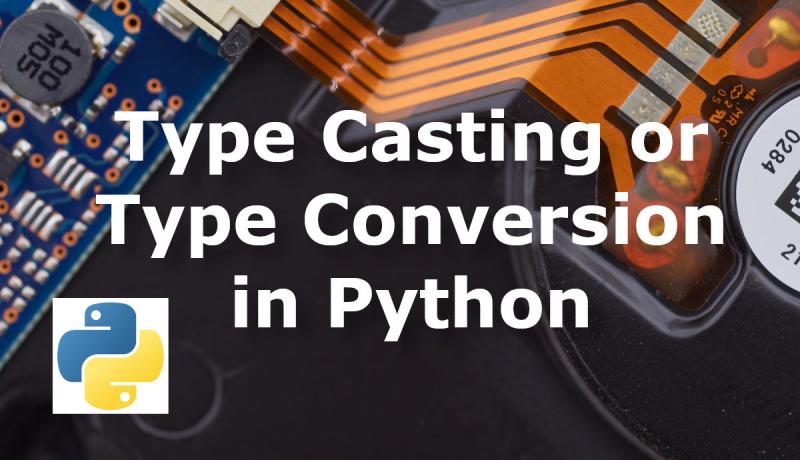
Python programming language has built-in support for various data types. Some times, the programmers need Type Conversion or Type Casting support in Python. Let us know more in this Last Minute Python tutorial with examples.
Type Conversion is also called Parsing if it involves Strings.
You can check the compiling and running a python program article before experimenting with these examples below.
Python Type Casting or Type Conversion
Converting one type of data into another type is called Type Casting or Conversion. Most of the conversions in Python are explicit in nature using some functions or methods.
Below is the list of allowed type conversions with numeric data.
- int to float conversion
- int to complex conversion
- float to int conversion
- float to complex conversion
Float to int conversion is always lossy.
Below is the list of invalid type casting or conversions with numeric data.
- complex to int conversion
- complex to float conversion
Below is the list of possible type conversions or parsings between string and numeric types.
- str to int
- str to float
- str to complex
- int to str
- float to str
- float to complex
Below is the list of possible conversion between bool and other data types.
- int to bool
- float to bool
- complex to bool
- string to bool
- bool to int
- bool to float
- bool to complex
Below is the list of possible or allowed type conversions among Sequences.
- range to list
- range to tuple
- range to set
- list to tuple
- list to set
- tuple to list
- tuple to set
- set to list
- set to tuple
- set to str
- tuple to str
- range to str
- list to str
Example 1:
a = 10 b = float(a) #int to float conversion print(b) #output 10.0 c = complex(a) #int to complex conversion print(c) #output (10+0j) d = 15.5 #float e = int(d) #float to int conversion print(e) #output 15 f = complex(d) #float to complex print(f) #output (15.5+0j)
Example 2:
str_a = '15' int_a = int(str_a) #string to int conversion print(int_a) #output 15 print(int_a + 1) #output 16 str_b = '25.89' float_b = float(str_b) #string to float conversion print(float_b) #output 25.89 int_b = int(float_b) #float to int conversion print(int_b) #output 25 str_c = '25+3J' con_c= complex(str_c) #string to complex conversion print(con_c) #output (25+3j)
TIP: It is a good practice to parse numbers in a string (str) format to float first and int conversion next. If you directly cast a float value inside the string to int, you will get an error like "ValueError: invalid literal for int() with base 10".
You can convert a bool type value to an int without using the int() method. This conversion is implicit or automatic.
Example 3:
boolean = True a = 10 + boolean #boolean to int conversion print(a) #output 11 bool1 = bool(3) #int to bool conversion print(bool1) #output True bool2 = bool(5.5) #float to bool conversion print(bool2) #output True bool3 = bool('abc') #string to bool conversion print(bool3) #output True bool4 = bool(5+6j) #complex to bool conversion print(bool4) #output True
Example 4:
list1 = [5, 7, 9, 11, 15] tuple1 = tuple(list1) #list to tuple print(tuple1) #output (5, 7, 9, 11, 15) set1 = set(list1) #list to set print(set1) #output {5, 7, 9, 11, 15} list2 = list(set1) #set to list print(list2) #output [5, 7, 9, 11, 15] list3 = list(tuple1) #tuple to list set5 = set(tuple1) #tuple to set tuple5 = tuple(set5) #set to tuple range1 = range(0,6) list4 = list(range1) #range to list print(list4) #output [0, 1, 2, 3, 4, 5] set4 = set(range1) #range to set print(set4) #output {0, 1, 2, 3, 4, 5} tuple4 = tuple(range1) #range to tuple print(tuple4) #output (0, 1, 2, 3, 4, 5)
You can try some other combinations of these data types for conversions or type castings.
Share this Last Minute Python tutorial on Type Casting or Type Conversion with your friends and colleagues to encourage authors.