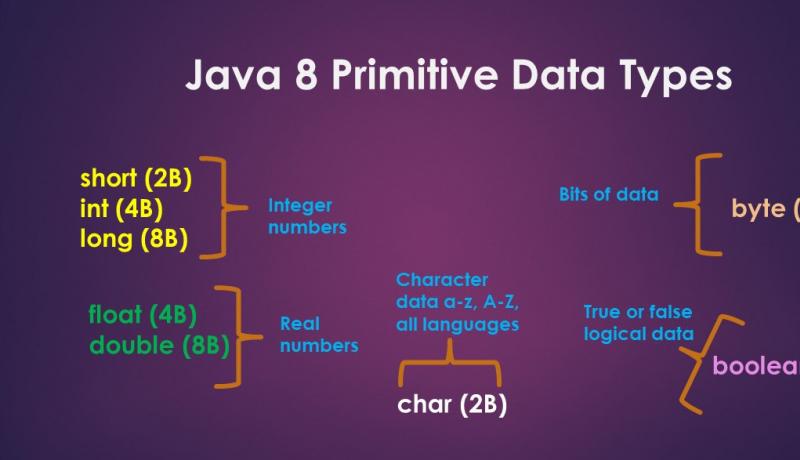
A Data type refers to the type of data we use in our Java program. There are different types of data namely integer numbers, real numbers or fractions and character data. These are non object data types.
Java Language Primitive Data Types
Java does not offer unsigned data types like C language. It provides only a signed version of integer data types. Sizes of data types are machine independent in Java. So irrespective of hardware and operating system implementations, sizes of Java data types remain the same to provide consistency and portability.
Note: These primitive data types in Java are implemented in non object oriented way. This retains the similar performance offered by languages like C and C++. This is the only reason why Java is not a pure Object Oriented Language.
Java language has 8 different primitive data types.
- byte
- short
- int
- long
- float
- double
- char
- boolean
1. Byte
Byte is the smallest data type in Java language. Byte occupies 8 bits in memory. Byte data type is used in applications involving Input Output buffers, streams and raw data. Binary data is also processed using byte data type in many software. Using Byte for numeric applications is not recommended. You can use short, int and long for number type calculations.
Number Range of Byte Data Type
Byte data type can hold numbers between -128 and + 127.
Size of byte data type
Byte occupies only 8 bits or 1 byte in memory.
Example:
byte buf = 124;
2. Short
Short is a numeric data type. A Short data type is half of int data type.
Number Range of short Data Type
Short data type can hold numbers between -32768 and +32767.
Size of short data type
Short data type occupies 2 bytes or 16 bits of memory.
Example:
short num = 1245;
3. Int
Int is a numeric data type. Byte and Short numbers are converted into Int type in expressions for evaluation.
Number Range of int Data Type
Int data type can hold numbers between -2147483648 and 2147483647.
Size of int data type
Int data type occupies 4 bytes or 32 bits of memory.
Example:
int num = 123456;
4. Long
Long is a numeric data type. Byte, Short and Int numbers are converted into Long type in expressions for evaluation if atleast one number is of long type.
Number Range of long Data Type
Long data type can hold numbers between -9223372036854775808 and 9223372036854775807.
Size of long data type
Long data type occupies 8 bytes or 64 bits of memory.
Example:
long num = 123456L or 123456l;
5. Float
Float data type is used to represent real numbers with a fractional part. Java implements IEEE-754 standard to implement floating point numbers. Float is called Single Precision data type. Floating point data is for storing real numbers. On 32 bit machines, float data type is efficient for manipulating real numbers.
Number Range of float Data Type
Float data type can hold numbers between 1.4e-045 to 3.4e+038.
Size of float data type
Float data type occupies 4 bytes or 32 bits of memory.
Example:
float sal = 123456.235f or 123456.235F;
6. Double
Double data type is used to represent real numbers with a fractional part. Double is called Double Precision data type. Double stores the same data as that of a float. The only difference is that double data type stores more digits of precision after the decimal point. Double can store very big real numbers. On 64 bit machines, double data type is efficient for manipulating real numbers.
Number Range of double Data Type
Double data type can hold numbers between 1.4e-045 to 3.4e+038.
Size of double data type
Double data type occupies 8 bytes or 64 bits of memory.
Example:
double sal = 123456.235;
7. Char
Character type data is represented using char data type. While C language uses ASCII to represent just 128 characters, Java language uses UNICODE to represent all international 65536 letters of all languages all over the world. So a char type in Java is bigger with 2 Bytes of storage.
Number Range of char Data Type
Char data type can hold numbers between 0 to 65535.
Size of char data type
Char data type occupies 2 bytes or 16 bits of memory.
Example:
char code = 'A'; char type = 90;//number is converted to character
8. Boolean
Boolean data type represents only two values namely true and false. All relational operations or expressions result in one of these two values. C considers number Zero as false and Non-Zero number a true. But Java does not work in that way.
Values for Boolean Data Type
true, false.
Size of boolean data type
Boolean data type is a primitive data type implemented by Java. Size may be 1 bit.
Example:
boolean exp = true; //works boolean kk = 1; //Error. Can not assign number if(exp) { //works } if(kk) //kk is not boolean type. Error { //.... }