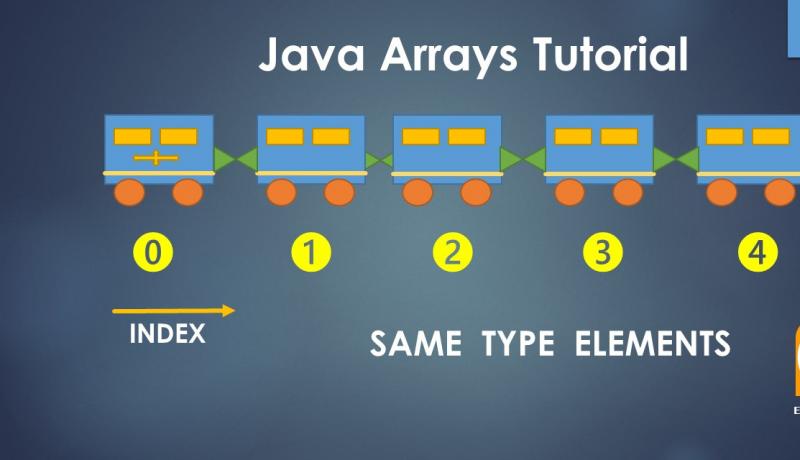
An array is a collection of elements of same data type Primitive or Object type. Each element has a position number called an Index. The size of an array is simply the number of elements of that array. Let us learn Java arrays and multidimensional arrays in detail.
Note: Java arrays are implemented as Objects. So, a Java array variable has some predefined methods and fields. One such useful read-only FIELD is the length, which holds array size.
Java One Dimensional Arrays
An array can be thought of like a Train with a number of Coaches and each coach has a number. If we know the coach number, we can directly go to that coach easily. Coach number is comparable to an array element INDEX. A Single train represents one dimension 1D or 1D array.
Multiple trains represent Multidimensional arrays where each train also has an index starting with Zero along with a separate index for train coaches or array elements. Index of an array always starts with ZERO and ends with SIZE-1.
0) Array Declaration
Array declaration is nothing but declaring an array variable with a Type. Type can be primitive or non-primitive. Array declaration does not allocate memory for an array variable. There is no need to mention the Size of an array during declaration. Difference between a normal variable and an array variable during declaration or accessing is the addition of Square Brackets and a Subscript indicating the Size, Index or element Position.
Syntax:
Type[] variable; (or) Type variable[]; eg. int ary[]; String[] strAry; int[] ary1, ary2, ary3; int ary1[], ary2[], ary3[];
1) Array Inline Initialization or Shorthand Initialization
Array Inline Initialization does not ask for mentioning its size. At the time of the Declaration itself, you can initialize all array elements using a Shorthand method with two braces and a list of values separated by Commas. You should not add size within square brackets next to the array variable. It is a static way of initializing as the array size cannot be specified at Runtime afterwards.
The problem with this approach is that you can not declare an array without size if you are not initializing it on the same line or statement and later use this Shorthand Initialization approach. You get an error like "Array constants can only be used in initializers".
Syntax:
TYPE variable[] = {value1, value2, ..., valueN}; eg. int marks[] = {45, 56, 76, 55};
Example: Array initialization with integers and String
int nums[] = {1,24,54,35,87}; for(int i=0; i<5; i++) { System.out.print(i + ","); } String names[] = {"CRICKET", "HOCKEY", "SOCCER"}; for(int i=0; i<names.length; i++) { System.out.println(names[i]); }
2) Lazy Array Initialization / Declare and Initialize Array Later Approach
Lazy array initialization is also called Dynamic Array Initialization. You should not specify the size during the declaration. You can specify the size of an array at Runtime. On the first line in the below example, we have not specified the size of an array.
Syntax:
TYPE variable[]; variable = new TYPE[ SIZE ]; eg. int ary1[]; ary1 = new int[5]; ary1[0]=45; ary1[4]=55; String str[]; str = new String[10]; str[2] = "CABBAGE"; str[3] = "TOMATO";
In general, variables that are declared inside methods are called Local variables. You can declare a local variable of any data type or Object type, but you can not use it without initialization. If you try to use an uninitialized variable, you get an error saying "The local variable may not have been initialized". Variables that are created using the keyword "new" are an exception to this rule.
Example:
public void show() { int p; //NO Error System.out.println(p); //Error for using it }
Rules for Lazy array Initialization:
- Array size must be specified along with new keyword.
- Array Type must be specified
- A valid identifier for array variable should be given
- Keyword "new" is used to specify the size of the array
To create a new Object or an array in Java, we use a "new" keyword. This "new" keyword allocates memory for the array or an Object. Using the keyword new causes the objects and array elements to get some default values. For primitive data types, default values are dependent on the data type. For Objects, the default value is null. Integers are initialized with a default value of ZERO. Boolean values are initialized with "false" by default.
Data Type | Default Value |
---|---|
byte | 0 |
short | 0 |
char | null or '\0' |
int | 0 |
long | 0 |
float | 0.0 |
double | 0.0 |
Object | null |
String |
null ( String is an Object) |
Java Multidimensional Arrays
A Multidimensional array in Java is simply an Array of Arrays. It can be comparable to a Matrix with a Row number and a Column number. The row index or number starts at 0. The column index or number starts at 0. Depth or Dimension of an array is represented by the letter 'D'. So, 3D arrays represent a 3-dimensional array.
An N-dimensional array consists of an array of (N-1)D arrays. For example, a 3D array consists of an array of 2D arrays.
Array Declaration
A multidimensional array can be declared just like declaring a Single Dimensional array as shown above. You should specify the leftmost dimension or subscript.
Syntax:
int ary[4][][]; //Left most subscript is compulsory int myary[2][];
Array Initialization
Multidimensional arrays in Java can be initialized in two ways.
- Inline Initialization
- Lazy Initialization
Inline Array Initialization
To declare and initialize a Multidimensional array, there is no need to mention the size or any dimension. All subscripts can be left empty within Square Brackets.
Example:
int twoAry[][] = {{1,2}, {5,4,3}}; for(int i=0; i<twoAry.length; i++) { for(int j=0; j<twoAry[i].length; j++) { System.out.print(twoAry[i][j] + " "); } System.out.println(""); } //OUTPUT //1 2 //5 4 3
Lazy Array Initialization
To create a multidimensional array with a new keyword, you need to specify the leftmost dimension or subscript.
Example
int twoAry[][]; twoAry = new int[2][]; //or //twoAry = new int[2][3]; twoAry[0] = new int[5]; twoAry[1] = new int[4]; int c=0; for(int i=0; i<twoAry.length; i++) { System.out.println("Row-" + (i) + "-size=" + twoAry[i].length); } //OUTPUT Row-0-size=5 Row-1-size=4
This is how Java Arrays work.
It is time to Share this tutorial with your friend and colleagues.