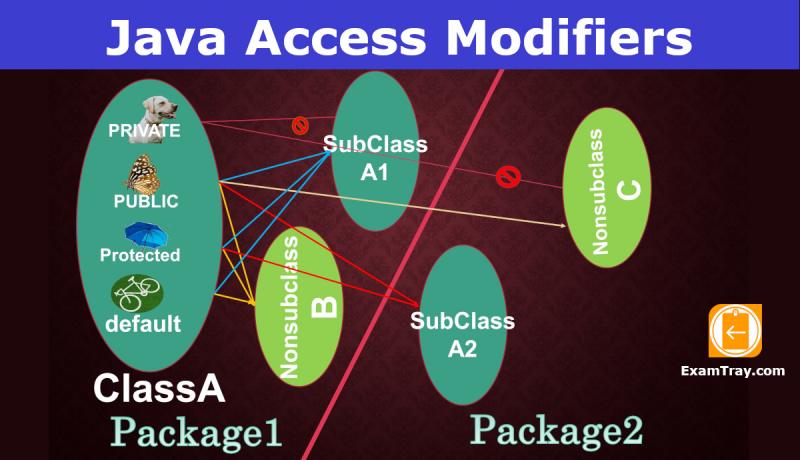
Java access modifiers allow programmers to control and safeguard data like variables and methods of a class. Selective allowing and hiding of data is possible through these Java access modifiers like public, private and protected. The "default" access has no keyword. These modifiers can be combined with packages to get even more encapsulation control. Let us know more in this Last Minute Java Tutorial.
Subclassing in Java is nothing but inheriting a given class for the reuse of readymade code.
Java Access Modifiers Explained
Java access modifiers like public, private, protected and default (keyword not used) work differently in the four possible scenarios. We should assume, we are in one of the below scenarios accessing a particular class.
- Same package direct access
- Same package subclassing
- Different package direct access
- Different package subclassing
1. Private Access Modifier
All private variables and methods can only be used by the same class members. Private members can not be accessed from outside of the class. Private members are most secure.
2. Public Access Modifier
All public variables and methods of a class can be accessed both from the same package and other package methods either directly or after subclassing the given class. Public members are less secure.
3. Protected Access Modifier
All protected variables and methods of a class can be accessed directly or by subclassing the given class of package. To access the protected members of a class belonging to an outside package, you need to subclass the original class you wish to access. In simple words, subclass a class, access its members from anywhere.
4. Default Access Modifier
All default variables and methods without any access-modifier keyword like public, private or protected are accessed with default-access. The default members can be accessed within the same package either by direct or by subclassing. The default members can not be accessed by a class of an outside package even by subclassing or inheritance.
Example Program
Let us create two packages package1 and package2. Under package1, create three classes ClassA, SubclassA and ClassC. Under package2, create two classes SubclassA2 and ClassB. We print member variables in the constructor of each class. Create separate folders for the packages and execute the program from a third package package3 using AccessModifiersExample.java program. It contains a Main() method.
package1.ClassA.java
ClassA contains four String variables with Java access modifiers default, public, private and protected. We try to access these variables from other classes. Only those variables which do not cause the compiler error are accessed in the constructor of each class.
package package1; public class ClassA { public String namePub ="PUBLIC"; private String namePriv = "PRIVATE"; protected String nameProt = "PROTECTED"; String nameDef = "DEFAULT"; public void show() { System.out.println("ClassA = " + nameDef + ", " + namePriv + ", " + nameProt + ", " + namePub); } }
package1.ClassC.java
package package1; public class ClassC { public ClassC() { ClassA a= new ClassA(); System.out.println("ClassC = SamePackage-NonSubclass = " + a.nameDef + ", " + a.nameProt + ", " + a.namePub); } }
package1.SubclassA.java
package package1; public class SubclassA extends ClassA { public SubclassA() { System.out.println("SubclassA - SamePackage-Subclass = " + nameDef + ", " + nameProt + ", " + namePub); } }
Package2.ClassB.java
package package2; import package1.ClassA; public class ClassB { public ClassB() { ClassA a = new ClassA(); System.out.println("ClassB - DiffPackage-Nonsubclass = " + a.namePub); } }
Package2.SubclassA2.java
package package2; import package1.ClassA; public class SubclassA2 extends ClassA { public SubclassA2() { System.out.println("SubclassA2 - DiffPackage-Subclass = " + nameProt + ", " + namePub); } }
AccessModifiersExample.java
package package3; import package1.*; import package2.*; public class AccessModifiersExample { public static void main(String[] args) { ClassA a = new ClassA(); a.show(); SubclassA a1 = new SubclassA(); SubclassA2 a2 = new SubclassA2(); ClassC c = new ClassC(); ClassB b = new ClassB(); } } //OUTPUT ClassA = DEFAULT, PRIVATE, PROTECTED, PUBLIC SubclassA - SamePackage-Subclass = DEFAULT, PROTECTED, PUBLIC SubclassA2 - DiffPackage-Subclass = PROTECTED, PUBLIC ClassC = SamePackage-NonSubclass = DEFAULT, PROTECTED, PUBLIC ClassB - DiffPackage-Nonsubclass = PUBLIC
Refer to the Java Access Modifiers table image to cross-check the output of the above program.
Share this Last Minute Java Access Modifiers tutorial with your friends and colleagues to encourage authors.