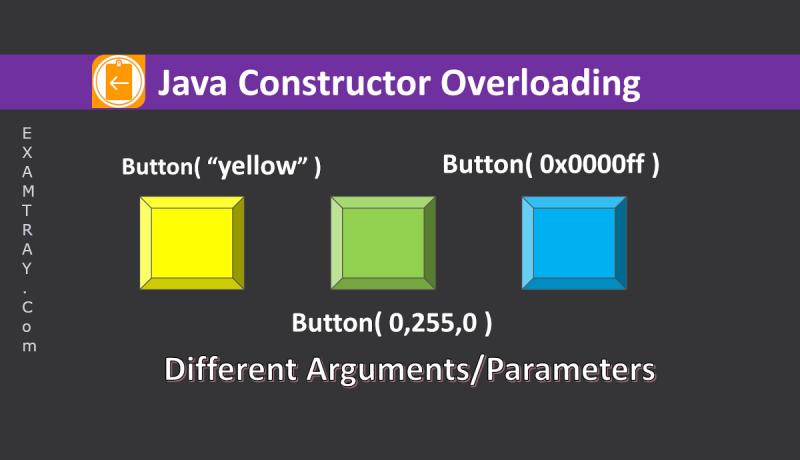
All Java classes contain a Constructor. Even if we do not create a constructor, the compiler creates a default constructor for us. A Constructor's name is the same as the Class name. Let us know more about Java Constructor Overloading and Constructor usage in this Last Minute Java tutorial.
What is a Java Constructor
A Java Constructor is like a Method without Return Type in which initializations happen.
Java Constructors are of two types.
- Default Constructor
- User-Defined Constructor
1. Default Constructor
A Default Constructor in Java is a constructor without any parameters (Zero parameters). If you do not write, Java compiler adds a default constructor before compiling for you. An example is given below.
class Piano { int keys; Piano() { //commented code //we can write some code like keys=20; } }
In the above example code, all new objects of the Piano type created will be having a default value Zero for the property "keys".
2. User-Defined Constructor
A User-Defined constructor in Java is a constructor created by the user with a certain number of Parameters. An example is given below.
class Piano { long keys; Piano(long numKeys) { keys = numKeys; } }
In the above example, we have created a constructor that accepts a number of piano keys as a parameter. Piano's Property "keys" has been initialized with the received argument or parameter. So, developers will have more comfort using this user-defined constructor.
Note: Constructors support implicit typecasting of parameters if those are of convertible or compatible data types. Here, upcasting of parameters happen automatically. Java Runtime automatically searches for a matching Constructor and invokes it. So, calling the above with Piano(int) parameter automatically calls the Piano(long) constructor if Piano(int) does not exist. Check the example below with integers and double values.
public Class Forest { double animalCount; Forest(double count) //accepts int, long, float and double values { animalCount = count; } public static void main(String[] args) { int animals = 10; Forest forest = new Forest(10); //passing int value System.out.println("Animals = " + forest.animalCount); } }
It is time to introduce Overloading of Java Constructors now.
Java Constructor Overloading explained
Java Constructor Overloading is nothing but writing more than one Constructor in a Class with distinct parameter list. That means Java allows you to use the same Constructor name any number of times as long as you write unique constructors. To maintain uniqueness, you can simply change the Data Type or Class Type while accepting parameters.
Java Constructor Signature is nothing but a combination of Constructor's name and Parameter List. To overload a constructor, you should maintain a unique Constructor Signature.
Below is a good example that explains Constructor Overloading.
public class Piano { int keys; String name; float price; Piano(int keys2) { keys = keys2; } Piano(float price2) { price = price2; } Piano(String name2) { name = name; } Piano(String name2, int keys2, float price2) { //some code } Piano(int keys2, float price2, String name2) { //some code } Piano(float price2, int keys2, String name2) { //some code } public static void main(String[] args) { Piano p1 = new Piano("ONE"); Piano p2 = new Piano(32); Piano p3 = new Piano(50.5f); Piano p4 = new Piano("TWO", 24, 45.5f); Piano p5 = new Piano(40, 25.5f, "THREE"); Piano p6 = new Piano(94.5f, 50, "FOUR"); } }
If you do not Overload a Constructor properly, you will get an error. Let us check another example that shows wrong way of defining constructors that fail to overload.
class Piano2 { int keys; int price; Piano2() { System.out.println("NEW Piano Created."); } Piano2(int keysNum) //Piano2(int) { keys = keysNum; } Piano2(int price2) //Piano2(int) //Error: Duplicate Constructor Signature { price = price2; } }
You should do Constructor Overloading in such a way that the Java Runtime can identify correctly which constructor to choose depending on the data type of parameters in the list. Otherwise, the compiler throws an error in the format "Duplicate method CONSTRUCTORNAME(datatype)".
This is about Java Constructor Overloading and its usage. We shall discuss Method Overloading in coming chapters.
It is time to Share this Last Minute Java Tutorial with your Friends and Colleagues to encourage authors.