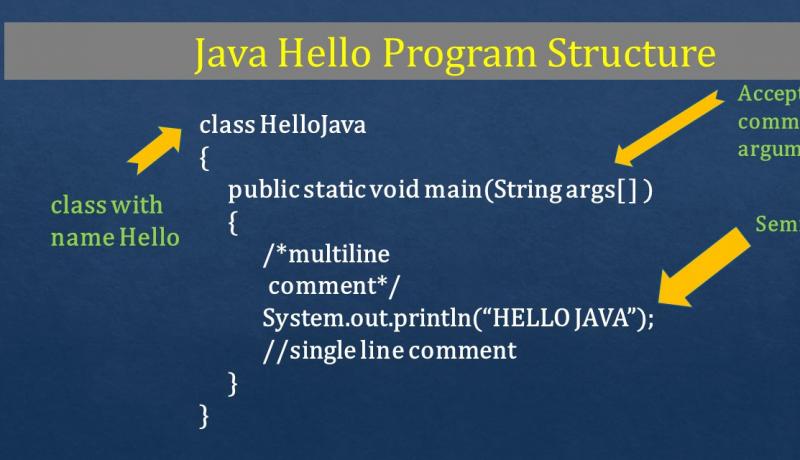
All java programs contain some class definition. All functions or methods are kept inside the class. You need to know how to place a main method with public static void inside Class definition. Let us know the basic structure of a Java Program for better understanding next Last Minute Java Tutorials in the queue.
Java Program Structure Explained
Here is the sample Hello Java program. Check the tutorial on installing and running Java.
We shall see each line and analyse.
class HelloWorld { /* Class variables (static) can come here */ // instance variables (non static) come here public static void main(String args[]) { int a=10; float b=12.345; System.out.println("Hello World Java"); } }
Observations are below.
- Keywords appearing in the above example are class, public, static, void, int and float.
- A Class is like an enclosure or a bigger block definition with inner members. A Class can contain variables and methods. It has opening and closing braces { }.
- HelloWorld is the name or identifier given to the class.
- main() is a function. In Java, you should call a function as Method. It has opening and closing braces { }.
- A multiline comment with /* and */ can contain any dummy info which is not processed.
- A single line comment with // allows us to write a single line comment.
- Every statement in Java must end with a Semicolon (;).
- Static variables and methods inside a class are called Static members or Class Members. Members can be Variables or Methods.
- Non static variables and methods inside a class are called Instance Members ( Instance Variables, Instance Methods).
- public before main method is an access modifier that exposes a method to be called from outside.
- static before main method makes it to be called even without instantiating an object.
- void before main method tells that the method does not return anything.
- Main method is taking an argument or paramter as a String Array - args[]. It allows the main method to take arguments from Command Line when running the program.
- Variables a and b are local variables that die after main method finishes execution.
- System.out has methods to read and print. Here we are using println which prints a new line after printing text.
- A Class can, in turn, contain variables of another class type similar to a Structure Type Variable in C language.
Thank you for reading this tutorial. It is time to Share it with your friends and colleagues.
Prev Chapter Link