall Through (Range Defining)
If there is no break statement after SWITCH CASE statements, program control goes to the next CASE. The statements under the next CASE are also executed. Without checking the case constants, all the statements in all other cases, are executed down the ladder. This behaviour is not intended. If there is a break statement, program control exits the SWITCH case after executing the statements.
In the below example, you can see the range 1-4 and 5-8 defined by the SWITCH statement. Observe that break statement is present only at the end of the Range we need.
Example:
class SwitchCaseRange { public static void main(String args[]) { int a=2; switch(a) { case 1: ; case 2: ; case 3: ; case 4: System.out.print("Range: 1-4"); break; case 5: ; case 6: ; case 7: ; case 8: System.out.print("Range: 5-8"); break; default: System.out.println("UNKNOWN"); } } } //OUTPUT //Range: 1-4
Switch Case INPUT Types
A Switch case does not work on all data type values. boolean, float, double and long data type constants are not allowed. Starting with JDK 7 or JAVASE 7, String type constants are supported.
The switch case works with the following Java literals or constants.
- byte
- short
- char
- int
- enum
- String (As of JDK 7)
Example: Switch with Strings
class SwitchStrings { public static void main(String args[]) { String name = "JAVA"; switch(name) { case "java": System.out.println("java");break; case "JAVA": System.out.println("JAVA");break; default: System.out.println("Unknown"); } } } //OUTPUT //JAVA
Example: Switch with enums
class SwitchEnums { static enum CAR {JEEP, KIA, JAGUAR} public static void main(String[] args) { CAR car1 = CAR.JEEP; switch(car1) { case KIA: System.out.println("KIA");break; case JEEP: System.out.println("JEEP");break; case JAGUAR: System.out.println("JAGUAR");break; default: System.out.println("UNKNOWN"); } } } //OUTPUT //JEEP
Switch Case Errors
Most possible switch case error is about the Case Constant used. One such error is "case expressions must be constant expressions". Simply add the "final" keyword before the variables used as Case Constants.
Example:
class SwitchErrors { public static void main(String[] args) { final String name = "JAVA"; switch(name) { case name: System.out.println("java");break; } } } //OUTPUT //java
Switch Case Constant Rules
- Variables are not allowed as constants
- "final" type variables are allowed
- Duplicate case constants are not allowed
- "break" statement is optional
- "default" statement is optional
- Only JDK 7 and above supports String constants
- Conditional statements are not allowed.
- Case constants are checked only for equality condition against Switch INPUT
- More than and Less than conditions cannot be checked.
- Nesting of Switch cases is allowed to any depth.
- Allowed case constant types are byte, short, char, int, enum and String
Switch Case Vs IF-ELSE-IF Ladder Performance
A switch case has good performance when compared to an IF-ELSE-IF ladder. After the compilation of Java program, a JUMP Table is created by the compiler. This Jump table helps to control the execution path at Runtime without checking again. In the case of IF-ELSE, all checkings are done at Runtime. So IF-ELSE is a bit slow.
Nesting of Switch
You can happily nest one switch statement inside another switch statement. Nested Switch is usually kept at Case Statements section followed by a break statement to avoid fall through. We have used multiple cases inside the outer switch and inner switch.
Example:
class NestedSwitch { public static void main(String[] args) { char type = "B"; int model = 4; switch(type) { case 'B': System.out.println("B"); switch(model) { case 4: System.out.println("FOUR"); } break; case 'C': System.out.println("C"); break; default: System.out.println("UNKNOWN"); } } } //OUTPUT //B //FOUR
This is how a Switch works in Java.
Share this tutorial with your friends and colleagues to encourage authors.