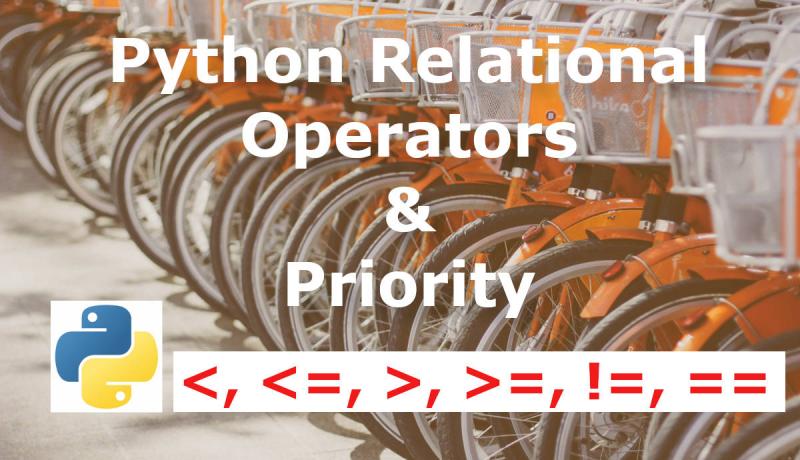
Python language supports 6 relational operators or comparison operators. These operators allow you to compare two variables or constants. Let us learn more about these relational operators in this Last-minute Python tutorial with examples.
You can also learn Arithmetic operators in Python to create complex expressions.
Python Relational Operators or Comparison Operators
The output of a comparison operation (with operators) is always boolean type with the value either True or False. So, these relational operators are used inside conditional statements like IF, ELIF and WHILE.
Relational operators list is given below.
SNO | Operator Symbol | Operator Name |
1 | > | Greater Than |
2 | >= | Greater Than or Equal to |
3 | < | Less Than |
4 | <= | Less Than or Equal to |
5 | == | Equal to |
6 | != | Not Equal to |
These operators have the same functionality in other languages like C and Java.
Examples:
#using Less Than operator =20; k=30; if(p<k): print("YES") else: print("NO") #output YES #using Less than or Equal to operator c=1 while(c <= 5): print(c, end=' ') c+=1 #output 1 2 3 4 5 #using Not equal to operator fr_1 = 'apple' fr_2 = 'orange' if(fr_1 != fr_2): print("\nNot Equal") #output Not Equal str2= ''; if str2 != None: print('Empty string is not None') #output Empty string is not None
In the above example, two strings (fr_1 and fr_2) are compared. Python compares string variables using the actual values instead of memory locations. So, the strings with different values are considered different in Python. Also, an empty string is not treated as the None data-type.
Also, Python compares Sequence data types like list, tuple, set and dictionary using the internal elements. So, if two lists, for example, are having the same elements, then those lists are the same. Python comparison-operators know this and give the output accordingly.
Comparing two or more arrays works in the same way as comparing the sequence objects. If the elements are the same in two arrays, those arrays are considered identical in Python.
Examples:
#comparing lists list2 = [3, 5, 8, 10] list3 = [3, 5, 8, 10] list4 = list2 print(list2 == list3) #output #True print(list2 == list4) #output #True #comparing dictionary items dict2 = {1:'tiger', 2:'deer'} dict3 = {1:'tiger', 2:'deer'} dict4 = dict2 print(dict2==dict3) #output #True print(dict2==dict4) #output #True print(dict3==dict4) #output #True #comparing Arrays from array import * ary2 = array('i', [4,5,6,7]); ary3 = array('i', [4,5,6,7]); ary4 = ary3 print('Array ==', ary2 == ary3) #output #Array == True print(ary2 == ary4) #output #True
Python supports chaining of relational operators. This feature allows Python to combine multiple conditions into a single condition. This feature allows programmers to easily understand the logic written by others.
Example without chaining: We have used a Logical operator "and" in this example to combine two conditions.
p2=11; p3=20; p=15 if p>p2 and p<=p3: print("Gorilla")
Example with chaining:
k=21; n=30; c=24; if k<c<=n: print("Plane")
Notice that the operator chaining is very easy to use and easy to understand.
Python Relational Operator Priority
All the 6 Python Relational or Comparison operators have the same priority. So the order of evaluation of an expression is from the left to right if more than one relational operator is used.
Arithmetic and Bitwise operators have higher priority than Relational operators. Assignment and Logical operators have lesser priority than Relational operators.
Comparison Operator | Priority |
<, <=, >, >=, !=, == | 1 (Same) |
Example:
marks=98 if marks>95 and marks<100 print("Good Score")
In the above expression, the first condition with 95 is evaluated first. Then the condition with 100 is evaluated. Both conditions involve relational operators having equal priority.
Share this Last Minute Python tutorial on Relational Operators and their Priority with your friends and colleagues to encourage authors.