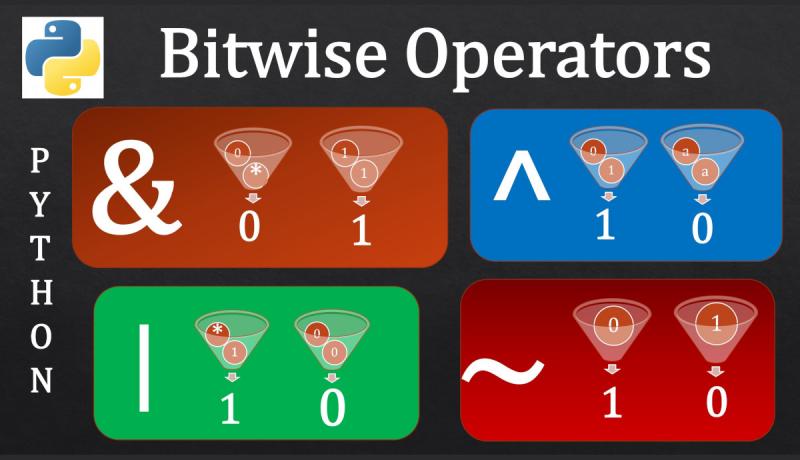
Python Bitwise Operators work on integer type operands at bit-level. A number is converted to 1's and 0's before a bitwise operator is applied. Let us learn more in this Last Minute Bitwise Operators and Priority tutorial using good examples.
Bitwise operators are symbols but not keywords like in logical operators and boolean operators.
Tip: bin() method converts a number in the decimal system to binary.
Python Bitwise Operators
There are six bitwise operators in Python.
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise Exclusive OR (^)
- Bitwise Complement (~)
- Bitwise Left Shift (<<)
- Bitwise Right Shift (>>)
1. Bitwise AND (&)
Bitwise AND is represented by the Ampersand (&) operator.
If both the inputs are 1s, Bitwise AND gives the output as 1. Otherwise, the output will be 0.
Bitwise AND Truth Table:
Input1 | Input2 | Output |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Example:
a=4; #0100 b=14;#1110 print(bin(a)); print(bin(b)); print(a&b) #output----- #0b100 #0b1110 #4 (0100)
2. Bitwise OR (|)
Bitwise OR is represented by the Pipe (|) operator.
If at least one input is 1, the output of a Bitwise OR is 1. Only if both inputs are 0s, the output is 0.
Bitwise OR Truth Table:
Input1 | Input2 | Output |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Example:
a=5; #0101 b=12;#1100 print(bin(a)); print(bin(b)); print(a|b) print(bin(a|b)); #output #0b101 #0b1100 #13 #0b1101
3. Bitwise Exclusive OR (^)
Bitwise XOR (Exclusive OR) is represented by the Carat or Cap operator.
If both the inputs are different, the output of a Bitwise XOR is 1. Otherwise, the output is 0.
Bitwise XOR Truth Table:
Input1 | Input2 | Output |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Example:
a=6; #0110 b=10;#1010 print(bin(a)); print(bin(b)); print(a^b) print(bin(a^b)); #output #0b110 #0b1010 #12 #0b1100
4. Bitwise Complement (~)
Bitwise Complement is represented by the Tilde (~) operator.
Bitwise complement operator toggles the input from 0 to 1 and 1 to 0.
Bitwise Complement Truth Table:
Input | Output |
0 | 1 |
1 | 0 |
Example: In the below example, after toggling all bits of 14 (00001110), you get 11110001 in binary. Since the leftmost bit is 1, it is a negative number. So, after ignoring the leftmost 1, you should toggle all bits (000 1110) and add 1 (000 1110 + 1 = 0001111) to the result to get the number in decimal notation. Prefix a minus (-) symbol before the result. It becomes -15.
In other words, to convert a negative binary number to decimal, you should do One's complement and add 1 to the result. This is also called 2's complement.
x=14; #00001110 print(x, '=', bin(x)) print(~x) #1111 0001 print(bin(~x)) #output #14 = 0b1110 #-15 #-0b1111 = +11110001
5. Bitwise Left Shift (<<)
Bitwise Left Shift is represented by the two less-than symbols (<<).
Left-Shift operator shifts the bits of a number to the left side a specified number of times. Overflow bits on the left side are ignored.
Example:
x=3; #0000 0011 print(x, '=', bin(x)) print(x, '<<2=', x<<2) print(x<<2, '=', bin(x<<2)) #output #3 = 0b11 #3 <<2= 12 #12 = 0b1100
6. Bitwise Right Shift (>>)
Bitwise Right Shift is represented by the two greater-than symbols (>>).
Right-Shift operator shifts the bits of a number to the right side a specified number of times. Overflow bits on the right side are ignored. The sign of the number is retained even after truncating the left most bits of the input number.
Example:
x=10; #0000 1010 print(x, '=', bin(x)) print(x, '>>2=', x>>2) print(x>>2, '=', bin(x>>2)) #output #10 = 0b1010 #10 >>2= 2 #2 = 0b10
Python Bitwise Operator Priority or Precedence
Python Bitwise Complement (~) operator has got the highest priority among all Bitwise operators. Bitwise OR (|) operator has the lowest priority among all bitwise operators.
Bitwise Operator Priority Table:
Bitwise Operator | Priority |
Bitwise Complement ~ | 1 |
Bitwise Left Shift <<, Bitwise Right Shift >> | 2 |
Bitwise AND & | 3 |
Bitwise XOR ^ | 4 |
Bitwise OR | | 5 |
Python Bitwise Operators have lesser priority than Arithmetic Operators. Only TILDE (~) operator has higher priority than Arithmetic operators. Bitwise operators have higher priority than Relational Operators, Assignment Operators, Membership Operators, Logical Operators and Boolean Operators.
In the coming chapters, you will learn Operator Priority of all Python operators.
Share this Last Minute Python tutorial on Bitwise Operators and their Priority with your friends and colleagues to encourage authors.