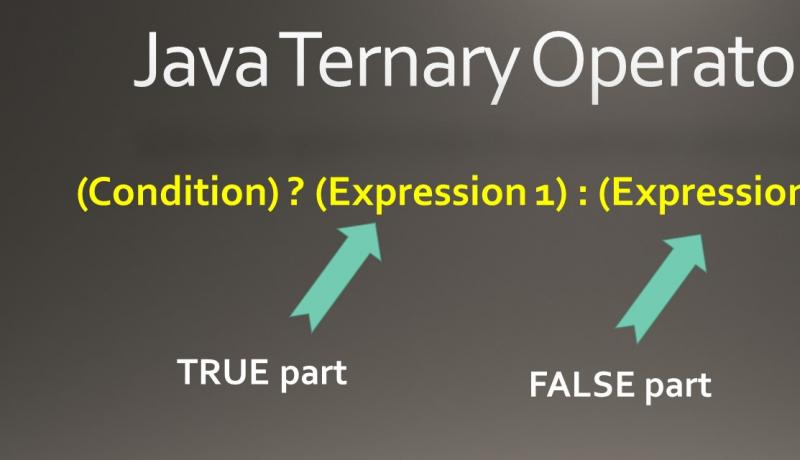
Java language has a special operator called Ternary Operator that works like a Shortcut IF ELSE control statement. It is also called a Conditional Operator.
Java Ternary Operator (?:) or Conditional Operator Explained
Java Ternary Operator (?:) or Question Mark Colon Operator tests a condition and moves the control to two branching points, one for true and one for false.
Syntax:
(condition)? (true part expression): (false part expression)
The condition can be a direct relational operation or a boolean logical expression or a simple true/false. True Part and False Part must return a value. So you can not write a Ternary operator statement that does not return anything. You can not even put Functions returning void.
You can use Java Ternary Operator with any control statement that expects a boolean type value. So, you can use Ternary Operator with IF, ELSE IF, WHILE, DO WHILE, FOR and more.
Java Ternary operator has got the least priority among all other operators like Arithmetic, Relational, Bitwise and Logical. It has more priority than Assignment operators and Lambda operator.
Example Error 1: Assignments and Return Value in Ternary Operator
You can not use Java Ternary Operator alone as a standalone statement. So, do not write a Ternary operator without an assignment operation. Also return value is a must. You can not put void returning method calls inside branching expressions of a Ternary Operator. You get the following errors.
- Syntax error, insert "AssignmentOperator Expression" to complete Expression
- The left-hand side of an assignment must be a variable
- void is an invalid type for the variable
- Type mismatch: cannot convert from void to int
class TernaryOperator1 { public static void main(String args[]) { int a =5; (a<6)?5:6; //Error int b = (a<6)?5:6; //WORKS int c = (a>6)?5:6; //WORKS int d = true?show():show(); //ERROR. No Return Value } static void show() { System.out.println("SHOW METHOD"); } } //ERROR: //Left Hand side of an assignment must be a variable //Solution: Use a variable and assign //Now b holds 5 as a<6 is true //Now c holds 6 as a>6 is false //show method returns void. It is not valid in Java.
Example 2: Ternary Operator with boolean values
You can put an expression resulting in any data type say byte, short, char, int, long, float, double, String and Object as part of TRUE PART or FALSE PART of a Ternary Operator.
class TernaryOperator2 { public static void main(String args[]) { boolean b; b = false?2<4: 5>7; //false?false:false } } //Now b = 5>7 ==> holds false
Example 3: Ternary Operator with null object Checking
An object occupies memory. If it is set to NULL (null), the memory occupied by it will be released by the Garbage Collector. If an object is null, you can not use or reference any of its member variables or methods.
class TernaryOperator3 { public static void main(String args[]) { String a = "Hello Java", b=null; b = (b==null)?a: b; //b =a System.out.println(b); b = (b==null)?a: "2 "+ b; // b = "2 " + b System.out.println(b); } } //Hello Java //2 Hello Java
Example 4: Ternary Operator with String Assignments Inside
You can do assignment operations inside TRUE Part or FALSE Part of a Ternary Operator. Make sure, the return type is compatible with the assigning constants. We have done String assignments in this example.
class TernaryOperator4 { public static void main(String args[]) { String a = "Hello Java"; String b = a!=null? a="HELLO":(a="JAVA"); System.out.println(b); } } //OUTPUT //HELLO
Example 5: Nesting of Java Ternary Operators with Multiple Conditions
You can nest one Ternary operator inside another Ternary operator. You should carefully select the output data type of one Ternary operator sitting inside another ternary operator. Here, the first condition is NULL comparison and the second condition is EQUALS comparison.
class TernaryOperator5 { public static void main(String args[]) { String a = "Java"; String b = a!=null? a.equals("Java")?"YES JAVA":"NO JAVA":"JAVA ISLAND"; //a!=null? (a.equals("Java")?"YES JAVA":"NO JAVA"):"JAVA ISLAND"; String a = null; String b = a!=null? a.equals("Java")?"YES JAVA":"NO JAVA":"JAVA ISLAND"; System.out.println(b); } } //OUTPUT //YES JAVA //JAVA ISLAND
Example 6: Using Ternary Operator with IF ELSE statements
You can use Ternary Operator inside an IF or ELSE IF statements if it is returning a boolean data type constant.
class TernaryOperator6 { public static void main(String args[]) { if(2>0?true:5<10) { System.out.println("Using Ternary Inside IF"); } } } //OUTPUT //Using Ternary Inside IF
This is how a Java Ternary Operator works. Practice these examples on a PC to get more knowledge.
Share this tutorial with your friends and colleagues to encourage authors.